In server streaming API the client will send one request to the server and in response will receive many responses from the server.
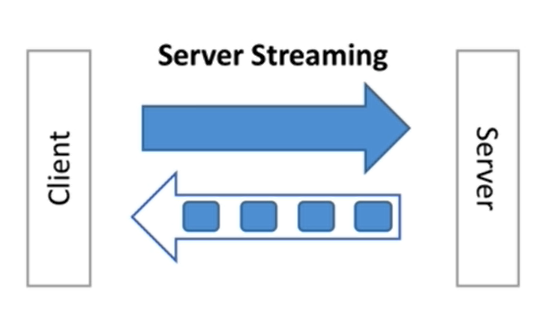
We will develop:
- A streaming server which will take ONE GreetManyTimesRequest that contains a Greeting
- It will return many GreetManyTimesResponse that contains a result string
Step 1 – Creating proto files and compiling it
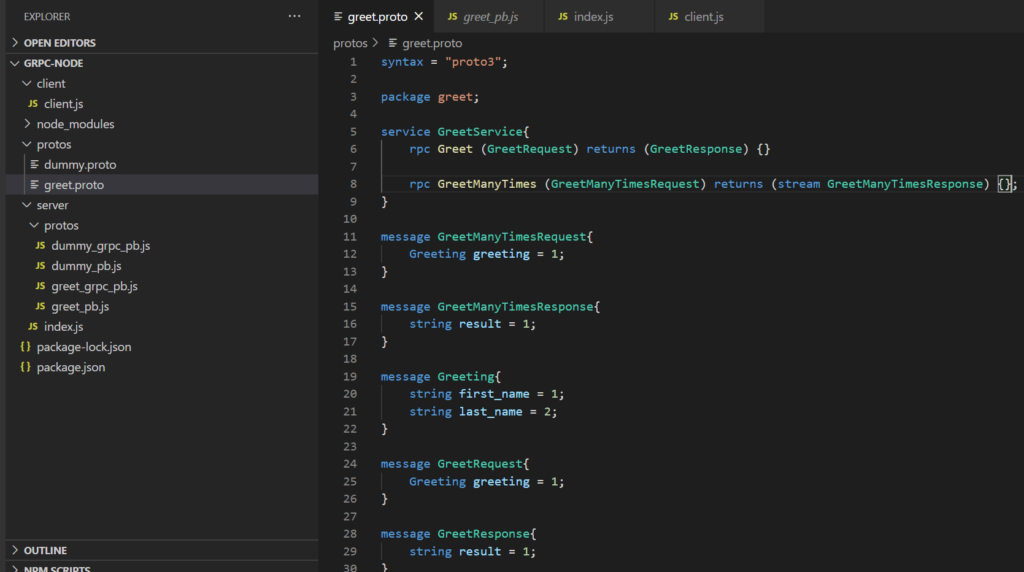
compiling
GRPC_TOOLS_PLUGIN="$HOME/AppData/Roaming/npm/grpc_tools_node_protoc_plugin.cmd" && C:/Users/deepak.sood/Downloads/protoc-3.13.0-win64/bin/protoc.exe -I=. ./protos/greet.proto --js_out=import_style=commonjs,binary:./server --grpc_out=./server --plugin=protoc-gen-grpc=$GRPC_TOOLS_PLUGIN
Step 2 – implementing server
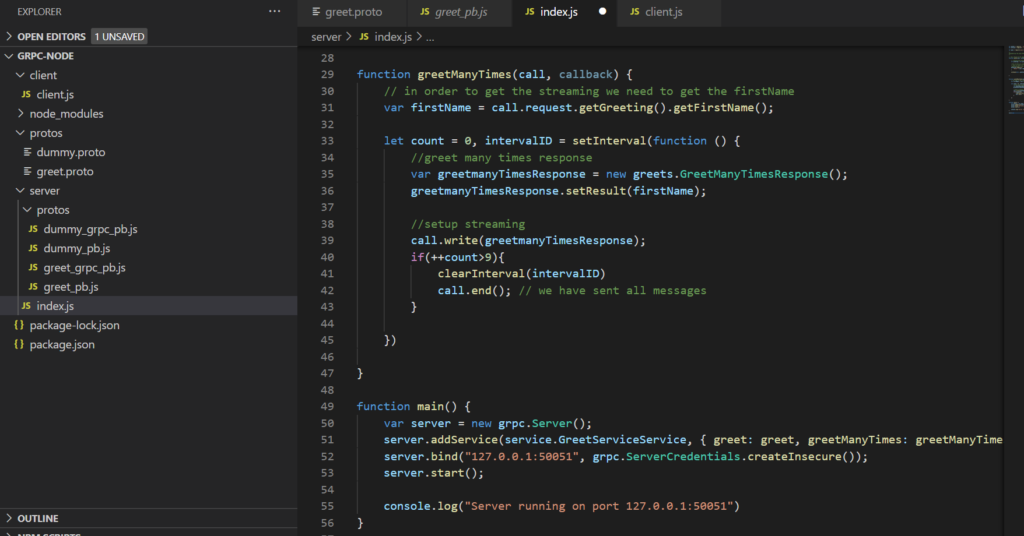
var greets = require('../server/protos/greet_pb');
var service = require('../server/protos/greet_grpc_pb');
var grpc = require('grpc');
/*
Implementing the greet RPC method
*/
// this should be the same name as our API as present in greet_grpc_pb file
function greet(call, callback) {
// creating the actual greeting response
var greeting = new greets.GreetResponse();
//now we have our greet response, so let's now construct the response
// call is used as it will have all the information from line 11 when called
//getGreeting is from the GreetRequest object
greeting.setResult(
"Hello" + call.request.getGreeting().getFirstName()
)
callback(null, greeting); // passed in the greeting to be passed on to the client
}
function greetManyTimes(call, callback) {
// in order to get the streaming we need to get the firstName
