Starting the Project
Let’s create a cart in this project.
The first step is to break down the components. There will be 3 components corresponding to the app:
- Nav Bar which will have cart icon
- cart list which will have list of items/products
- footer which will have the total price
Let’s create a sample project
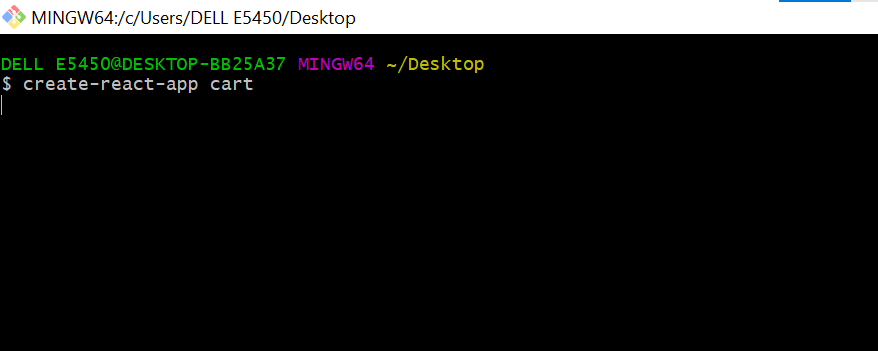
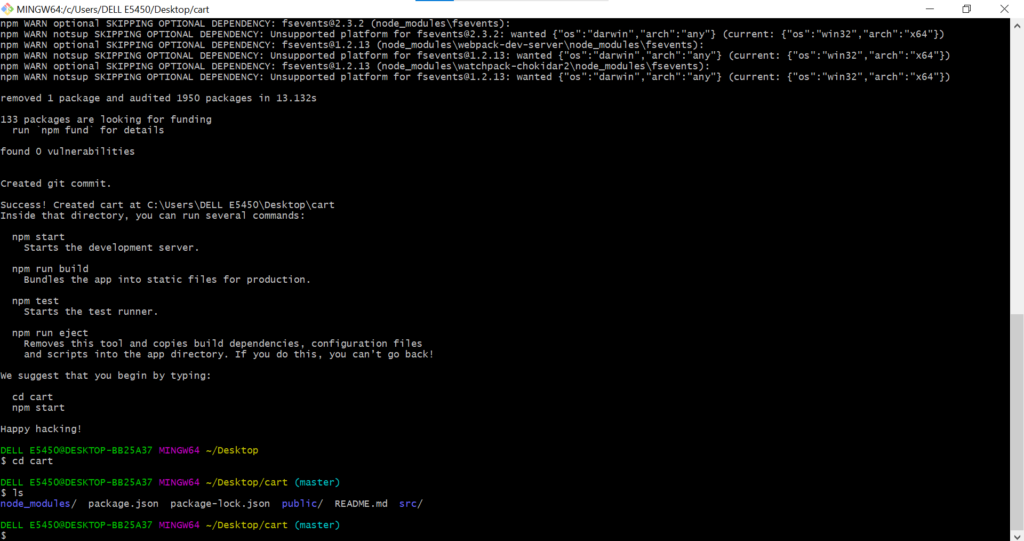
Let’s delete unwanted files from the project
App.test.js, logo.svg, reportWebVitals.js, setupTests.js
In index.js let’s delete the unwanted code.
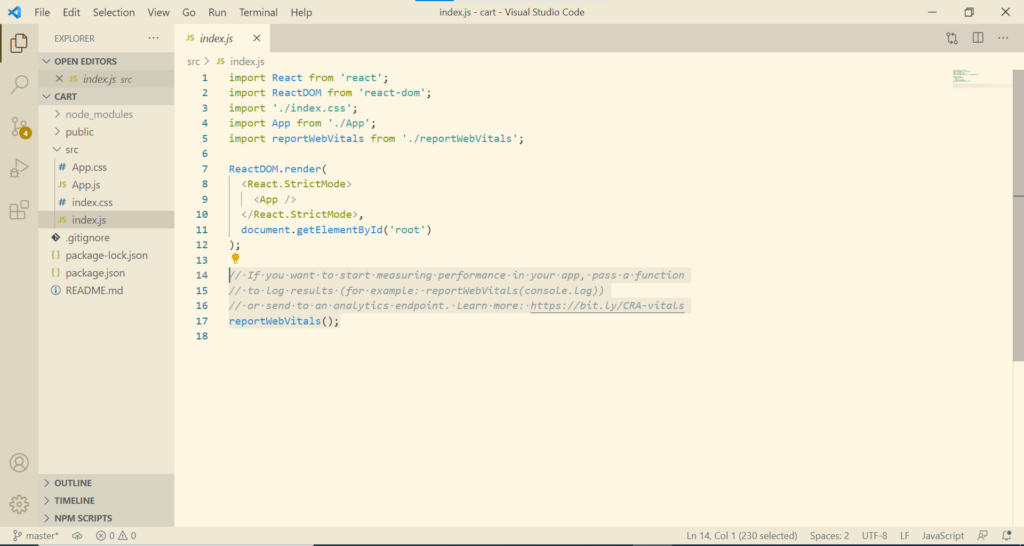
In App.js let’s delete the already written JsX code.
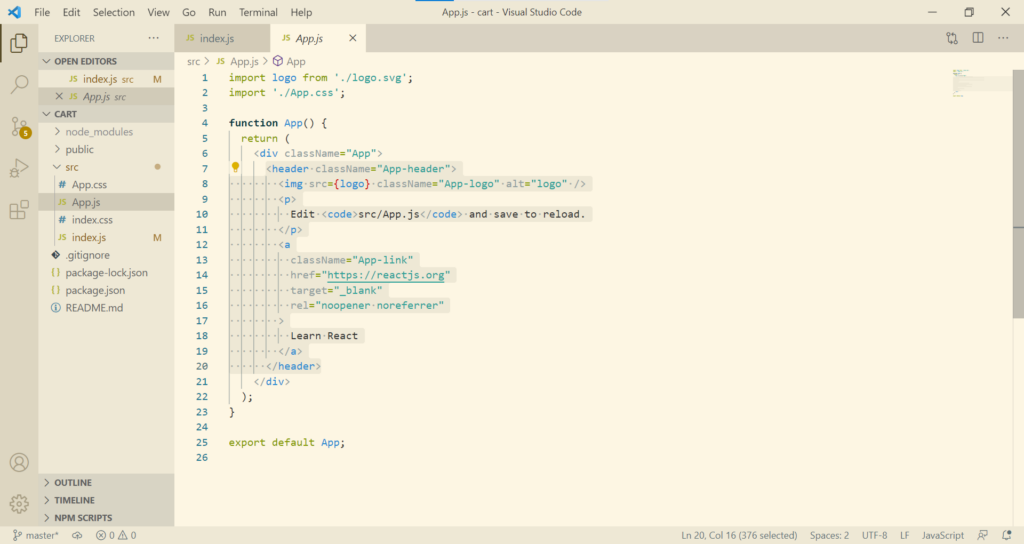
As we have deleted the above code, let’s delete the App.css file and its reference as well.
Now let’s run the project
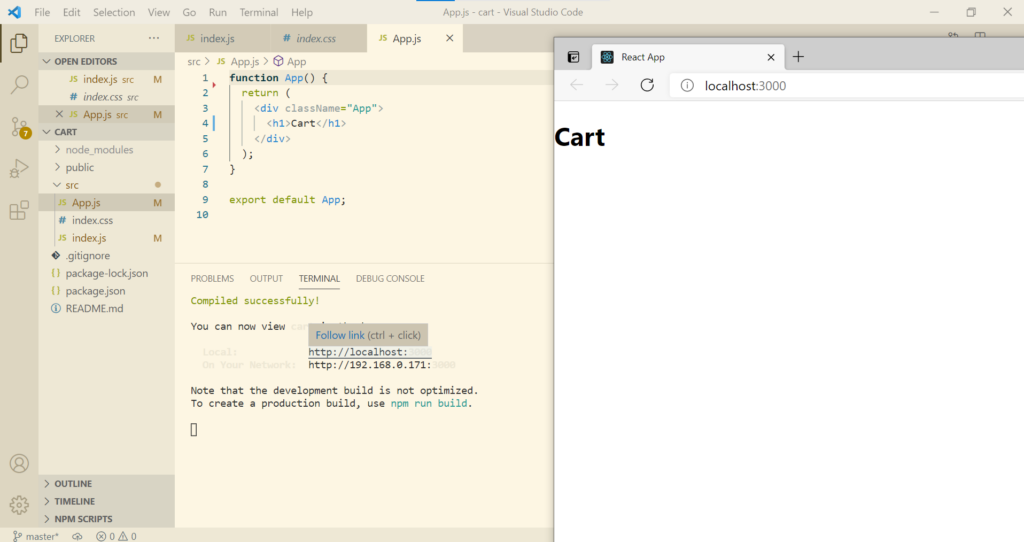
CSS for Cart Item
Now let’s jump to index.css file and delete the unwanted code.
