Printing Fact Variables using debug module
Now let’s learn how to fetch any value from a json output. Later in the blog we will be doing that. A json is a form of dictionary in python. Dictionary can have a key value pair, dictionary can have a dictionary and dictionary can have a list as well. In the same order we will fetch some value using the setup module. These keys are called the facts variables. We can disable this option in our playbook as well.
We have created a sample playbook named print_fact.yml and here, we are printing the value of the OS family. We have seen that there are lots of built-in fact variables that we get by default when setup module executes.
Here, we are using the debug module which will help us to print fact variable. Ansible uses Jinga2 templating and thus we have enclosed ansible_os_family in {{}}.
Let’s write a playbook in the same order.
---
- hosts: all
#gather_facts: False
tasks:
- name: Print some facts variables
debug:
msg: "OS Family is {{ansible_os_family}}"
- name: Print memory details
debug:
var: ansible_memory_mb.real
- name: Print free RAM size
debug:
msg: "Free RAM size is {{ansible_memory_mb.real.free}} MB"
- name: Python info
debug:
var: ansible_python.version_info[0]
Note: Debug here is a module name and var/msg are options available in it. Also, to access a dictionary we will use a .(dot) and to access a list index we will access an index number.
ansible-playbook playbook-exercise/print_fact.yml
Here is the output of the playbook:
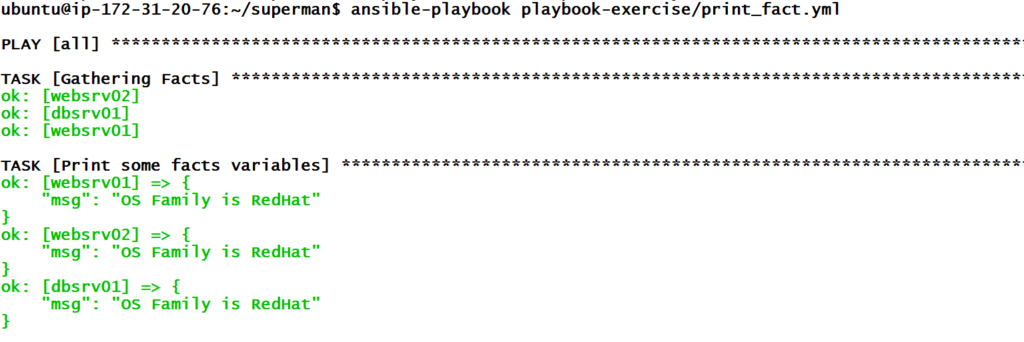
Levels of verbosity
Sometimes, even looking at the output doesn’t make us understand what exactly the issue is. So, there are 4 levels of verbosity and if we want to know more in the output, then we can add those levels during the execution of the playbook.
Level1
ansible-playbook -v web_db.yml
Level2
ansible-playbook -vv web_db.yml
Level3ansible-playbook -vvv web_db.yml
Level4ansible-playbook -vvvv web_db.yml
You can run all these commands to see the output. The number of occurrence of -v denotes the levels of verbosity.
Conditions in Playbook
In our previous article we got an error when we tried to install package httpd on web server 03 and we mentioned that we will resolve the error in the next article. So, let’s see what happened which caused installation failure on web server 03.
Httpd package could not be installed on web server 03 as web server 03 has an Ubuntu OS and this OS doesn’t have httpd instead it has apache2 to handle HTTP requests and yum is also not available there, so we will use apt to install the package in Ubuntu.
So, to do this, we can apply conditions in the playbook. Below is the code for the playbook which depending on the OS family decides which package to install using yum or apt.
