Before jumping into reading properties file, let’s first create a project as below:
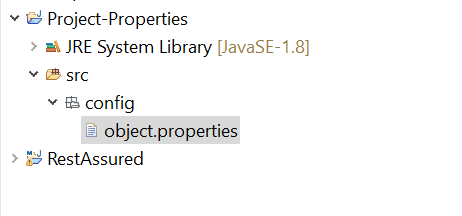
Now let’s put some values inside this object.properties.
name=Deepak
job= Software Engineer
age=30
Now let’s create another package where we will call this properties file
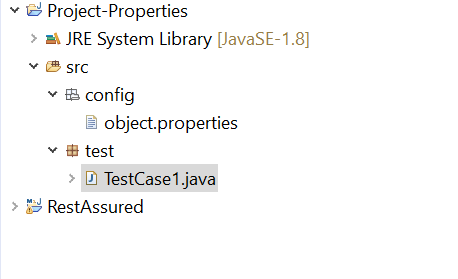
package test;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.util.Properties;
public class TestCase1 {
public static void main(String[] args) throws IOException {
Properties prop = new Properties(); // Creating instance of properties class of Java
FileInputStream fis = new FileInputStream(System.getProperty("user.dir")+"/src/config/object.properties");
prop.load(fis);
System.out.println(prop.getProperty("name"));
System.out.println(prop.getProperty("job"));
}
}
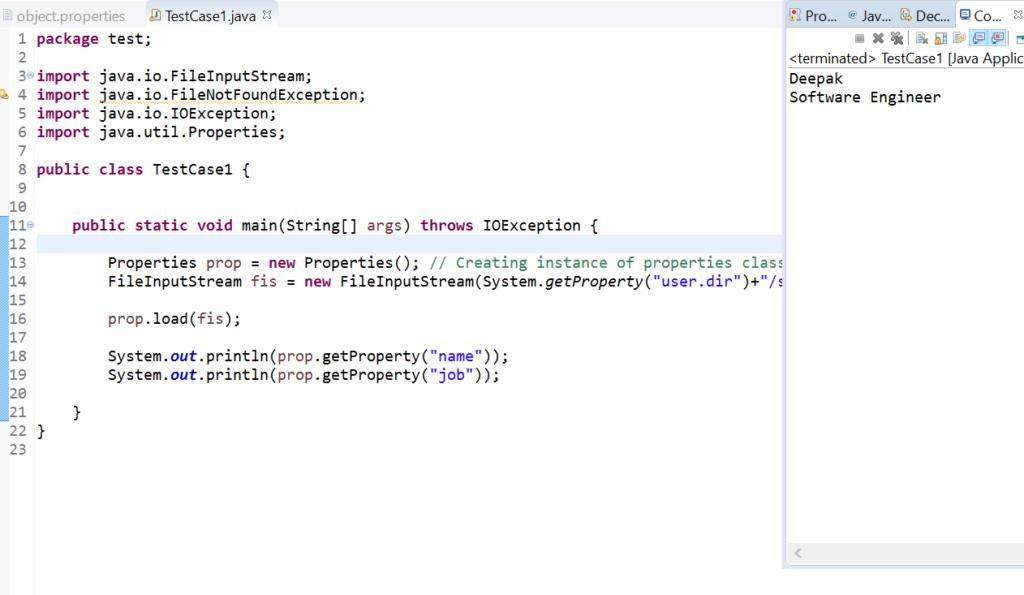
Java Stream
Java streams are used to write/read from a file.
Below code shows how to write to a .txt file
package test;
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
public class TestCase2 {
public static void main(String[] args) throws IOException {
File f = new File(System.getProperty("user.dir")+"/myTextFile.txt");
FileWriter fw = new FileWriter(f,false);
BufferedWriter writer = new BufferedWriter(fw);
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 3; j++) {
int num = (int)(Math.random()*100);
writer.write(num+"\t");//use a , to write to csv
}
writer.newLine();
}
writer.close();
}
}
Below code shows how to read from file
package test;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileReader;
import java.io.IOException;
public class TestCase3 {
public static void main(String[] args) throws IOException {
File f = new File(System.getProperty("user.dir")+"/myTextFile.txt");
FileReader fw = new FileReader(f);
BufferedReader reader = new BufferedReader(fw);
//System.out.println(reader.readLine());
String line = null;
//if above line of code is not written then it will skip the first line
while((line=reader.readLine())!=null) {
System.out.println(line);
}
reader.close();
}
}
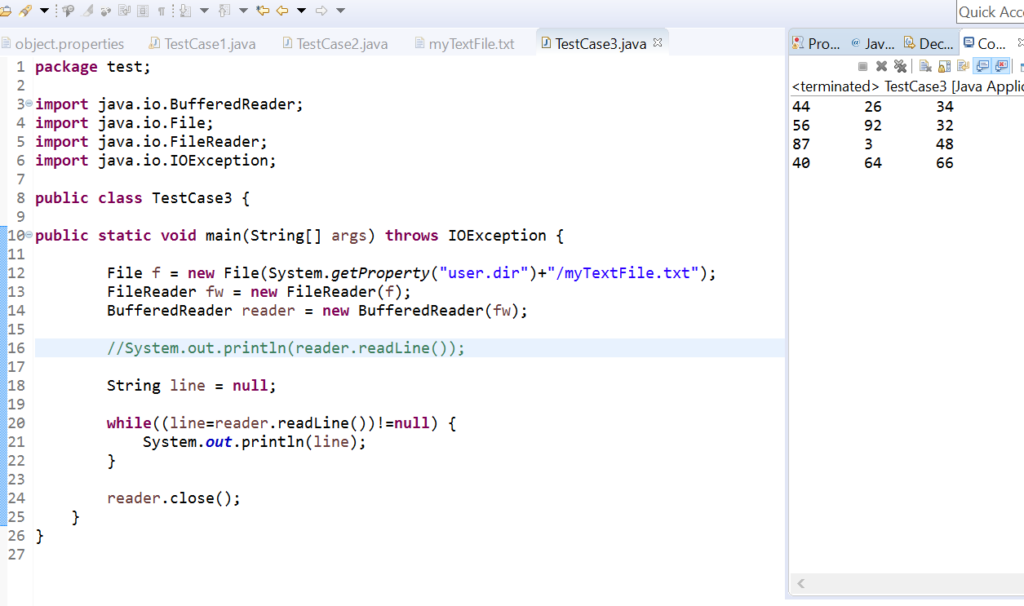
Intriguing post. I Have Been pondering about this matter,
so a debt of gratitude is in order for posting. Entirely cool post.
Thanks!
Best regards,
Harrell Cannon
This is article you shared great informationIi have read
it thanks for providing such a excellent Blog for reader.
King regards,
Thompson Dencker
I like this website – its so usefull and helpfull.
There are many things so much info on it.
King regards,
Thompson Dencker
Enjoyed reading the article above, really explains everything in detail,the article is very interesting and effective.
Best regards,
Balle Schneider