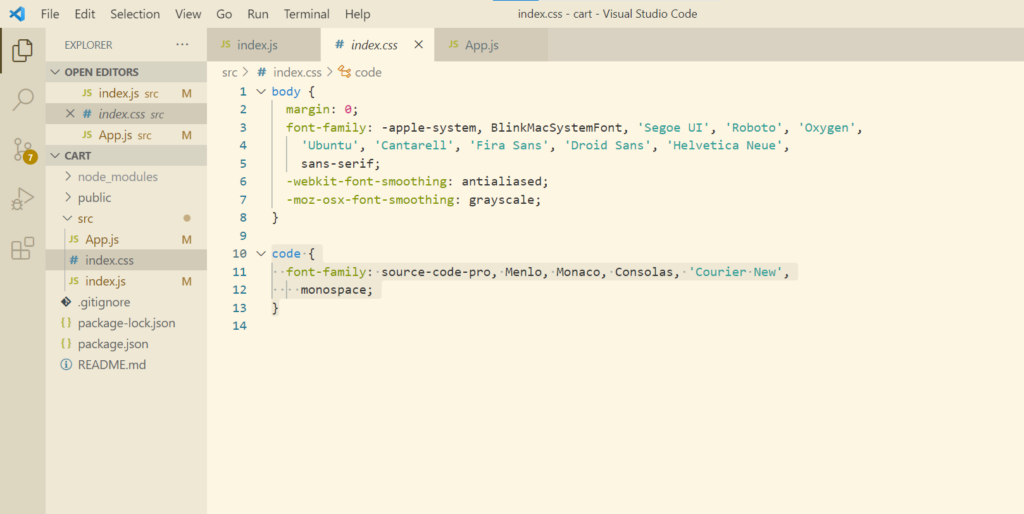
Let’s add some css style for the cart
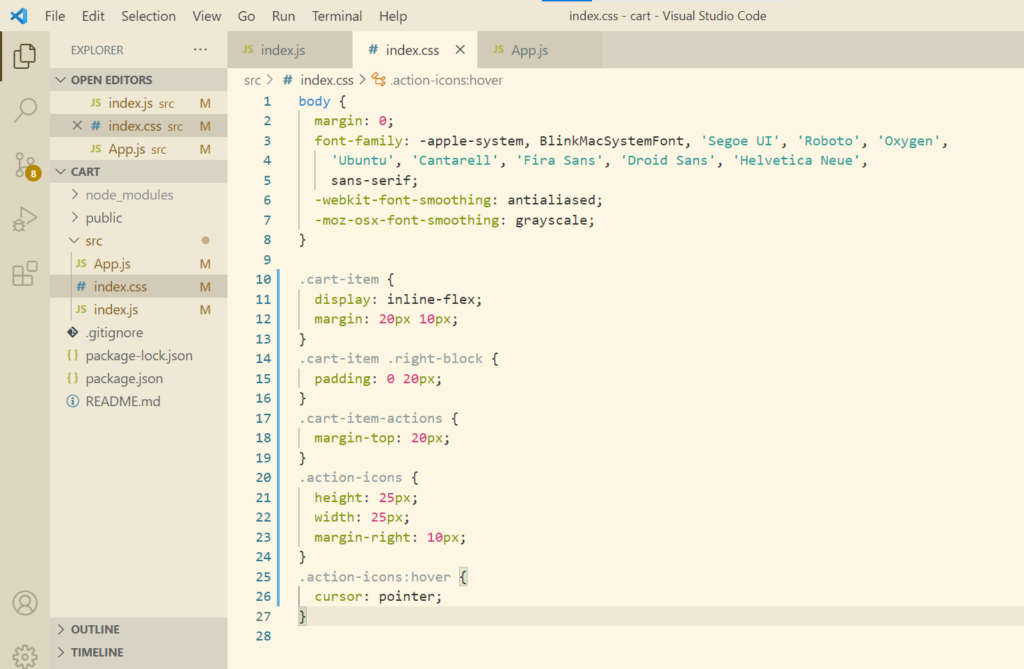
Let’s now understand this CSS with help of some diagram
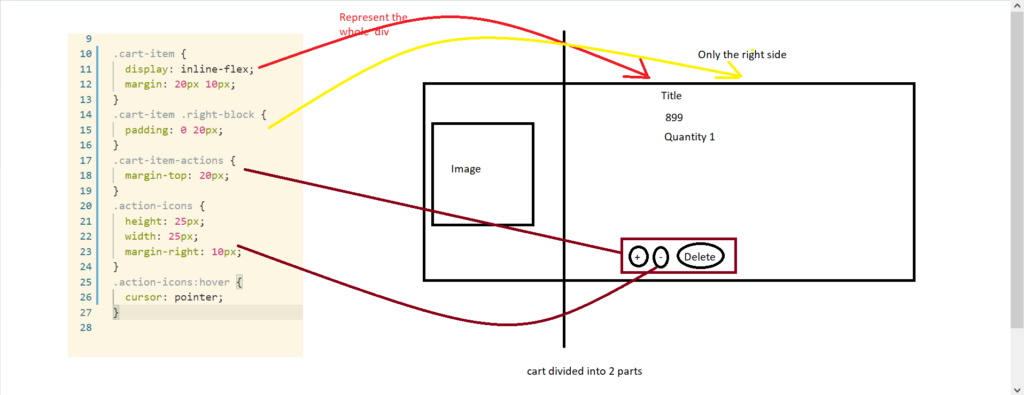
Let’s now start writing the very first component of our app which is the Cart item.
Inside our root component, we will render our cart item. Let’s create cart item CartItem.js
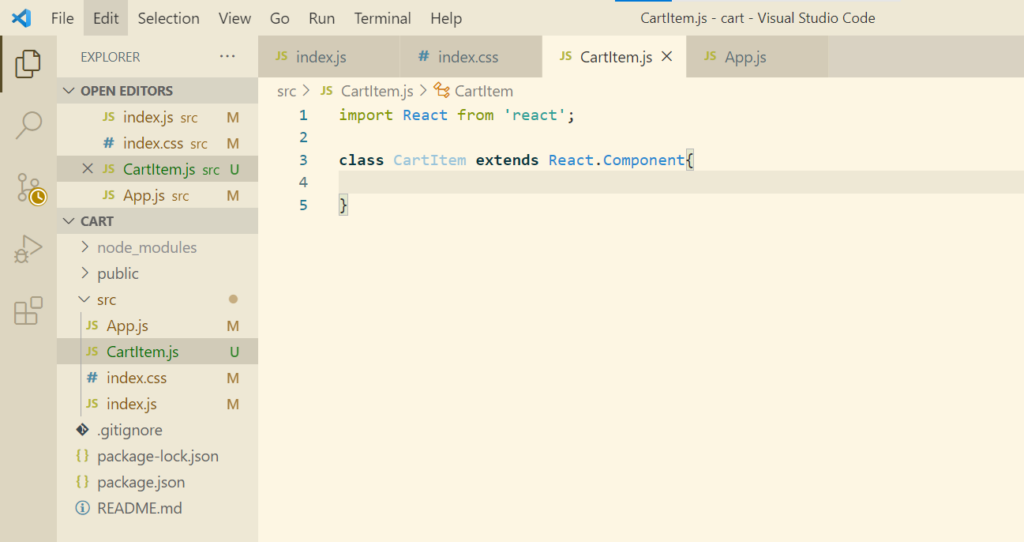
Cart.js is a class component and index.js is a function component as it is having function.
For class component to be a React component we need to give it one method – render() and this method returns a JsX which will describe the UI for that component.
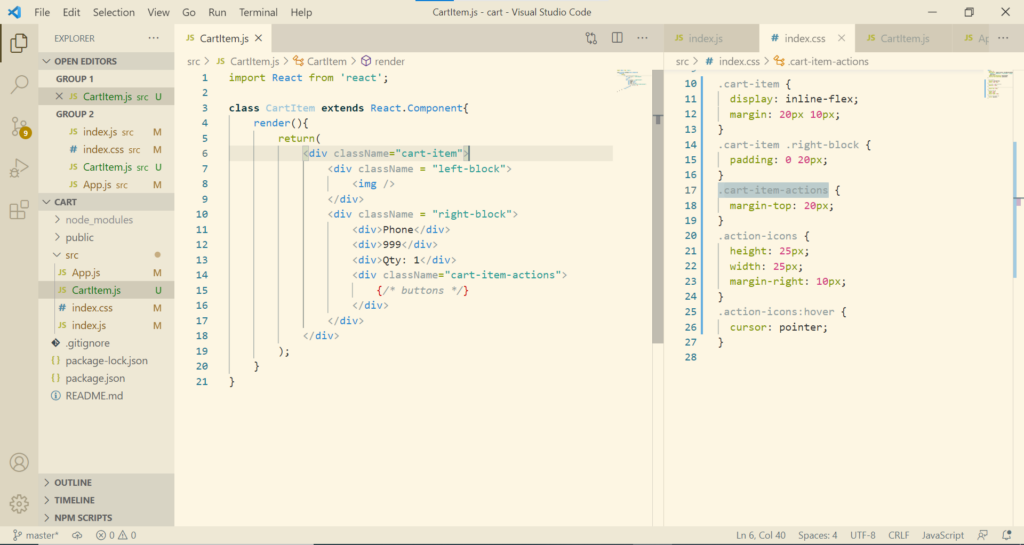
Its time to render this cart item into our root component.
