Let’s learn how we can manage and modify state. In this blog we will learn how to increase the Quantity by 1.
Let’s increase the quantity and click on add icon.
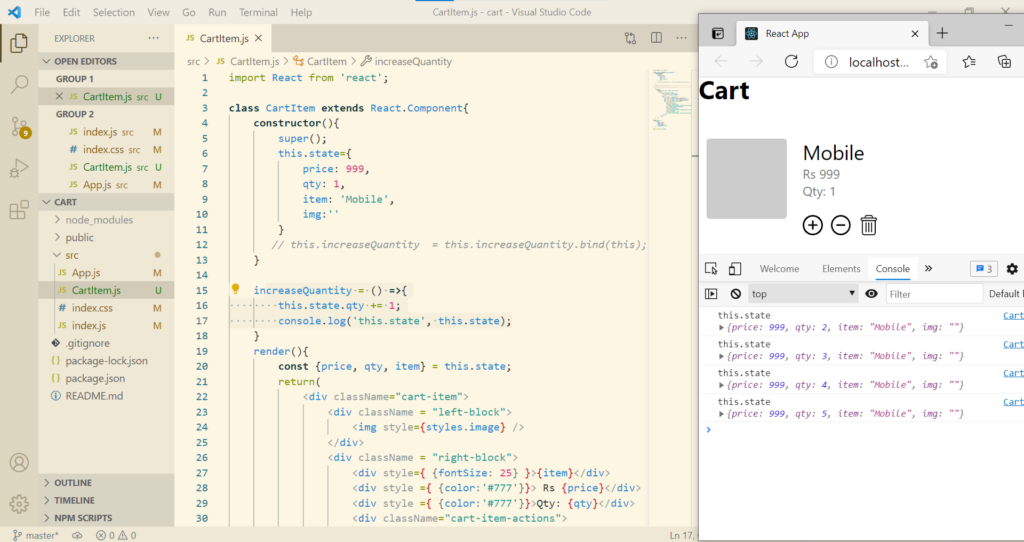
We can see that clicking on the Add icon increases the quantity by 1 each time. However the latest quantity is not being displayed. This is because the quantity of the object is increasing and react is unaware that it needs to re-render the component. So as to tackle this react gives us a function called setState() which is inherited from React.Component.
1st way
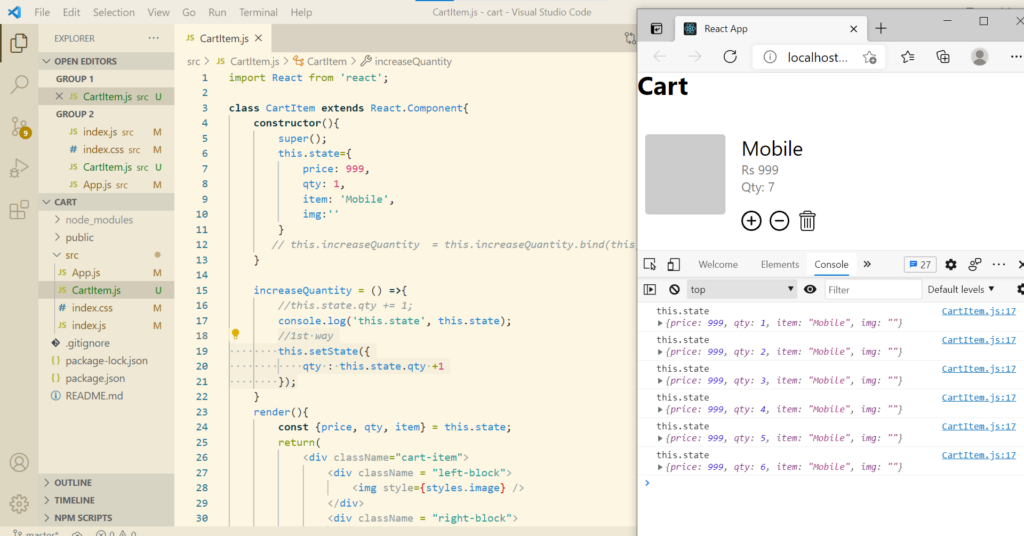
Behind the scenes React will pick up the setState object and update it in our original state object. This is done using Shallow Merging, which means React will only update the qty property and it will not impact any other property apart from the qty property.
