Creating Cart Component
In this blog let’s create a cart component. Let’s create a cart list as Cart.js.
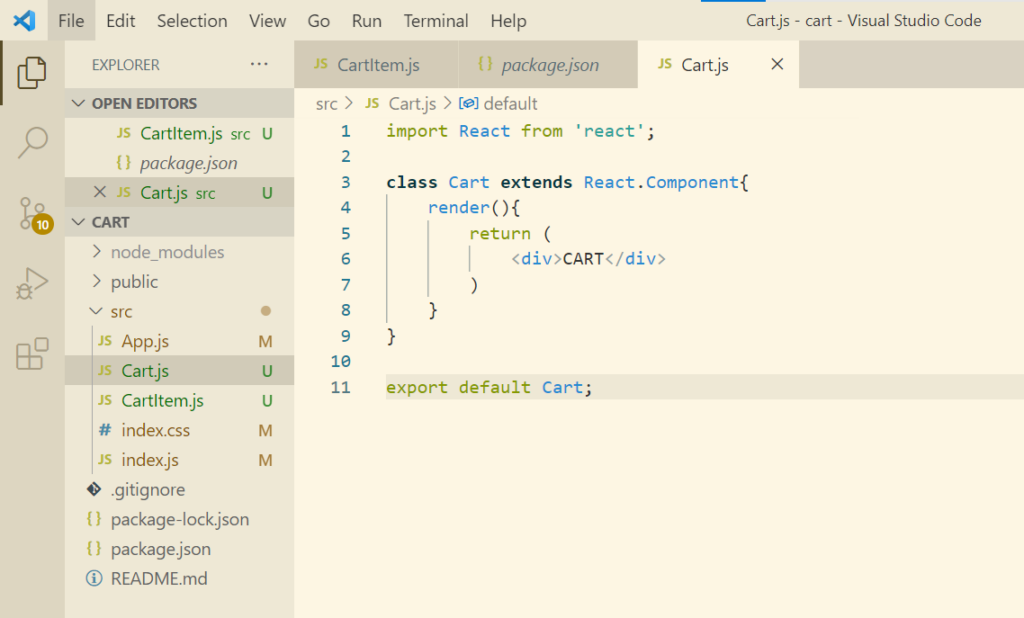
Import Cart.js in App.js