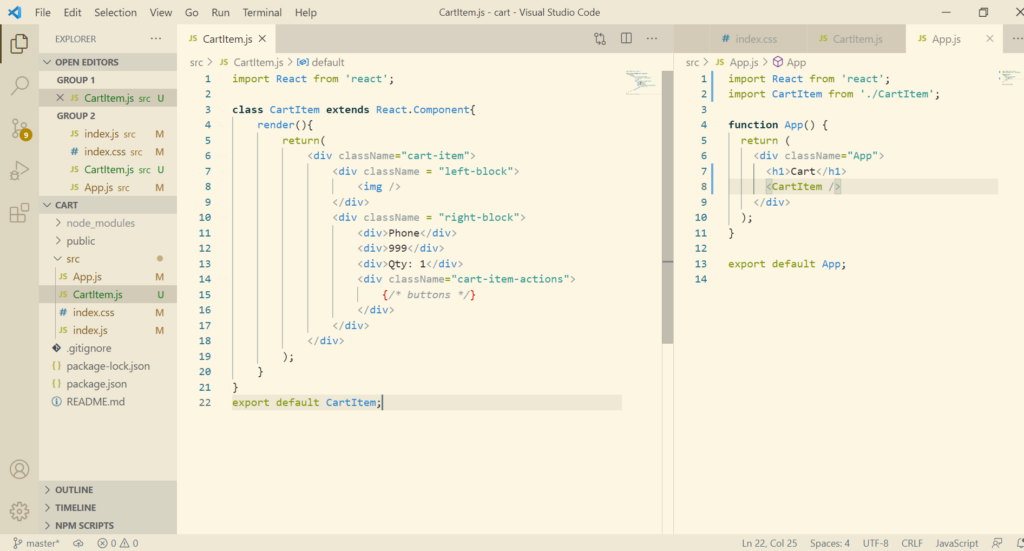
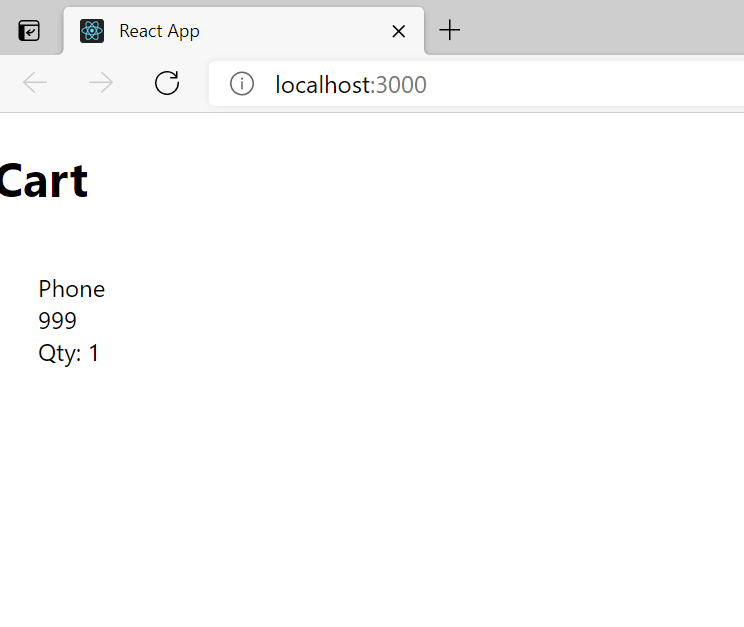
Its time to style items in JsX
Styling elements in JsX
As JsX is not Javascript therefore we cannot style our elements as the traditional way. We need to style our elements using objects.
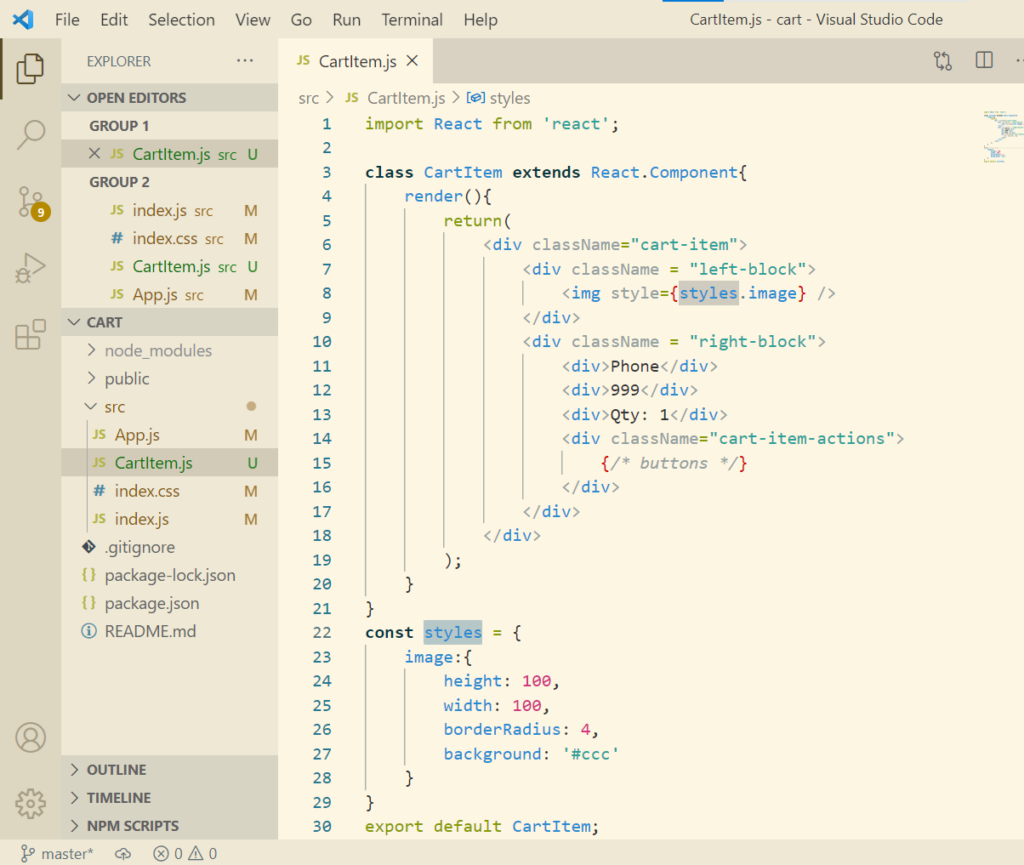
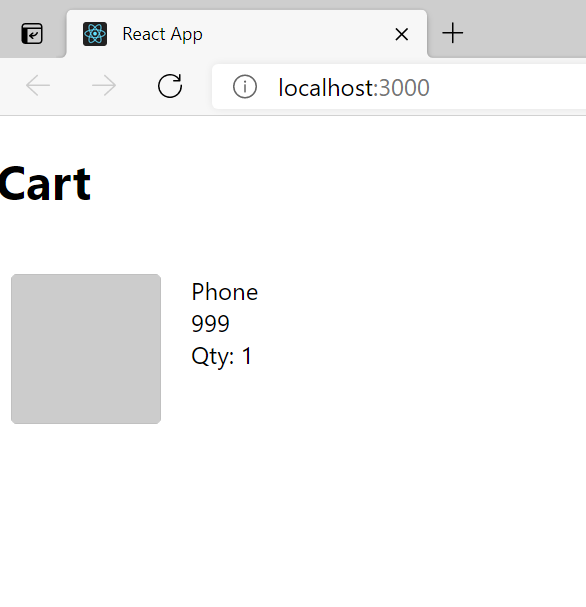
We can directly give these properties in the JsX code as well.