2nd Way
Here we can make use of the callback so as to achieve the desired goal.
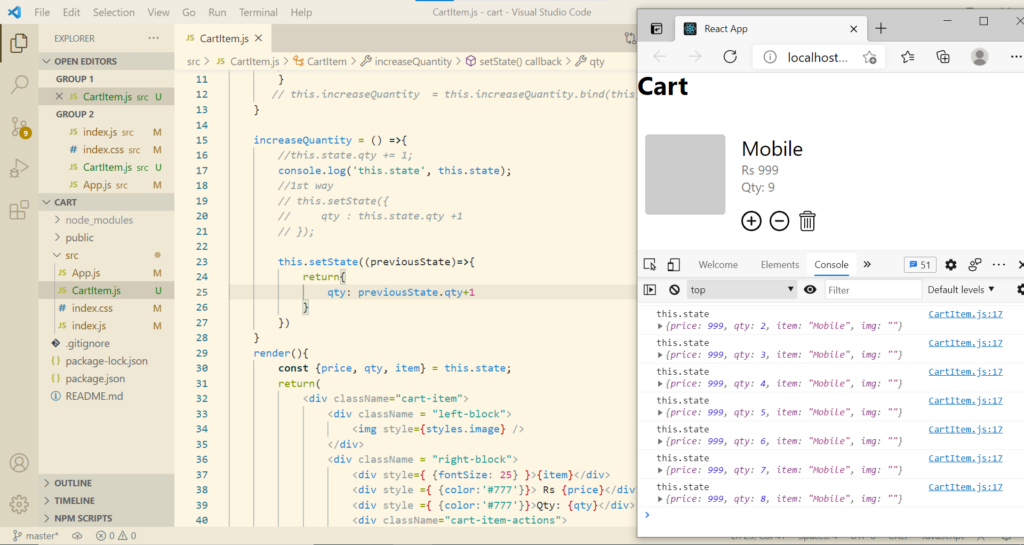
State in Depth – I
Let’s write the code for the decrease quantity.
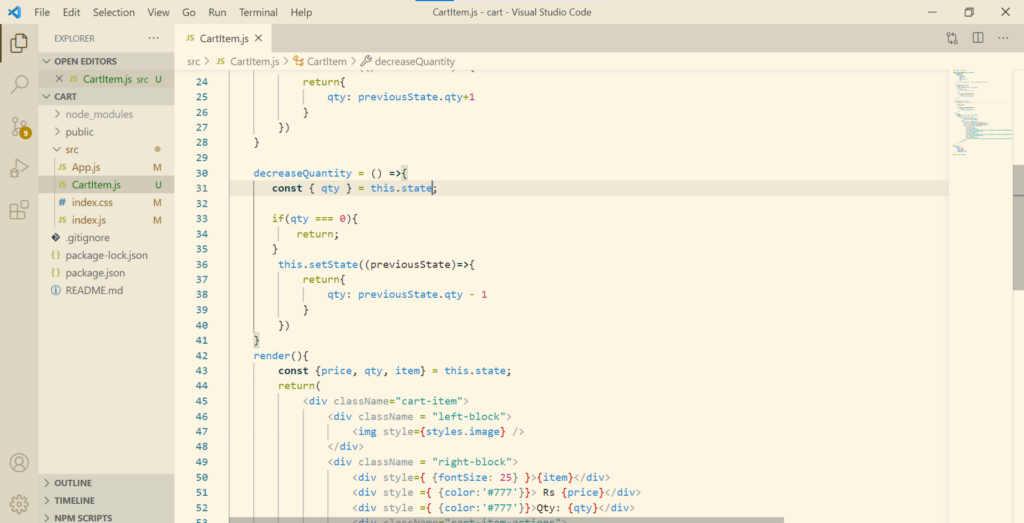
Let’s say if we want to increase the quantity by 3. We can directly do that by adding 3 in setState code. However, what if we write the setState function 3 times as below , Let’s use the 1st Way to write setState() function here?