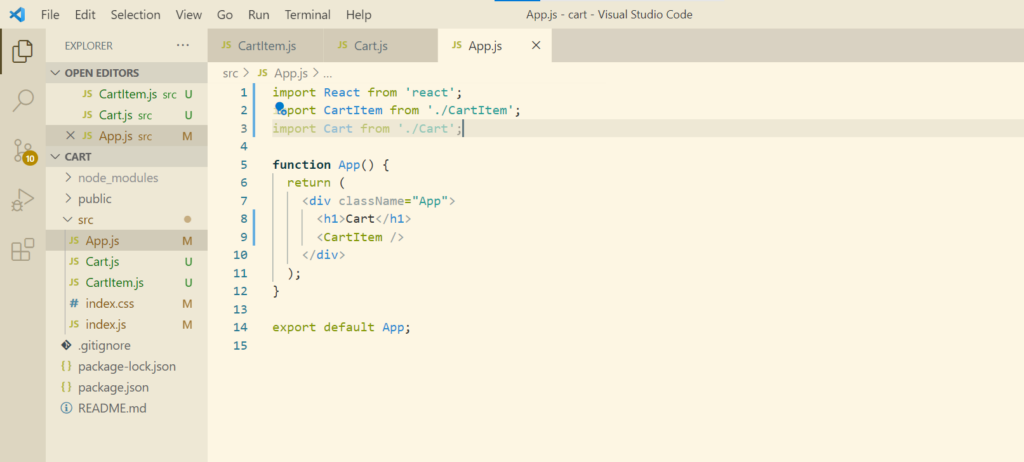
Instead of CartItem to be rendered inside the App.js, we need to render the Cart as CartItem would be inside the Cart
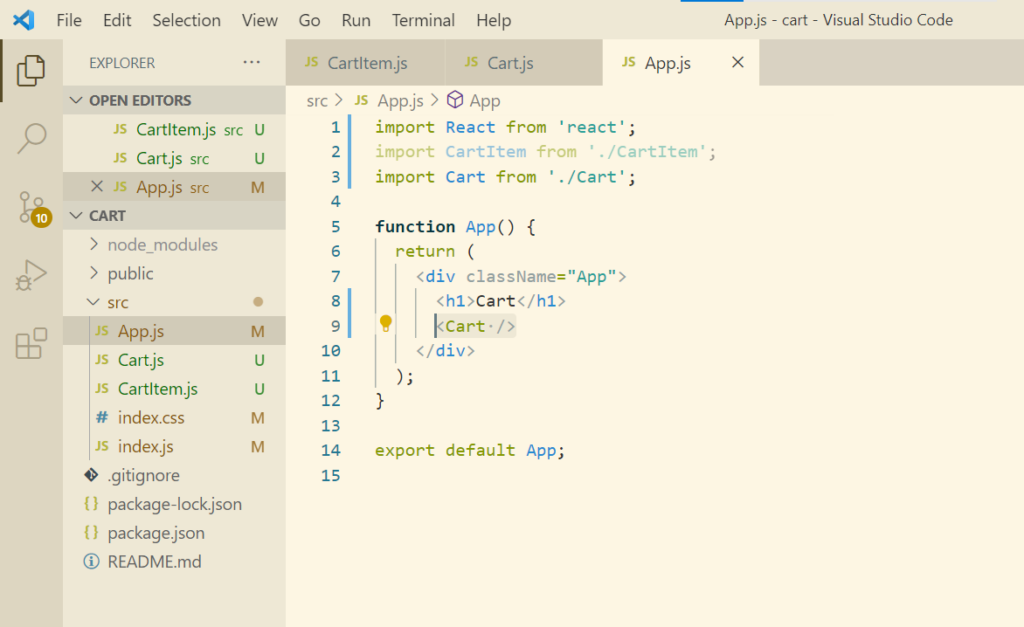