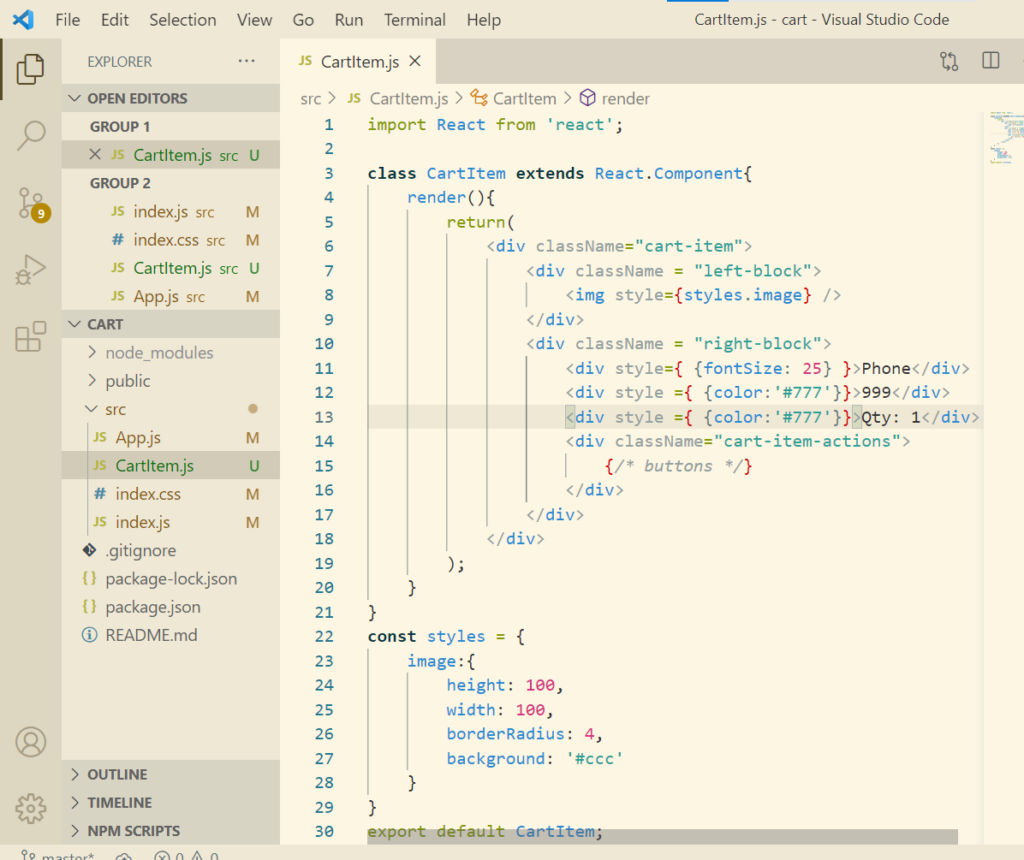
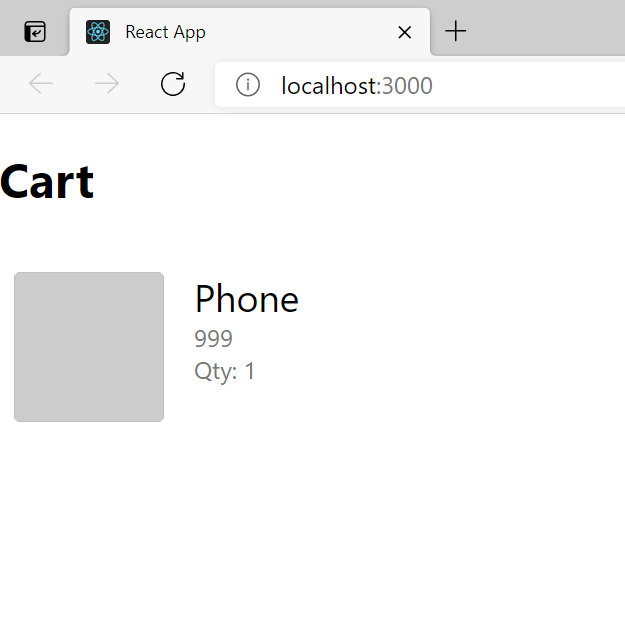
Adding State in CartItem
Before jumping into the state, let’s first add image to the icons
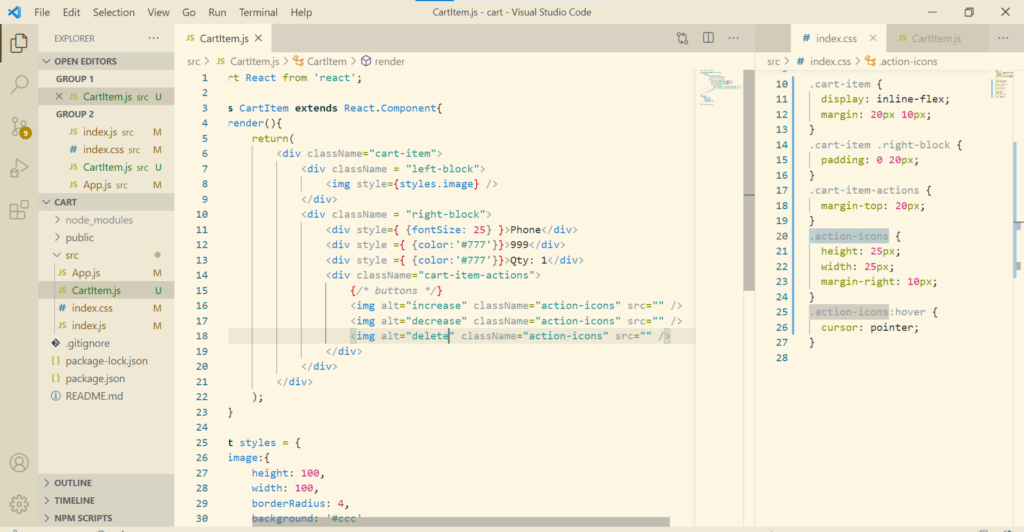
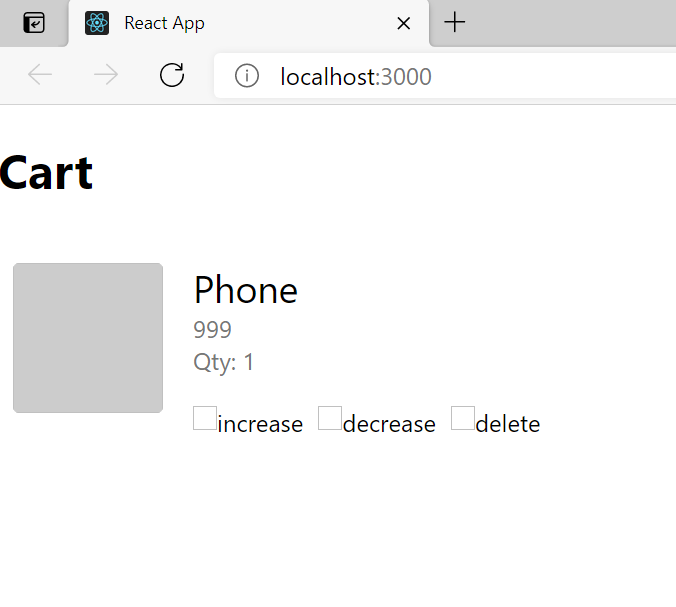
Image can be found at https://www.flaticon.com/
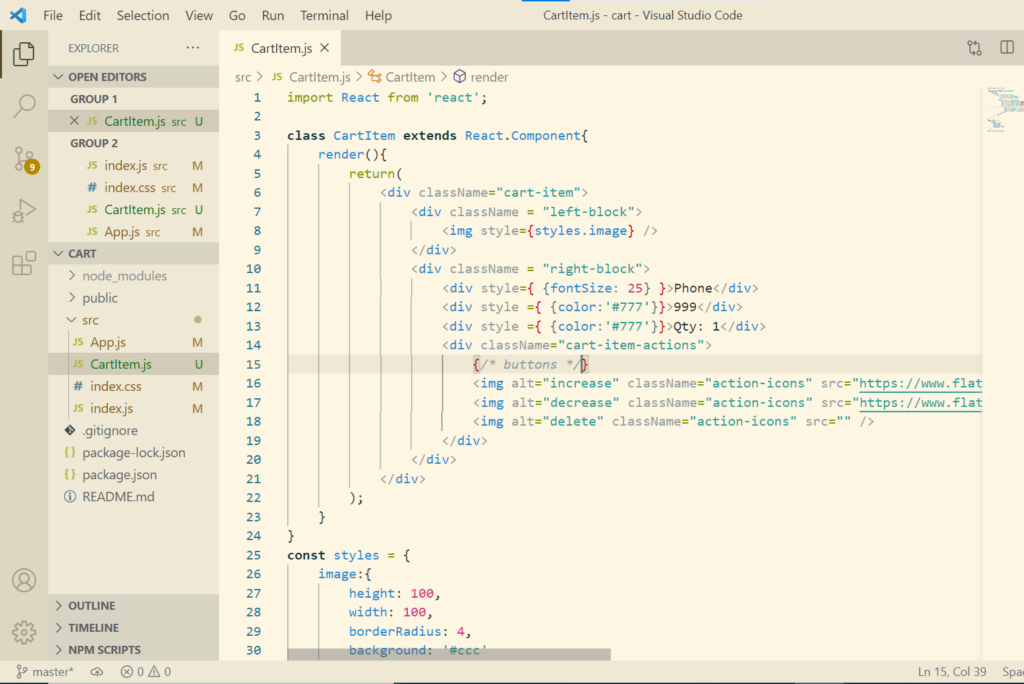
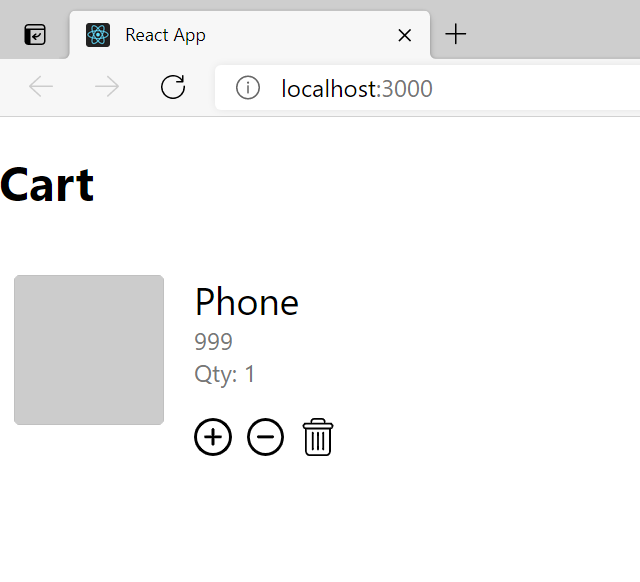
State is just a way to store your local data for a particular component. State is just a plain JS object.