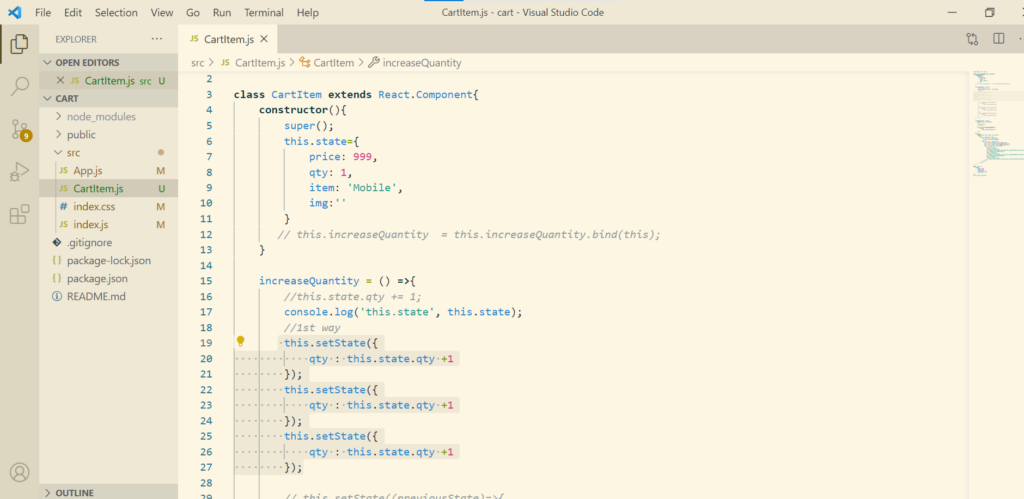
React will increase the quantity by just 1 only, this is due to a concept called Batching. In batching a shallow merge is being done on the 3 setState functions and the last setState() function is picked for execution and render() function is called only once.
Now let’s try to replicate the setState() function using the 2nd way.
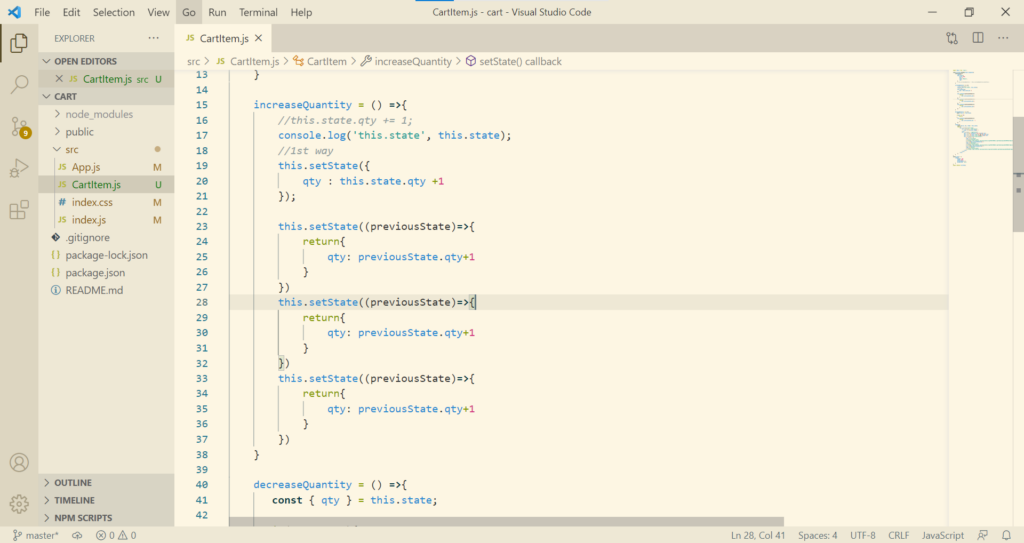
Now we see that the quantity is increasing by 3 but the render function is called only once.
