So as to understand asynchronous calls, let’s simulate a server behavior in our code.
Below I am writing a code which will be returned in 2 seconds time. Using setTimeout() function I am trying to simulate the behavior of the server. Let’s jump into practical.
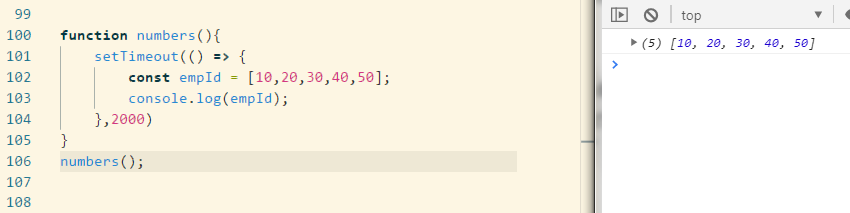
The above result will be displayed after 2 seconds. We are considering that this result has been returned by the server after 2 seconds.
Now we will do some processing on the empId being received, which will be returned by the server after 2 seconds again. Here is the code for it. We will write a callback inside a callback as below:
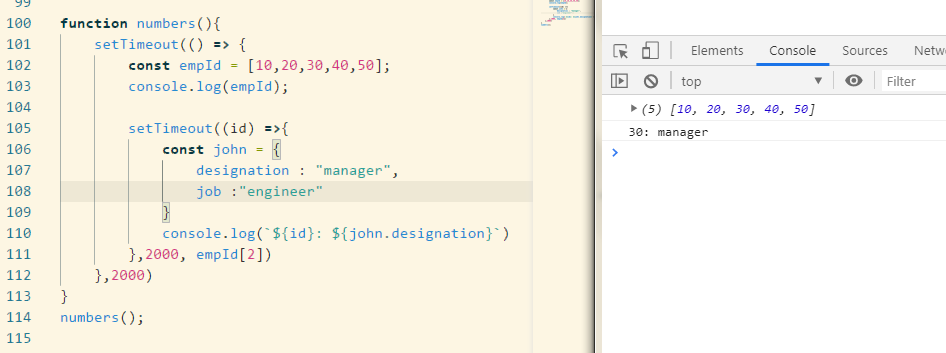
Here we have written a callback inside a callback. The output of the 2nd callback would be displayed after 2 second after the output of the 1st callback.
In the second callback we have passed the empId index 2 as the parameter to the second callback. Let’s write one other callback inside the second callback assuming we want to again process some data.
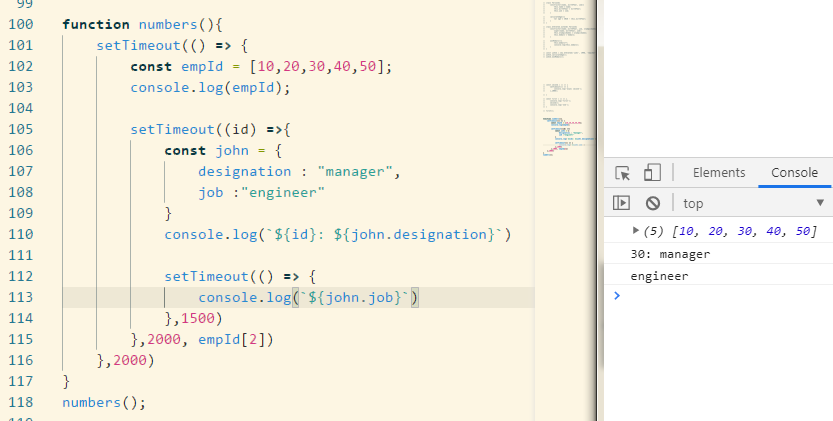
Similarly we have written another 3rd callback. As you can see that it has taken up a triangle structure and there is a lot of code to understand and manage. This triangle structure of the callback is known as callback Hell. That is the reason we have shifted from callbacks to Promises in ES6.
It’s time to have a look at the promises.
Promises
Promises is developed in ES6 specially to deal with asynchronous Javascript.
- Object that keep track about whether a certain event has already happened or not
- Determines what happens after the event has happened
- Implements the concept of the future value that we are expecting. It is similar to saying that “hey get me a value from the server in the background, and then promise, promises us to get that data in the future”
State of Promises
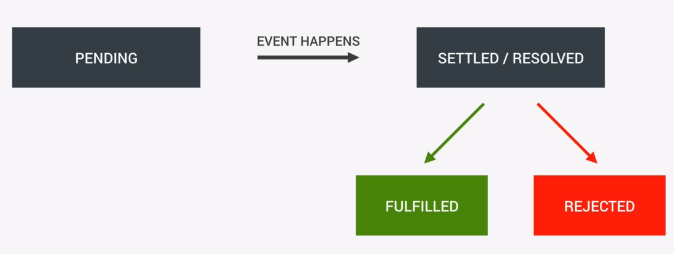
We can produce and consume promises. When we produce promises, we create a new Promise and send a result using that promise. When we consume it, we can use callback function for fulfillment and for rejection of the promise.
Let’s now produce a promise.