We need to define a constructor so as to define a state.
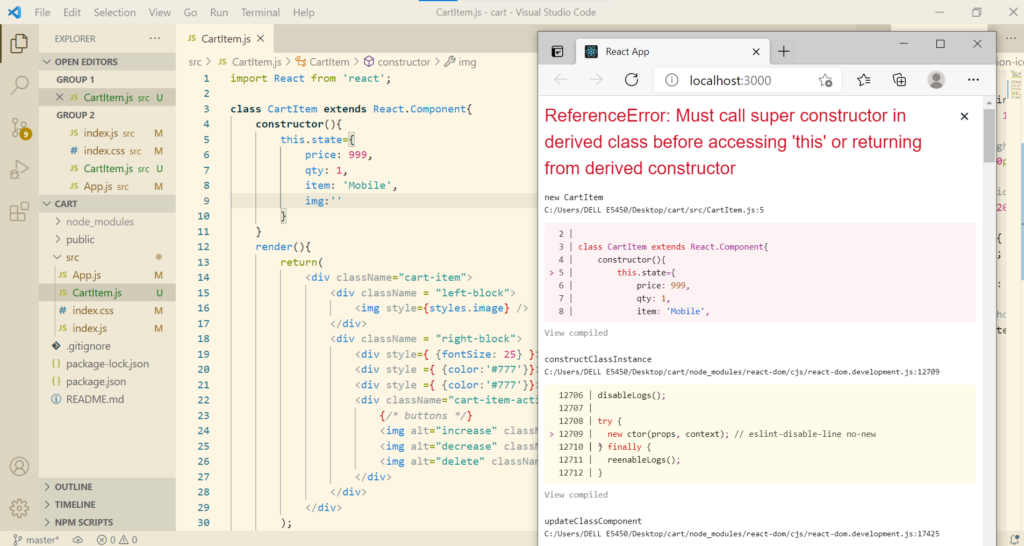
Let’s rectify it.
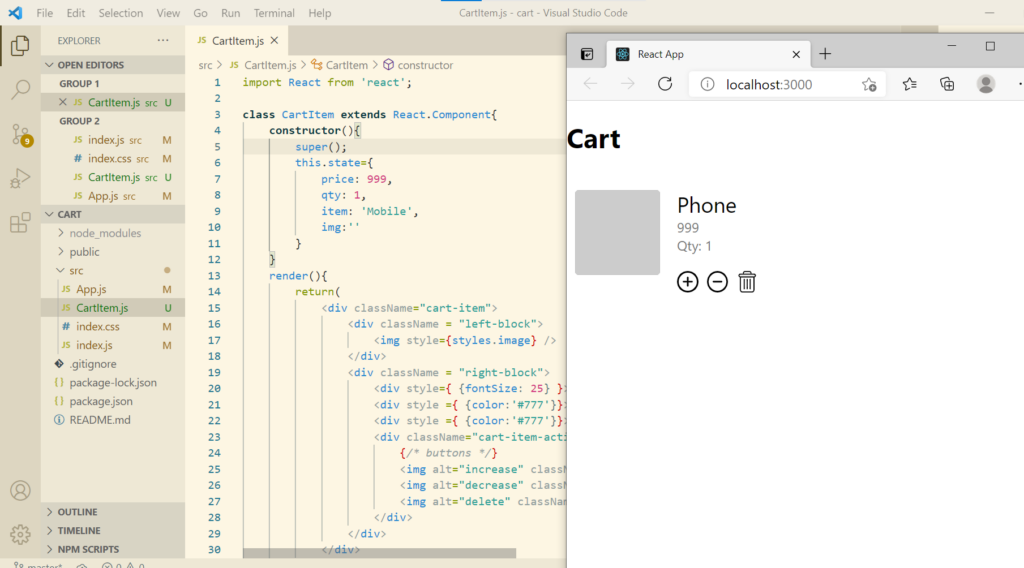
Let’s now use this state in our component.
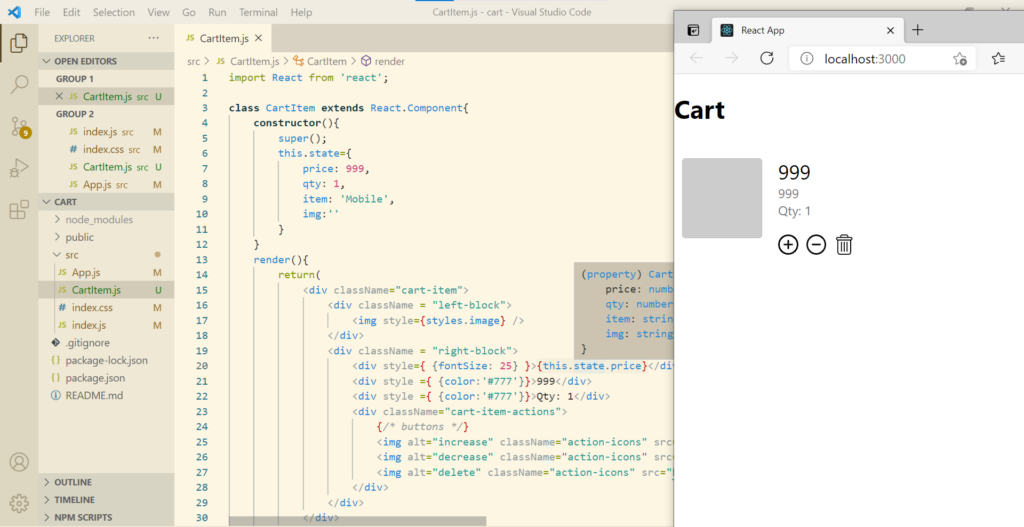
Better way is by using destructuring of objects.
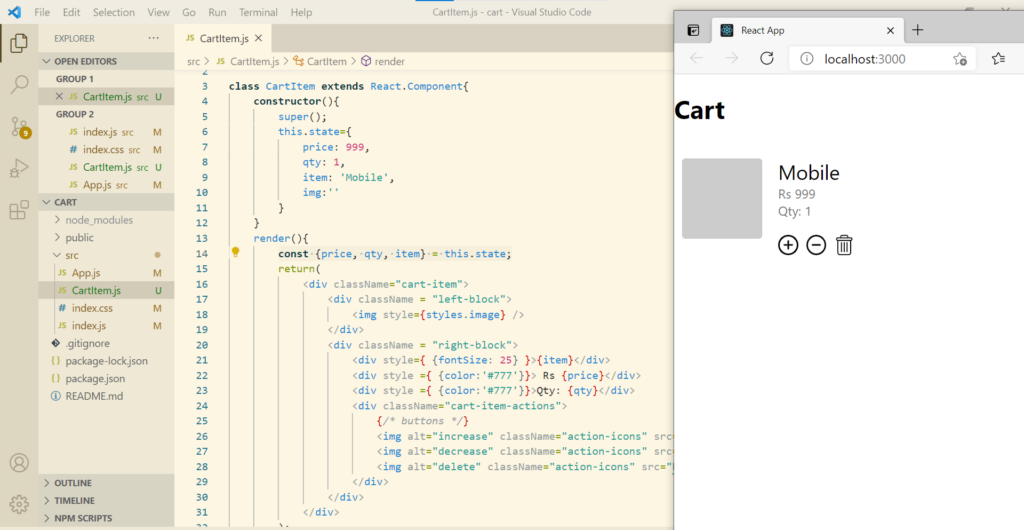
Increase Quantity
So as to increase the quantity we need to add an event listener to the increase quantity image.