This is because all the 3 callbacks are pushed to queue and and would be called one by one. While calling, the previous state would be increasing by 1.
One more thing to note here is that our setState() call is Asynchronous. What if we want to trigger a event listener after the setSTate() is completed. So as to handle this, React gives us an option to use a callback in setState() as below. We can use callback in 1st way and 2nd way as well.
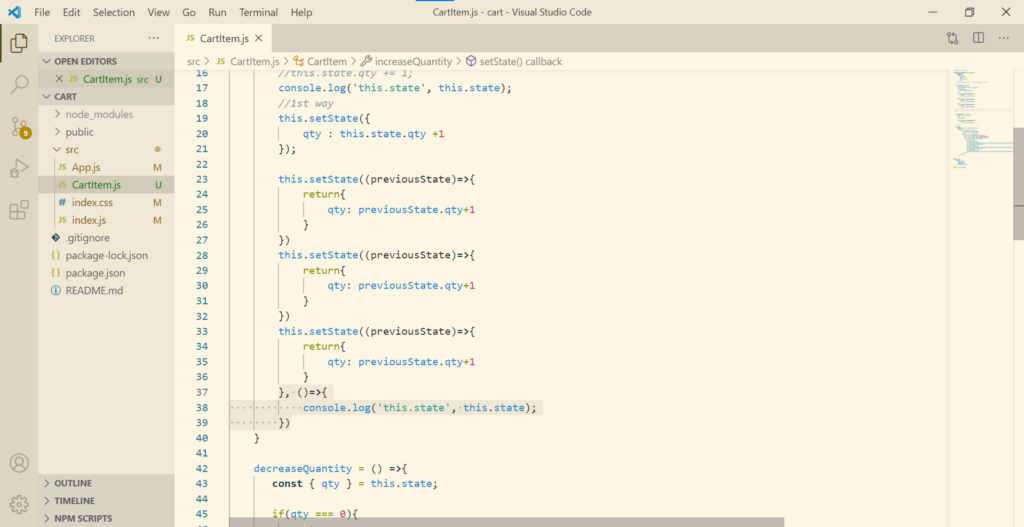
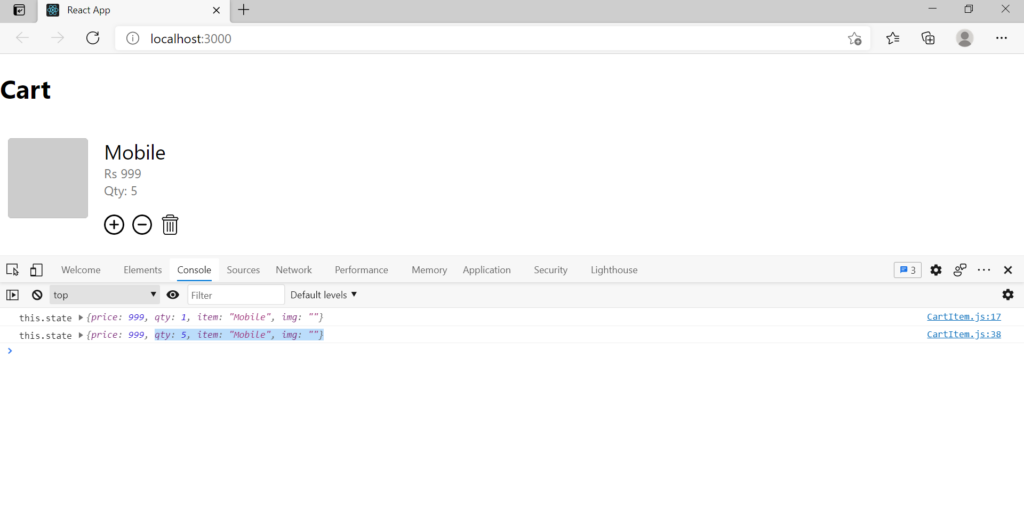
setState in Depth – II
Batching and callbacks concept is not applicable in cases of Ajax calls and Promises. In Promise and Ajax setState acts like a synchronous call.