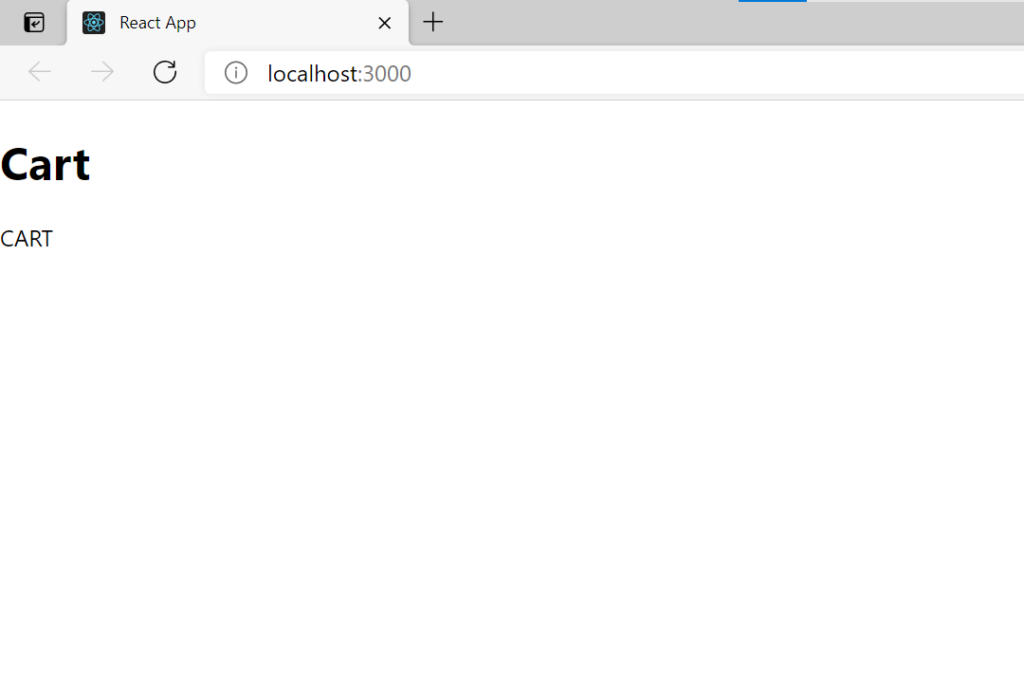
Now let’s import the CartItem inside Cart.js
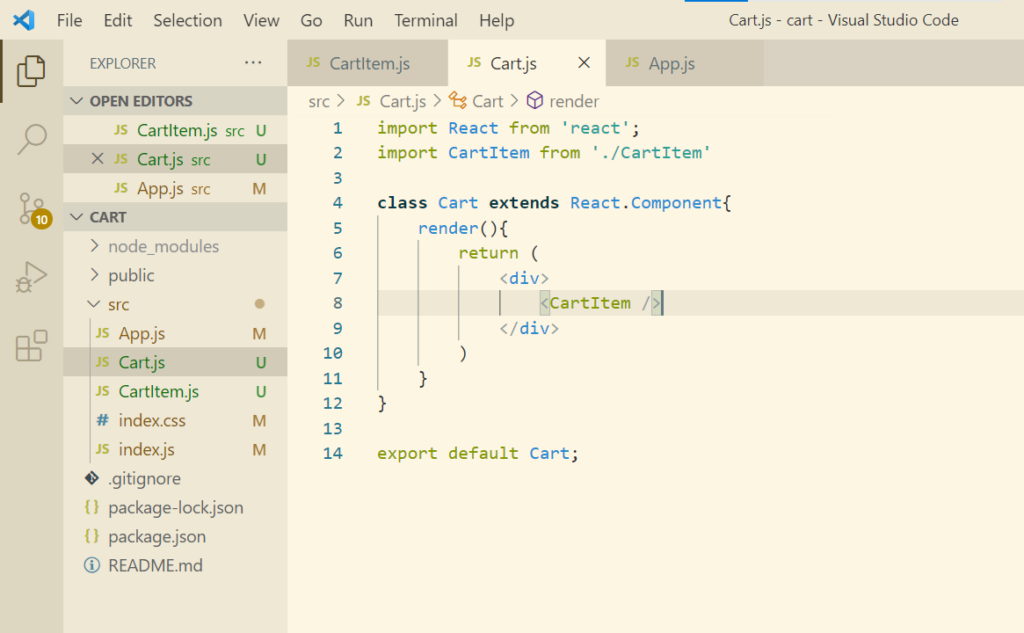
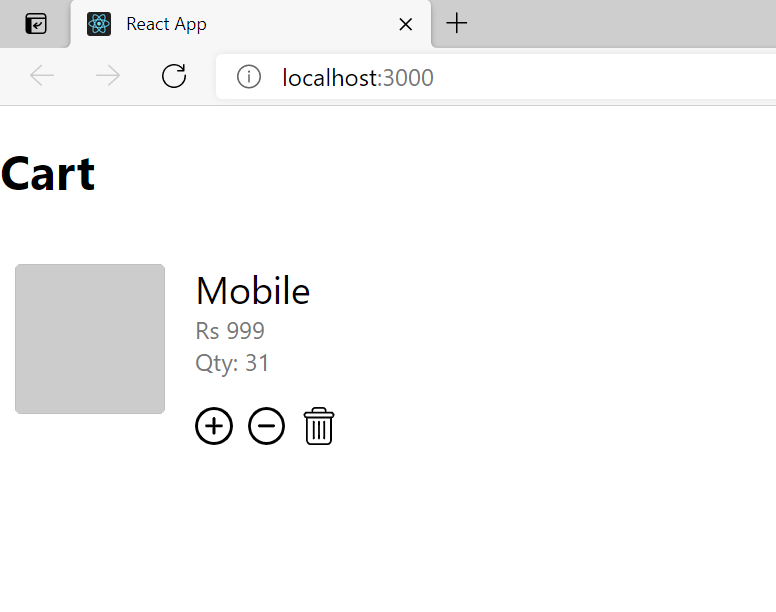
Let’s now add more Cart Items.