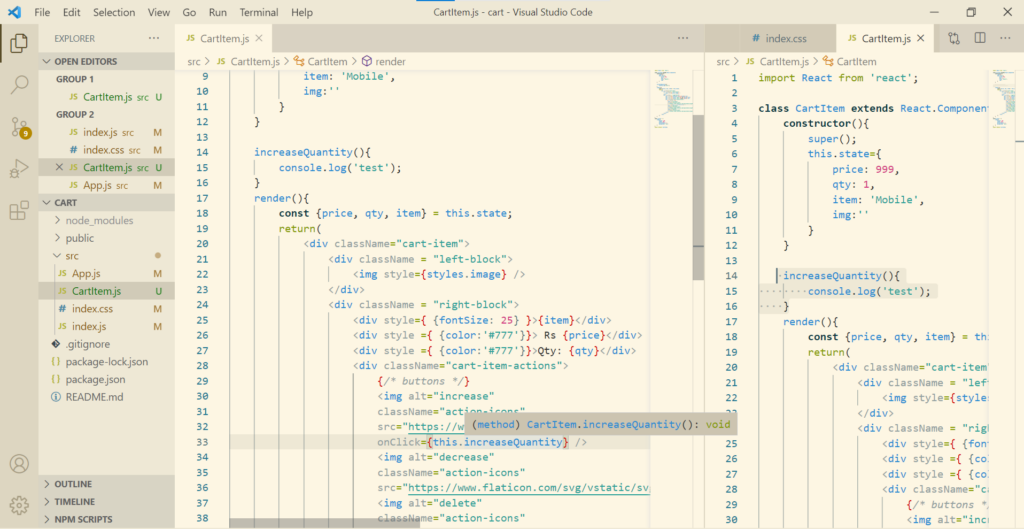
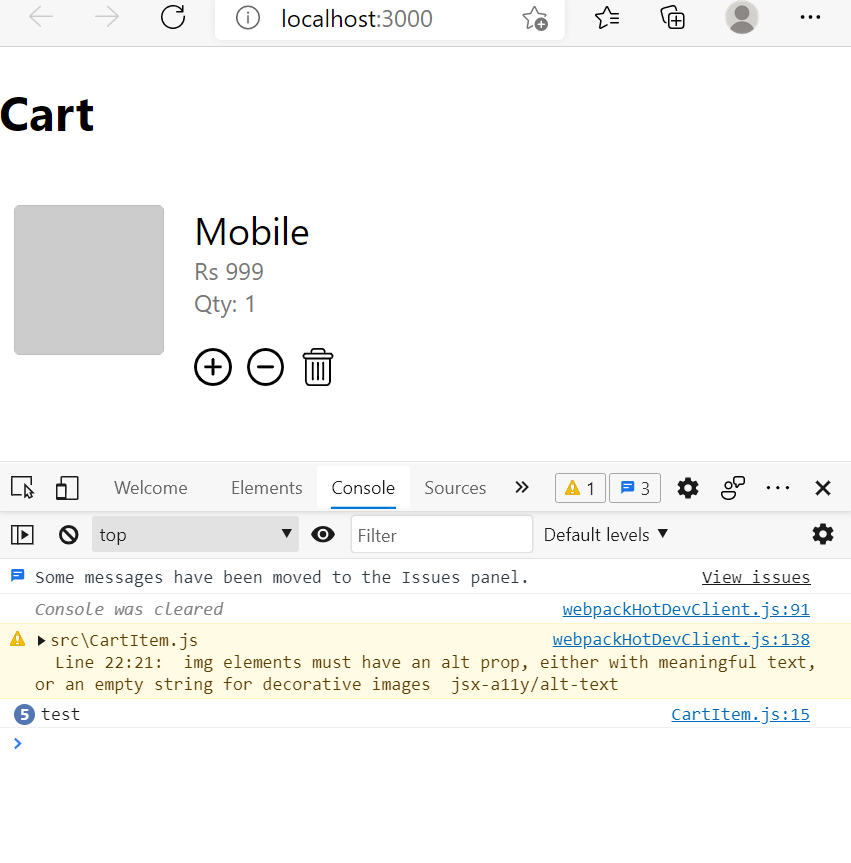
Now let’s change the quantity on the click.
Let see what this state looks like:
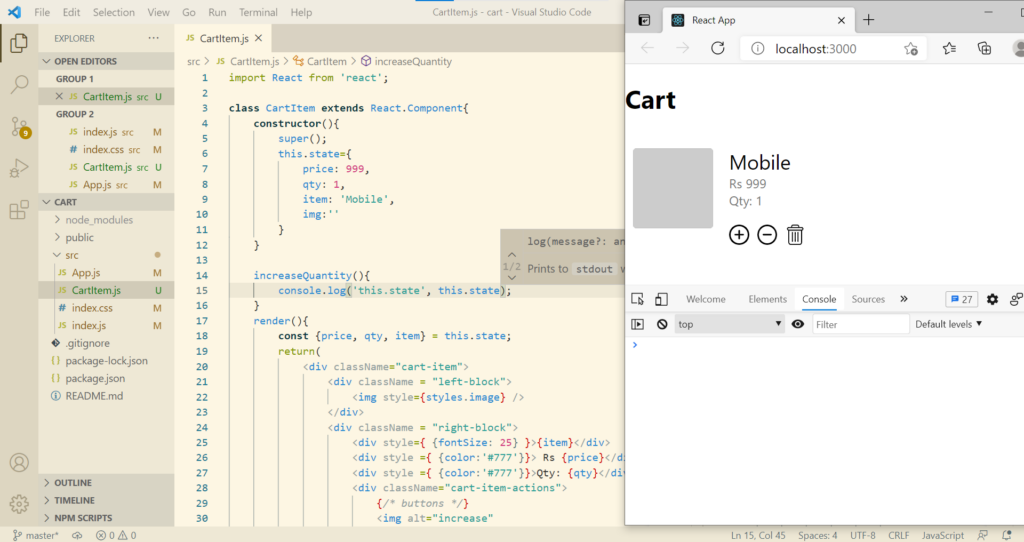
Let’s click on the + icon now.
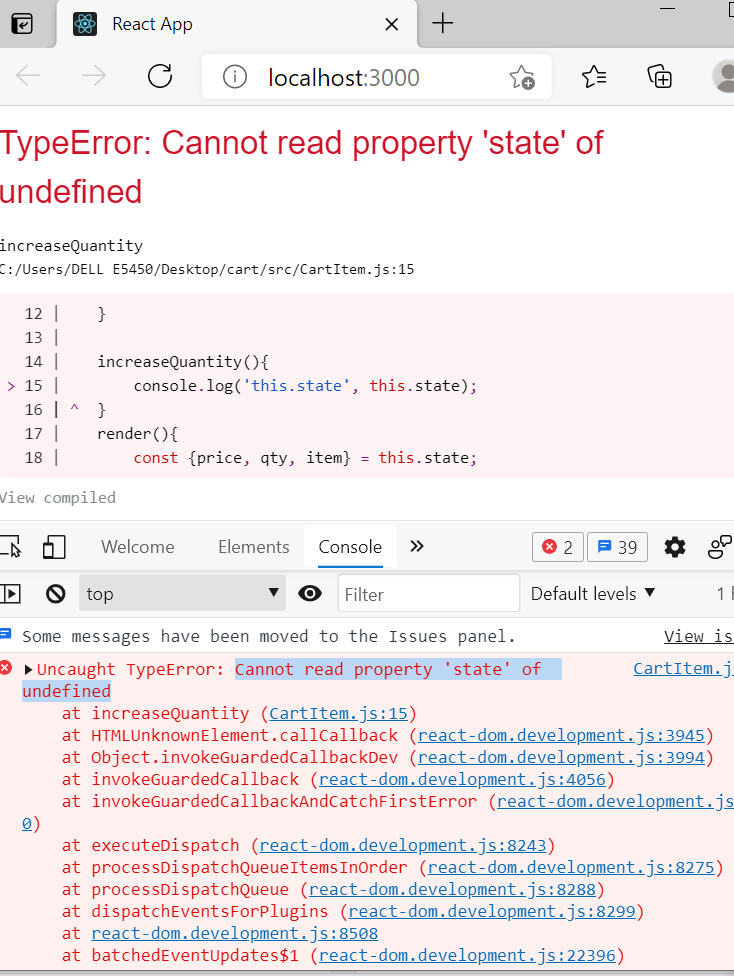
We got an error that state is undefined. Let’s check the value of this.