So as to understand this, let’s create a function and inside that function let’s call a promise to simulate an API call.
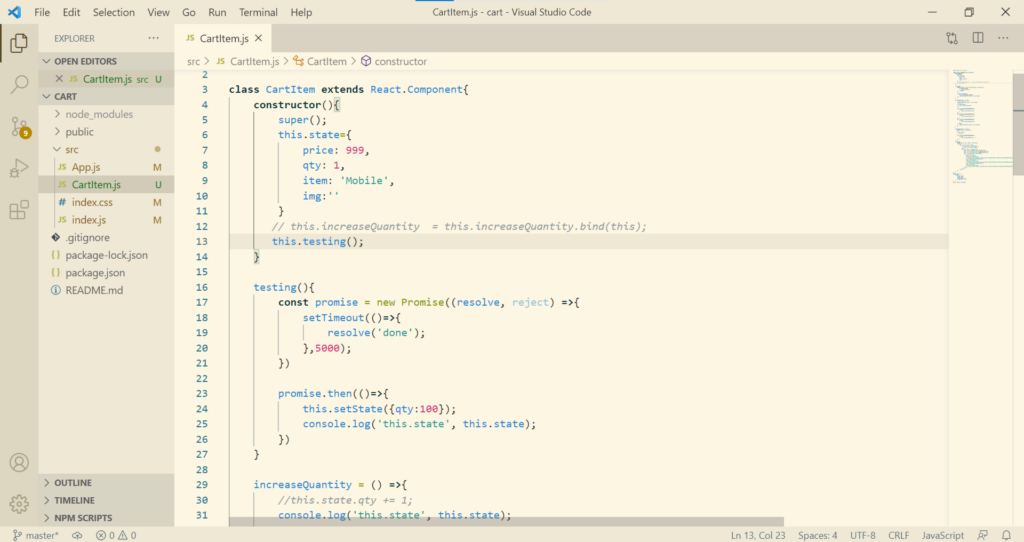
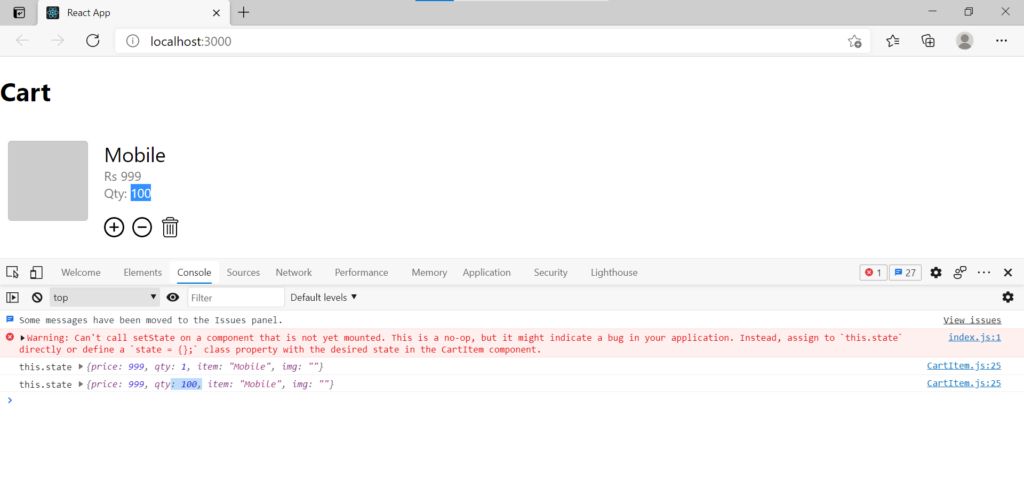
What if we call setState function 3 times as we did previously