var firstName = call.request.getGreeting().getFirstName();
let count = 0, intervalID = setInterval(function () {
//greet many times response
var greetmanyTimesResponse = new greets.GreetManyTimesResponse();
greetmanyTimesResponse.setResult(firstName);
//setup streaming
call.write(greetmanyTimesResponse);
if(++count>9){
clearInterval(intervalID)
call.end(); // we have sent all messages
}
})
}
function main() {
var server = new grpc.Server();
server.addService(service.GreetServiceService, { greet: greet, greetManyTimes: greetManyTimes })
server.bind("127.0.0.1:50051", grpc.ServerCredentials.createInsecure());
server.start();
console.log("Server running on port 127.0.0.1:50051")
}
main();
Let’s test this
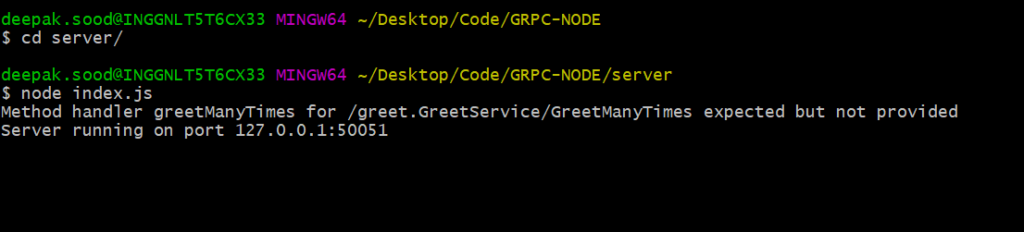
Step 3 – Implementing Client
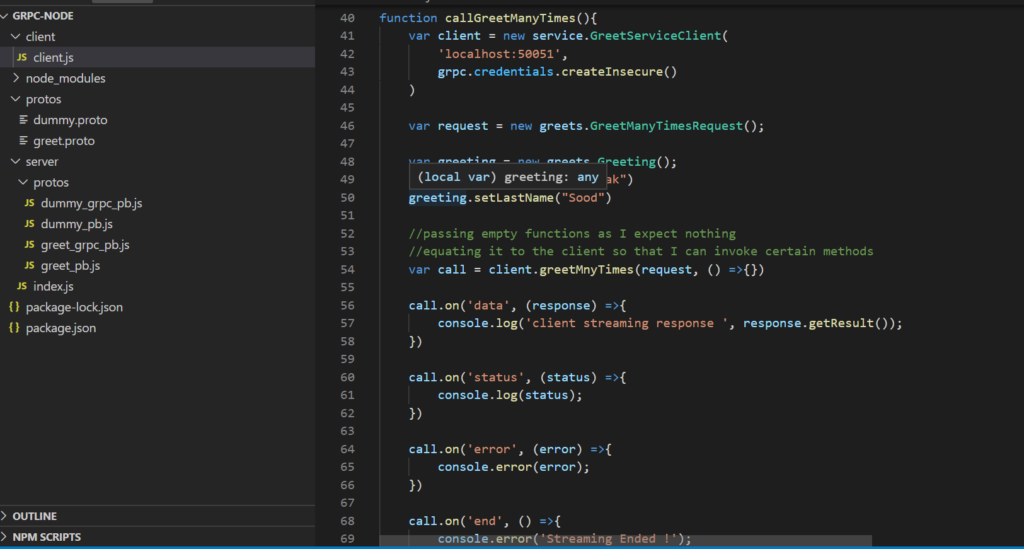
function callGreetManyTimes(){
var client = new service.GreetServiceClient(
'localhost:50051',
grpc.credentials.createInsecure()
)
var request = new greets.GreetManyTimesRequest();
var greeting = new greets.Greeting();
greeting.setFirstName("Deepak")
greeting.setLastName("Sood")
request.setGreeting(greeting);
//passing empty functions as I expect nothing
//equating it to the client so that I can invoke certain methods
var call = client.greetManyTimes(request, () =>{})
call.on('data', (response) =>{
console.log('client streaming response ', response.getResult());
})
call.on('status', (status) =>{
console.log(status);
})
call.on('error', (error) =>{
console.error(error);
})
call.on('end', () =>{
console.error('Streaming Ended !');
})
}
callGreetManyTimes()
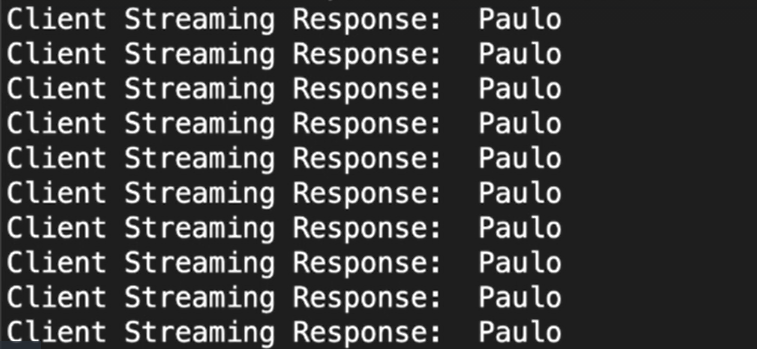
And that’s how it works !!