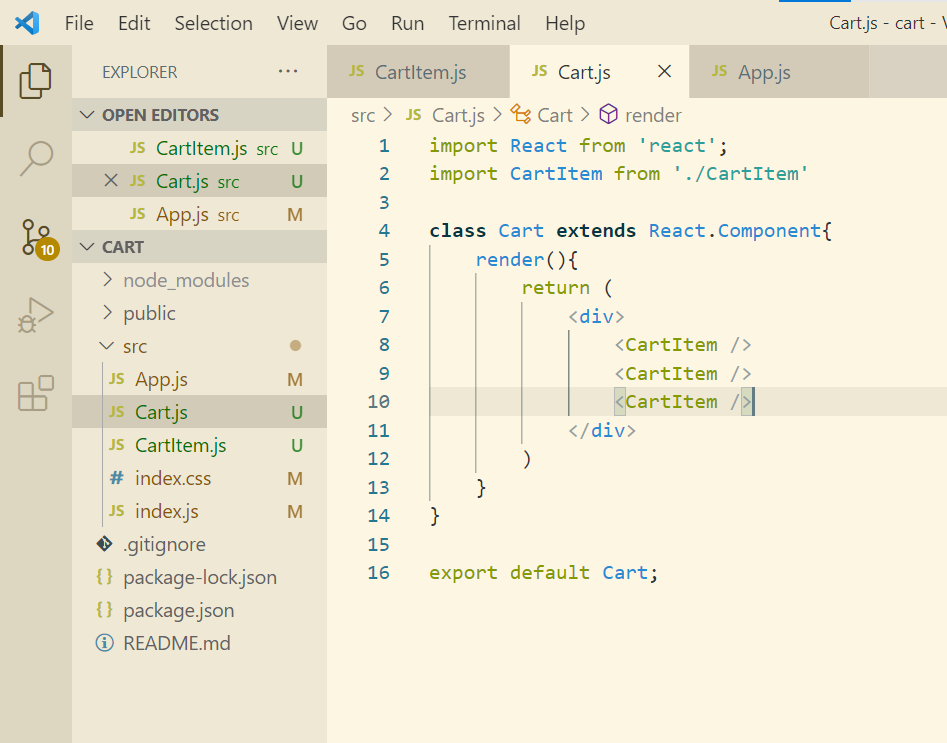
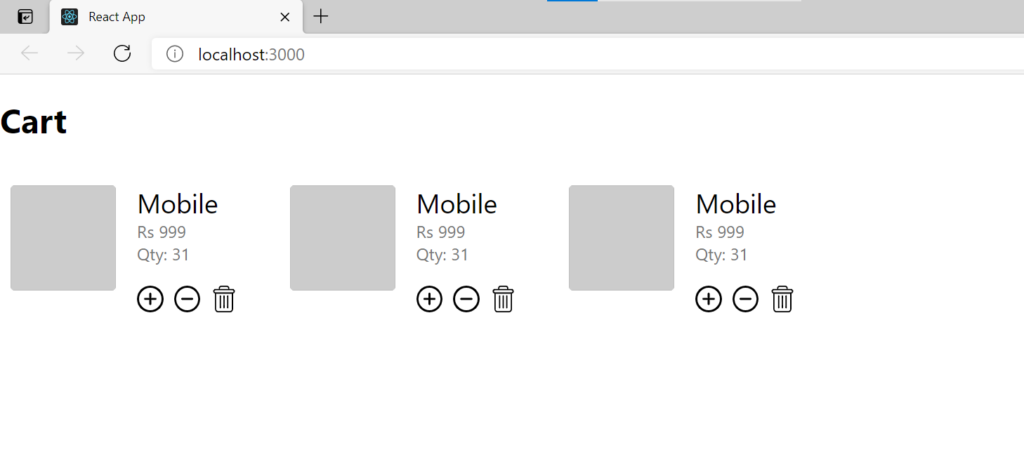
Let’s remove the heading and set some styling to this cart.
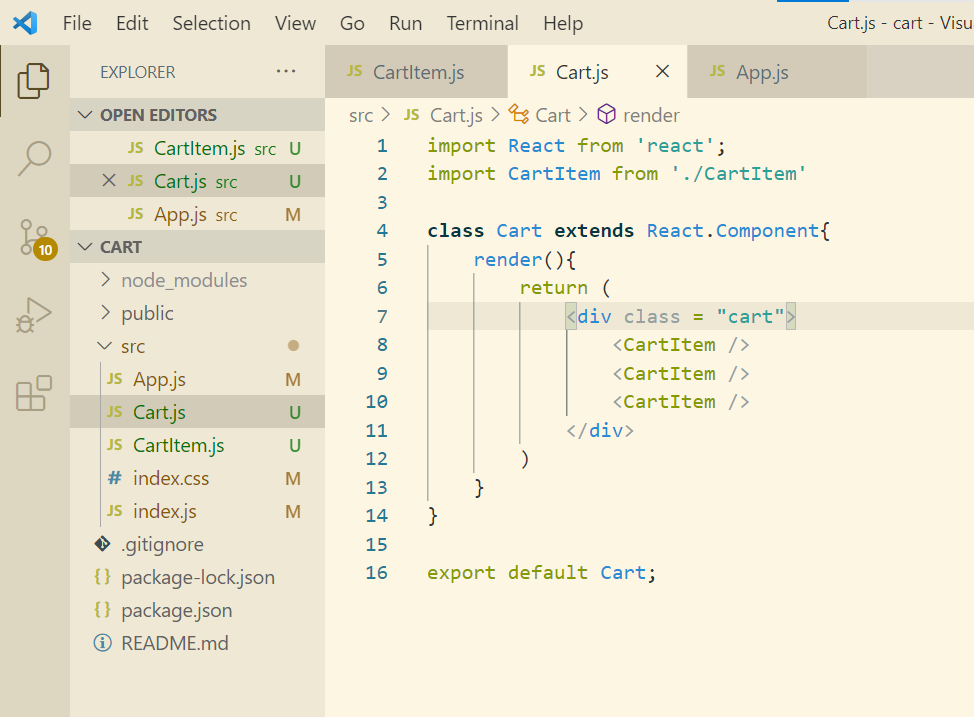