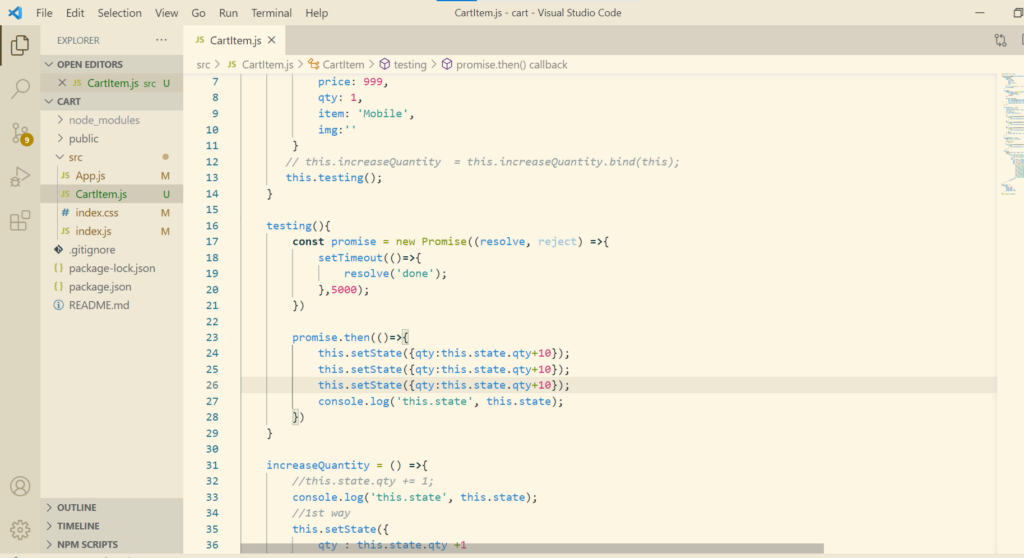
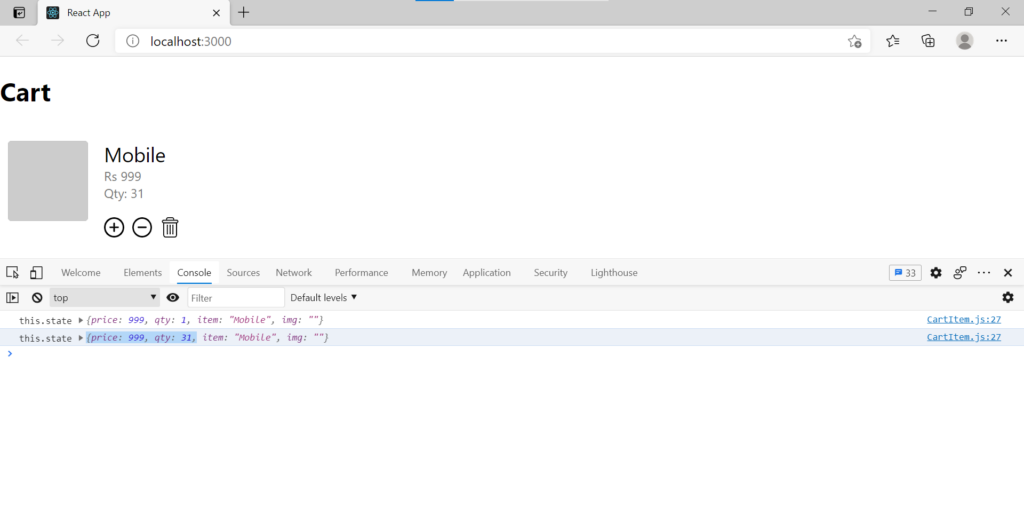
The render function is called 3 times and no batching has taken place here. Though it is a gap which would be addressed in the upcoming version of React.