Promise is created using the new keyword and we pass in an argument function called executor which is executed as soon as Promise is created. The executor takes 2 callback functions as a parameter resolve and reject. This is because the executor will tell the promise if the execution is successful or not. If it is successful we will call the resolve function else the reject function.

Let’s now create a similar simulation as we did in case of callbacks.
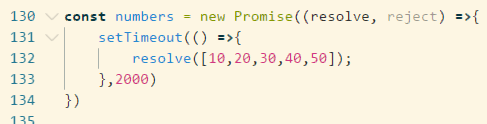
As we know that our setTimeout will always pass, therefore setting a resolve function which will return THE RESULT of the promise if the promise is successful after 2 seconds.
In the above case we do not need a reject function, but when the data is coming from the actual server, we would be requiring a reject function as well.
Its time now to consume the promises. Promises are consumed using then and catch.
Then method allows us to add an event handler for the case that the promise is fulfilled, which means there is a result. Therefore in them we need to pass a callback function which handle what we do in case the promise was successful.
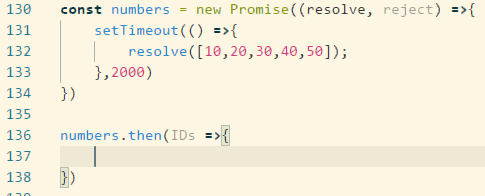
The Ids in line number 136 will hold the result of the Promise, which in this case will be the arrays.
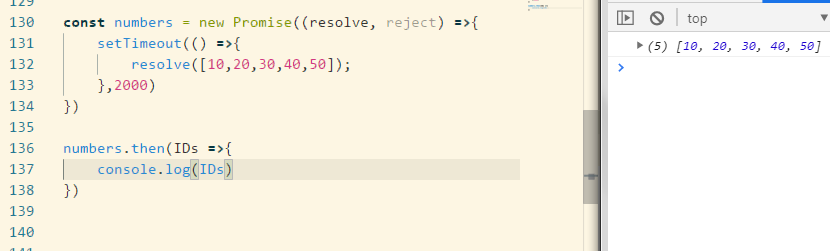
Now let’s have a look at the catch function which will handle the case if the promise has failed.
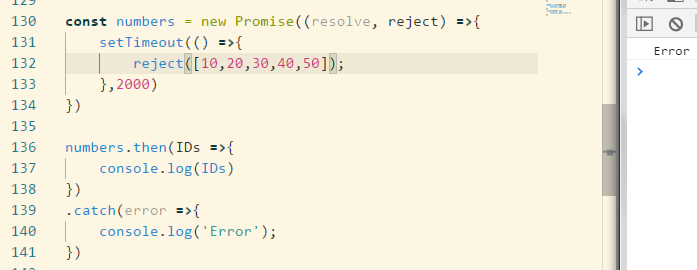
As in the starting of the blog we have seen how we have created nested callback functions. Now let’s now replicate the same things.
We will create a function which will receive the empId and which will then return a Promise.
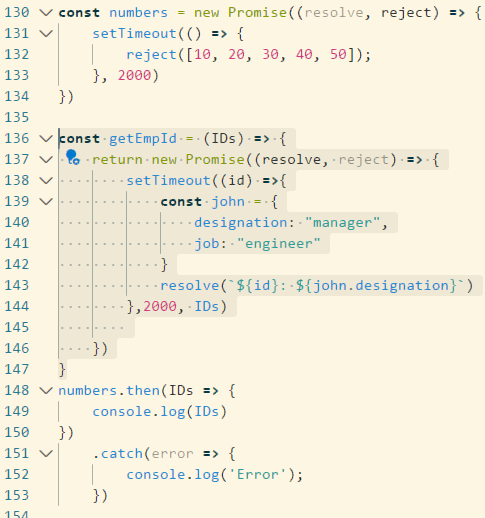
We will pass in IDs(line no 136) while calling the function, which is then passed to line number 144 IDs and further passed to line 138 id.
Consuming the above Promises
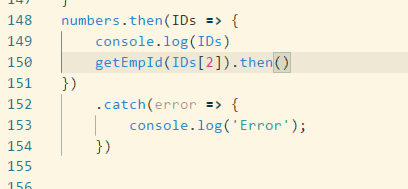
getEmpId returns a promise, that is why we have again used the. However, this is still not a good solution as we have not resolved the problem of callback hell.
We can do this using chaining where we will return a promise from the then method and again will use then and make use of chaining.
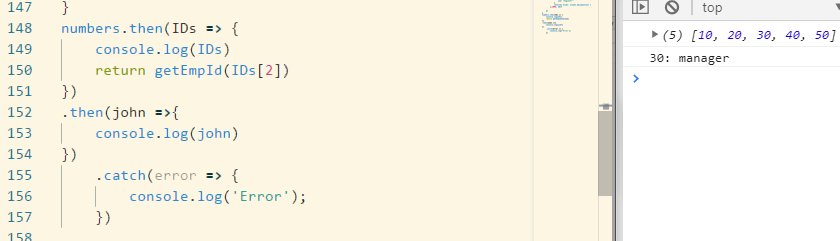
line 150 will returns a Promise and its result is captured in line no 152.
Similarly try doing it with the 3rd promise yourself.