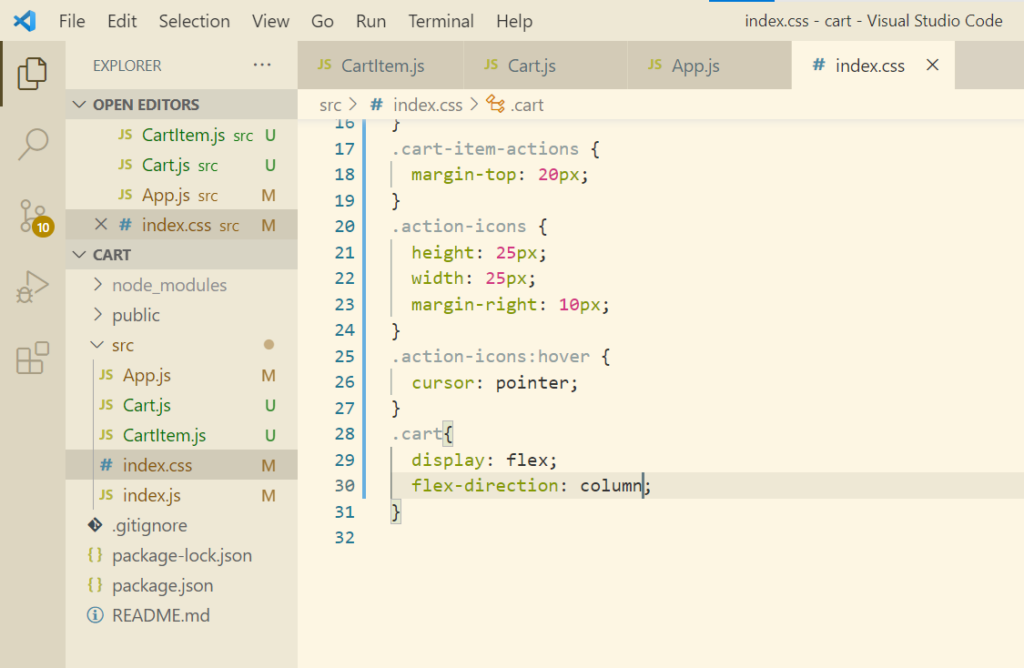
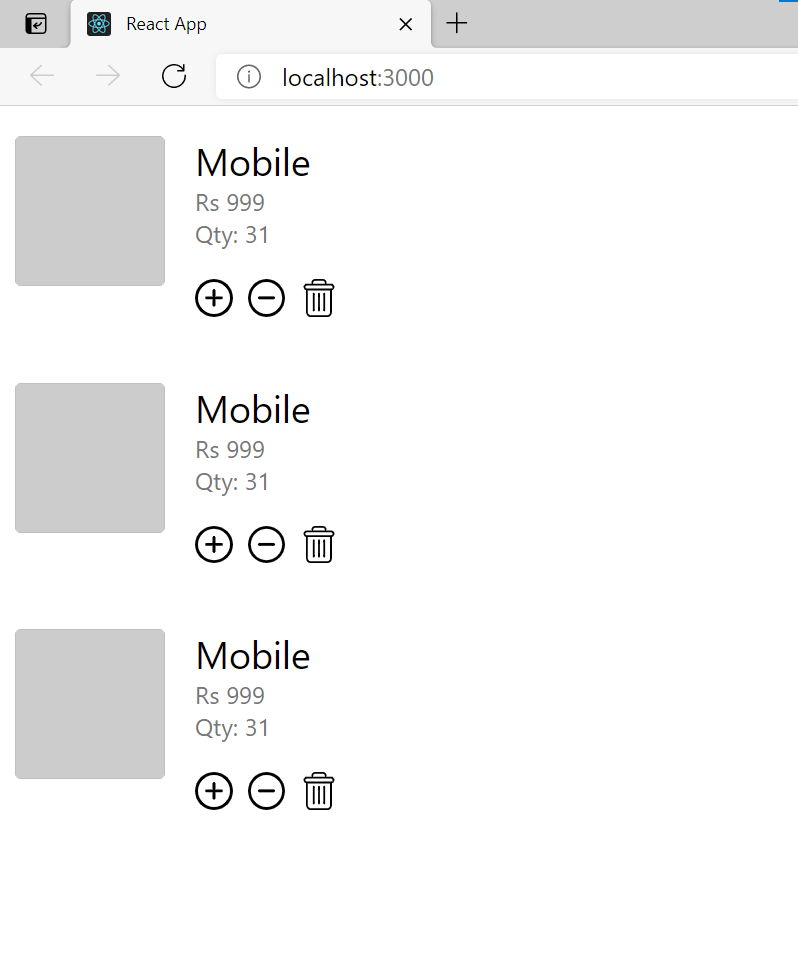
As of now we have Hard coded the CartItem. Going ahead we will make the CartItem dynamic.