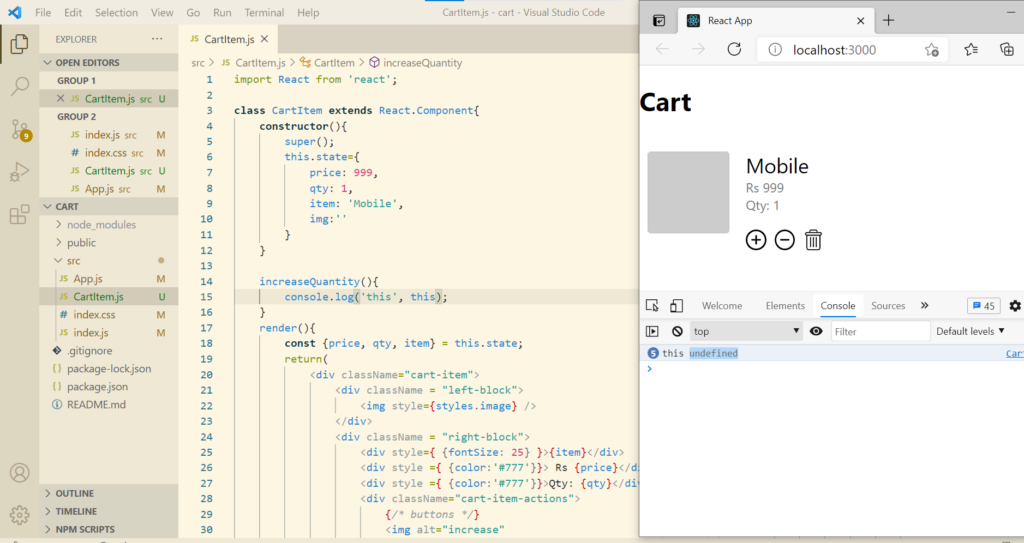
Why ?
This is because the value of increasedQuantity function is lost when it is called in line number 33.
Let’s understand this concept with help of an example
Consider a class with a constructor and a get method
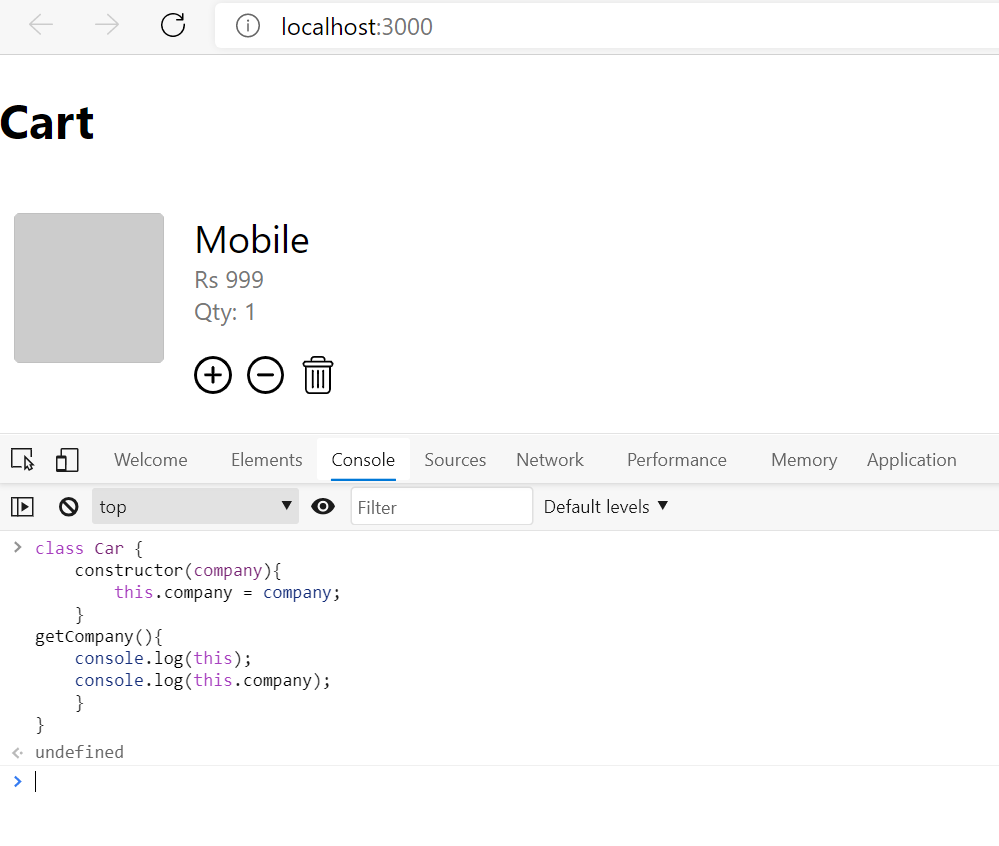
Let create an object now.
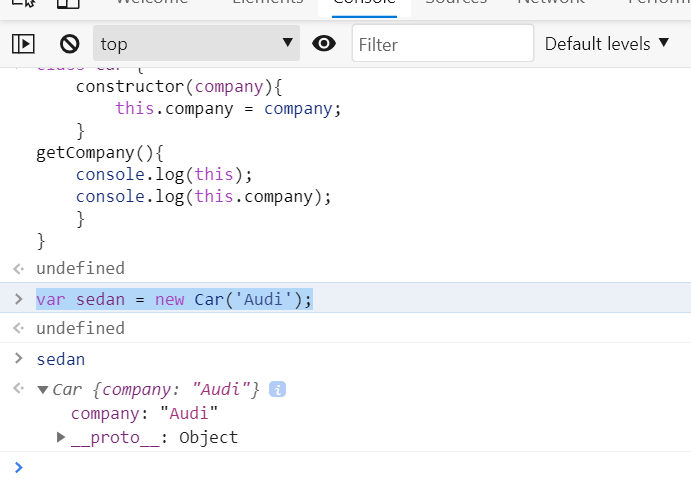
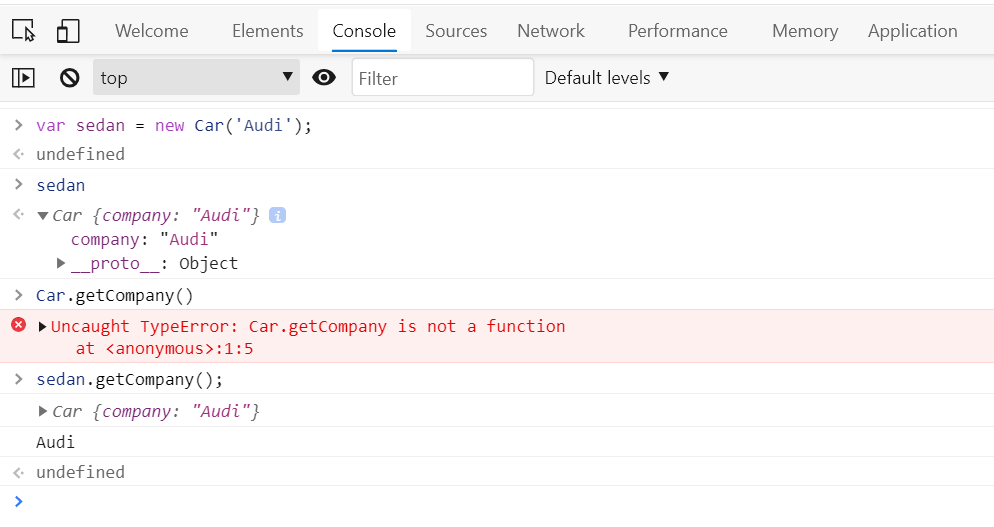
Let’s write var func = sedan.getCompany(); Here I am giving reference of my getCompany to func.