---
- hosts: websrvgrp
become: yes
tasks:
- name: Install httpd service
yum:
name: httpd
state: present
when: ansible_os_family == "RedHat"
- name: Install apache2 service
apt:
name: apache2
state: present
when: ansible_os_family == "Debian"
- name: Start and Enable httpd service
service:
name: httpd
state: started
enabled: yes
when: ansible_os_family == "RedHat"
- name: Start and Enable apache2 service
service:
name: apache2
state: started
enabled: yes
when: ansible_os_family == "Debian"
ansible-playbook playbook-exercise/conditions.yml
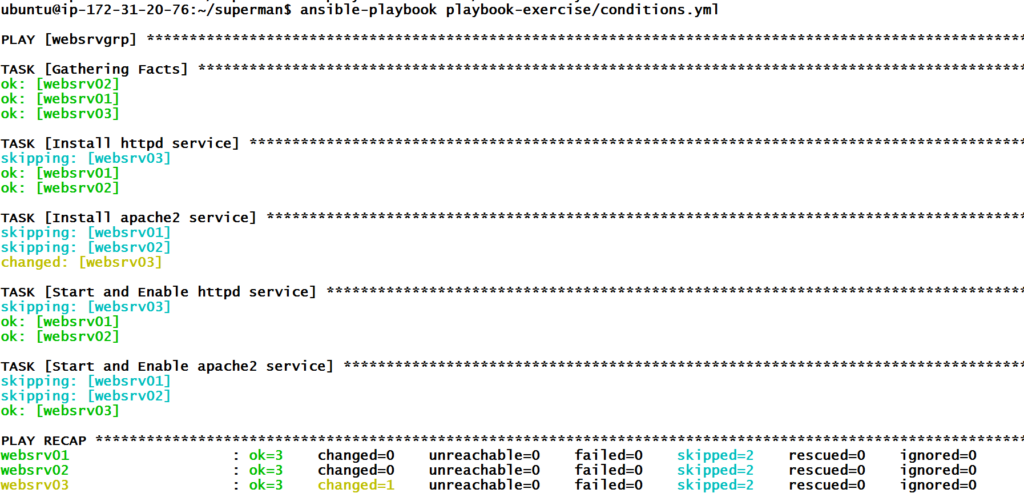
So, we can see now that issue has been resolved and for TASK [Install Httpd service] ansible is skipping web server 03 and for TASK [Install apache2 service] ansible is skipping web server 01 and 02.
That’s how we can do lots of interesting things with conditions in the playbook. For example, depending on free ram size with fact variables we can skip or perform some tasks, etc.
As we have learned conditions in the playbook, now let’s deploy some sample files on all web servers 01,02 and 03 and this time, I hope you will understand what is being written in the playbook.
Deploying sample file on different web servers using a playbook
So, to deploy files on web servers we are going to use copy and service modules of ansible.
- Copy module will copy the files from source to destination.