Rendering a List
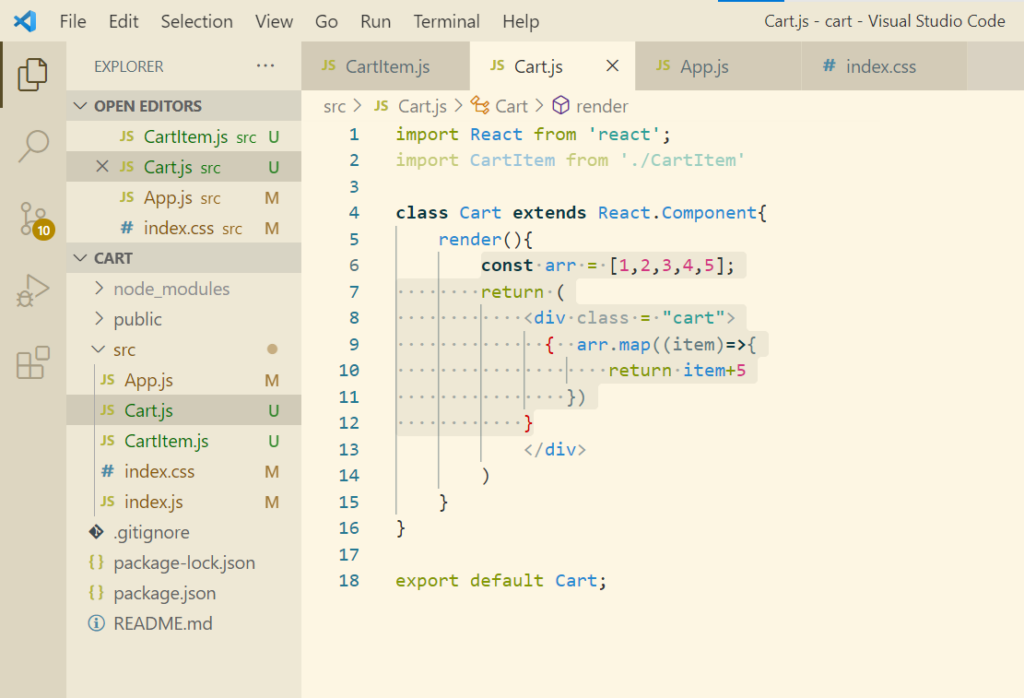
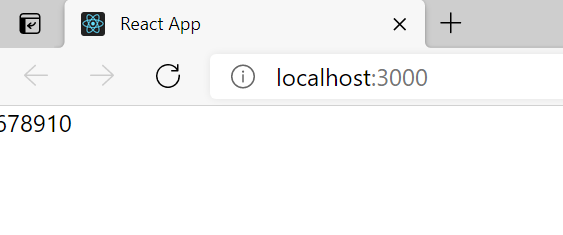
Props
Previously we have seen how to render a list in our App. It’s time to render the CartItem
Previously we have seen that the 3 cart items were same(mobile). This is because we have set local state of the cartItem. Now I want that different data should be displayed in the cart Item. This is done using Props. Props we can consider as arguments.
I can pass any data from Cart to CartItem using props.
Let’s pass the props.