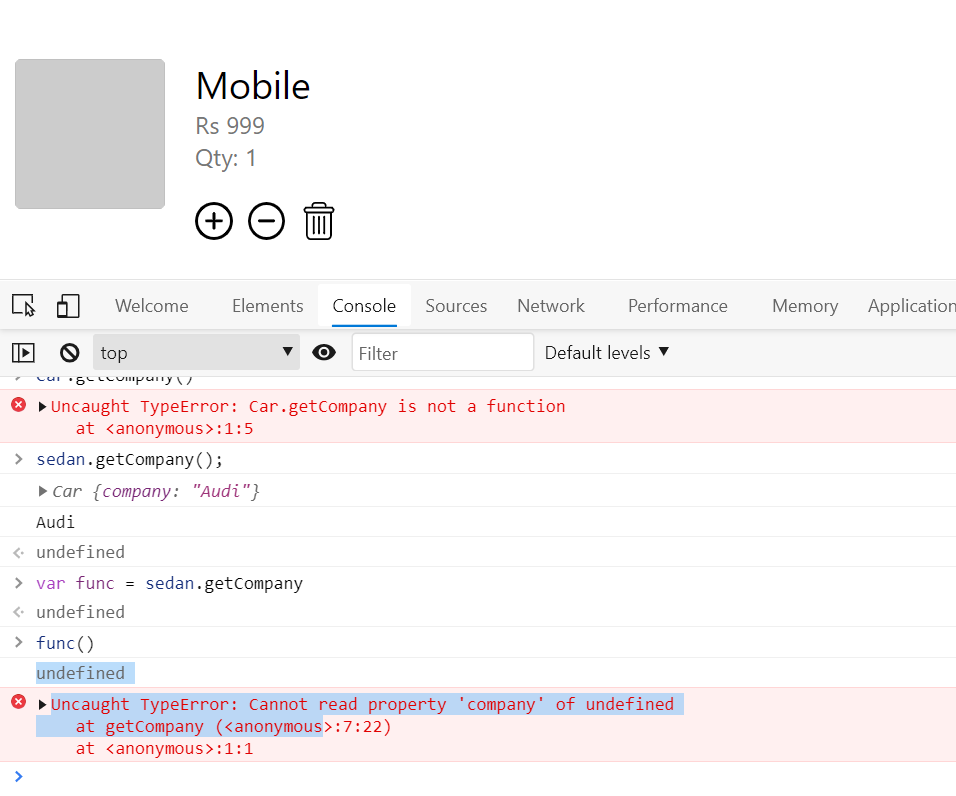
The similar thing is happening in our React.
When we are attaching the event listener, passing a reference to our increase Quantity function and when it internally call that function, the value of this gets lost.
Solution
1st way – By binding
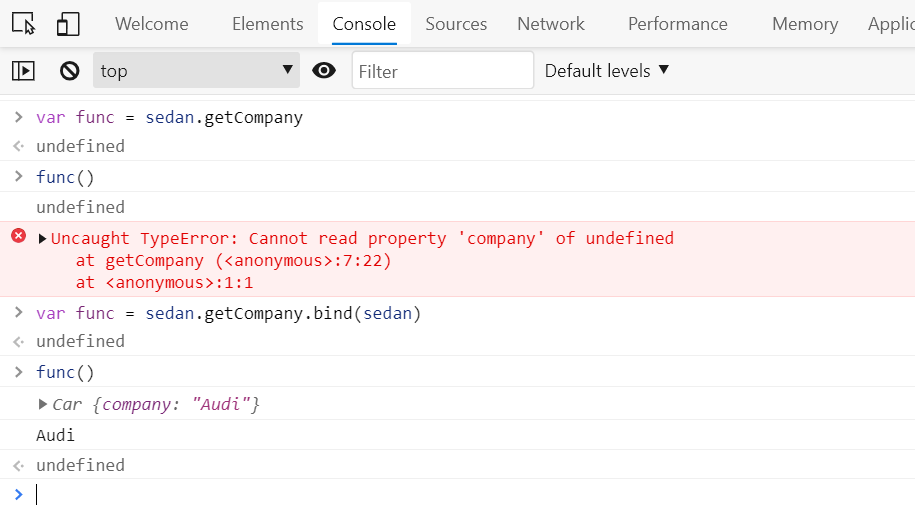
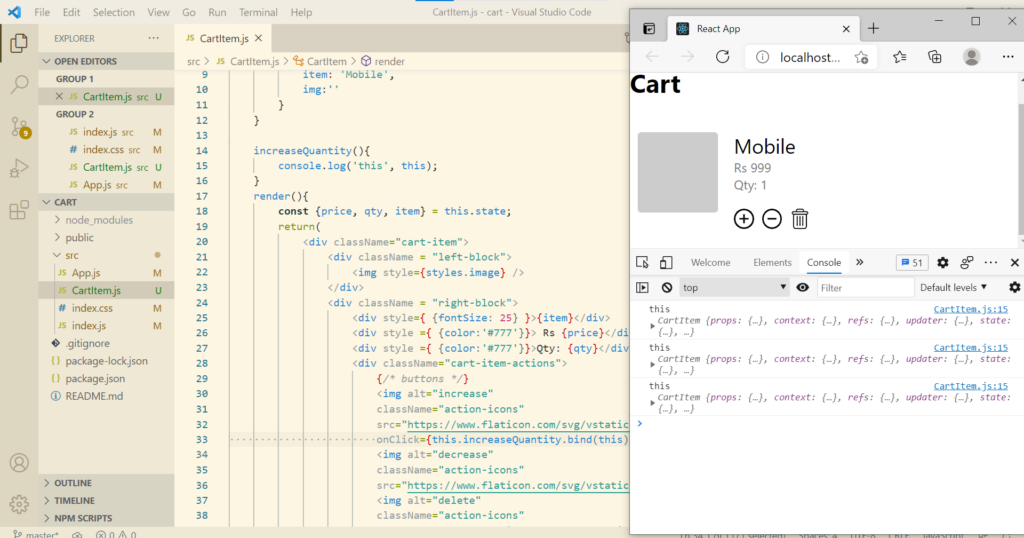
2nd Way – binding in the constructor
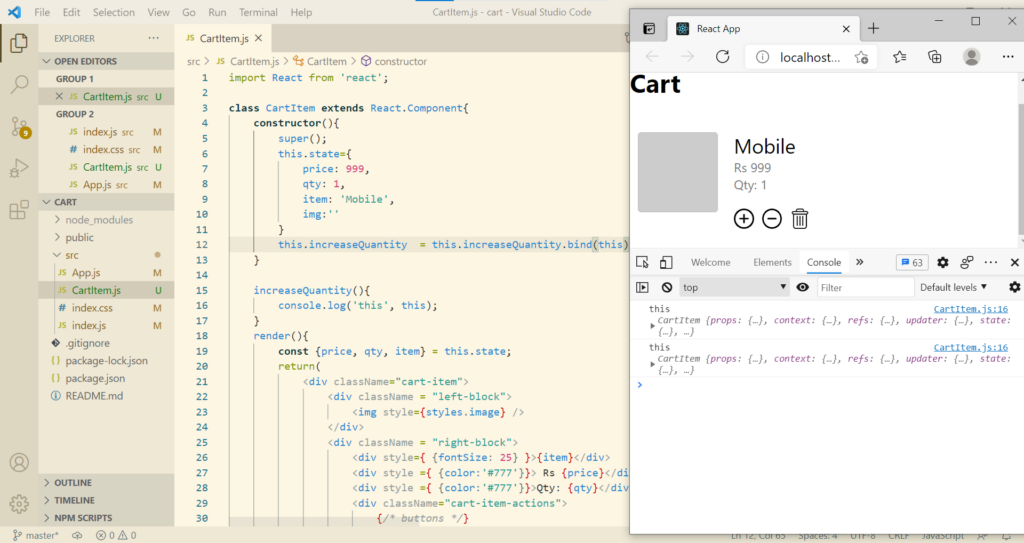
3rd way – using arrow functions – the best way
Arrow function will bind the value to the instance of the class.
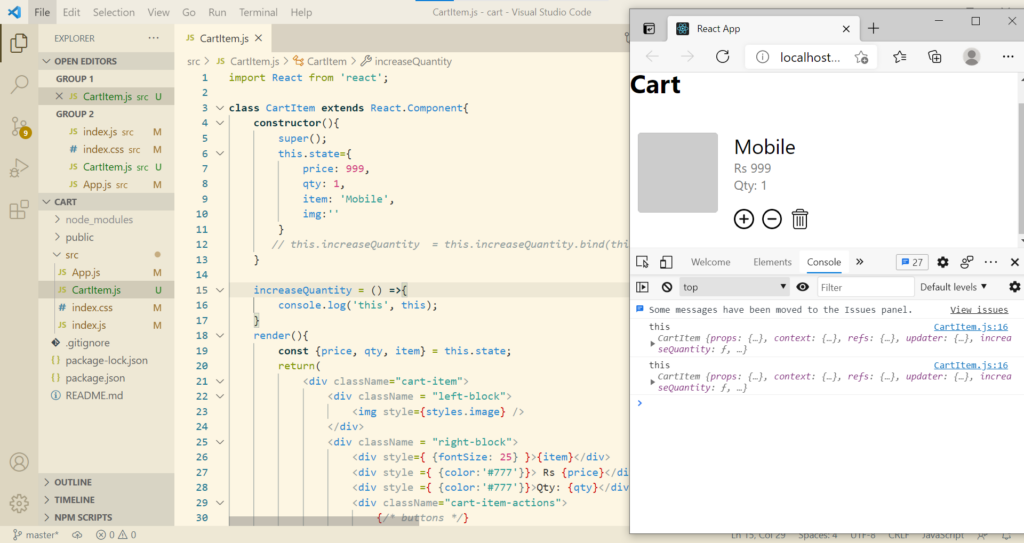