- The service module will help us in starting and enabling the service.
But before that let’s create a sample index.html file in the files directory with the below commands.
mkdir files
vi files/index.html
Let’s write a web_condition playbook to do the task for us.
---
- hosts: websrvgrp
become: yes
tasks:
- name: Install apache http SVC
yum:
name: httpd
state: present
when: ansible_os_family == "RedHat"
- name: Install Apache apache2 on Ubuntu
apt:
name: apache2
state: present
when: ansible_os_family == "Debian"
- name: Start and enable Apache http SVC
service:
name: httpd
state: started
enabled: yes
when: ansible_os_family == "RedHat"
- name: Start and enable apache2 SVC on ubuntu
service:
name: apache2
state: started
enabled: yes
when: ansible_os_family == "Debian"
- name: Deploy index file
copy:
src: files/index.html
dest: /var/www/html/index.html
owner: root
group: root
mode: '0644'
backup: yes
- name: Restart Apache httpd on Centos
service:
name: httpd
state: restarted
when: ansible_os_family == "RedHat"
- name: Restart Apache apache2 on Debian
service:
name: apache2
state: restarted
when: ansible_os_family == "Debian"
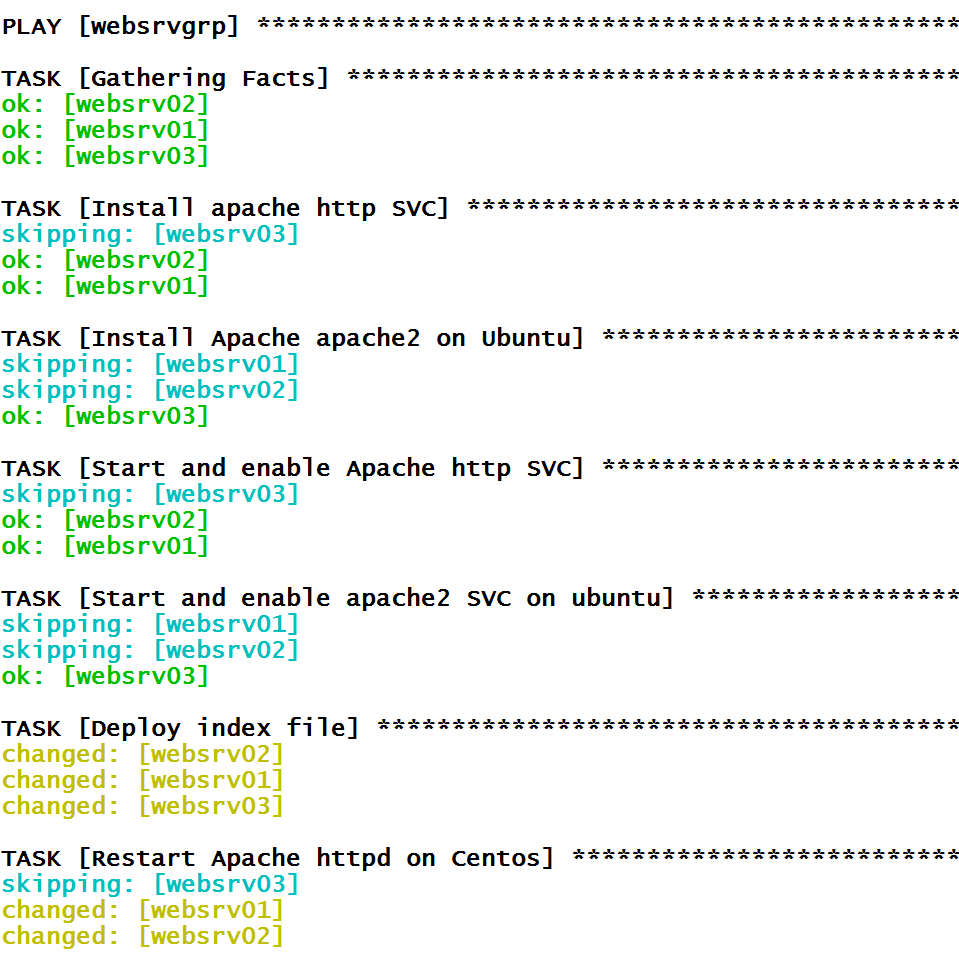
We can see that all of our tasks have been completed successfully. Now, let’s open one of the web servers using the public IP in AWS and paste it on the browser and see if it works.
On Browser
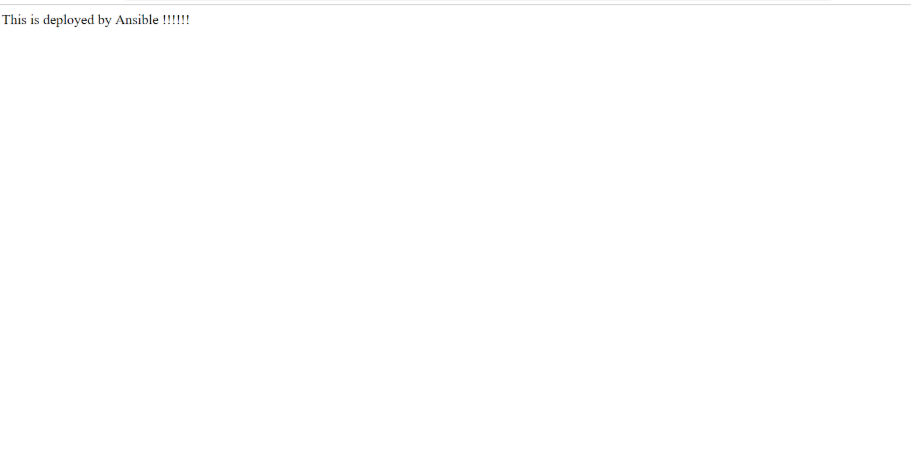