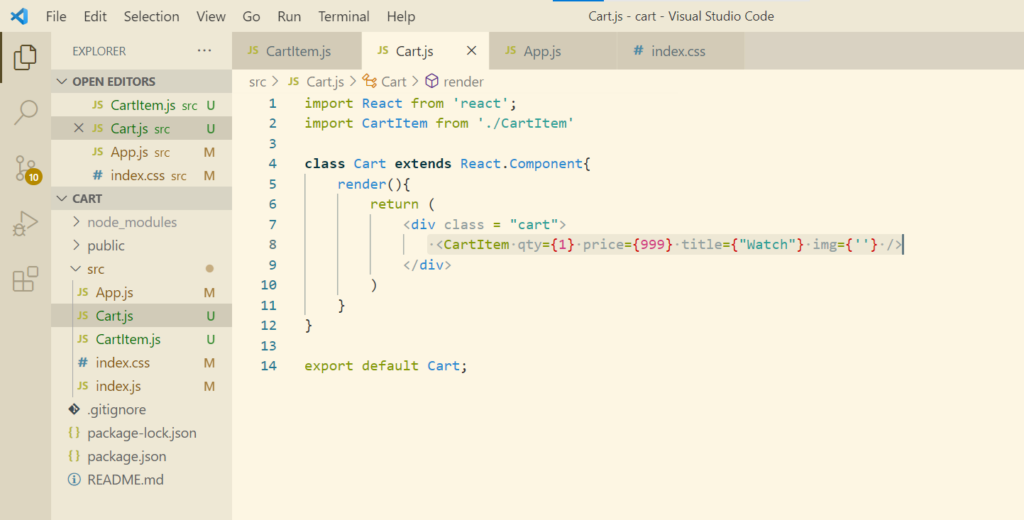
How to grab these props in my cartItem. Let’s navigate to the component.
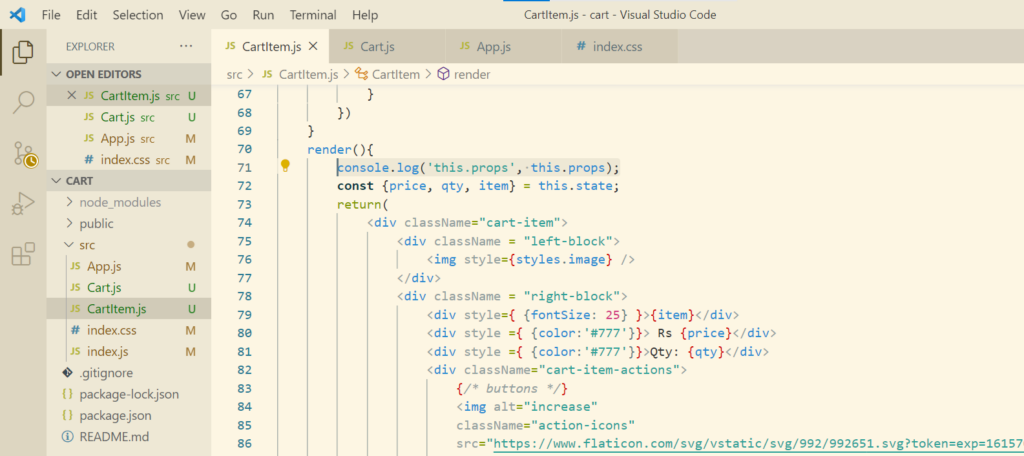
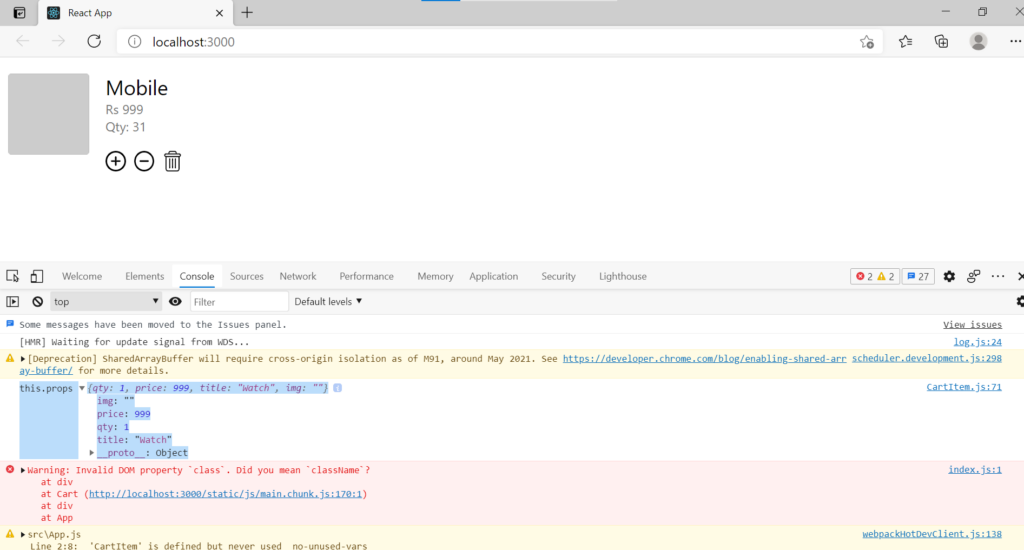
This way we can iterate over the cart component and pass it to CartItem component.
Now instead of doing this.state we can do this.props.
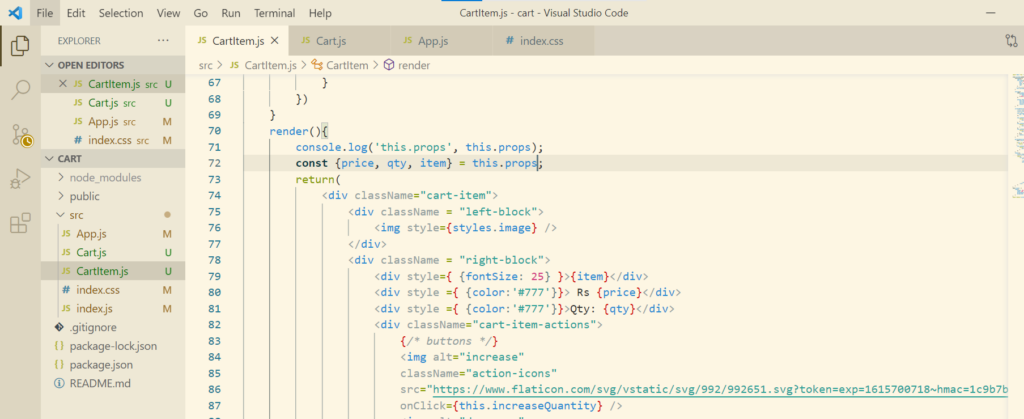
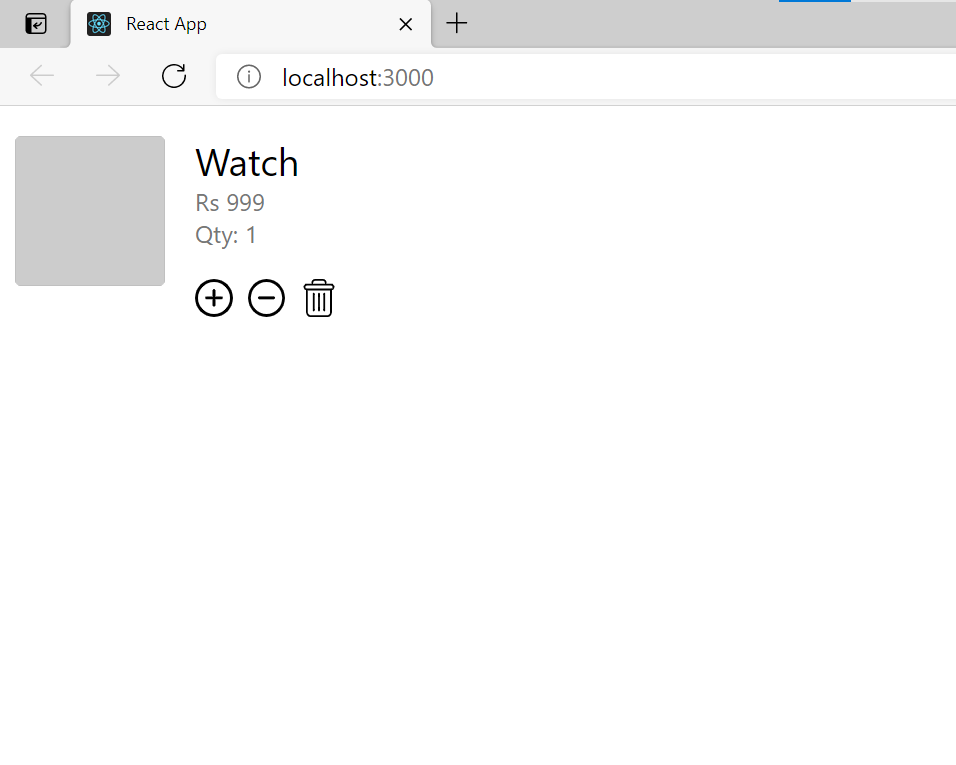
This means that I do not need a state in CartItem.js and we can use the state in our Cart.js and Cart.js will have a list of products.
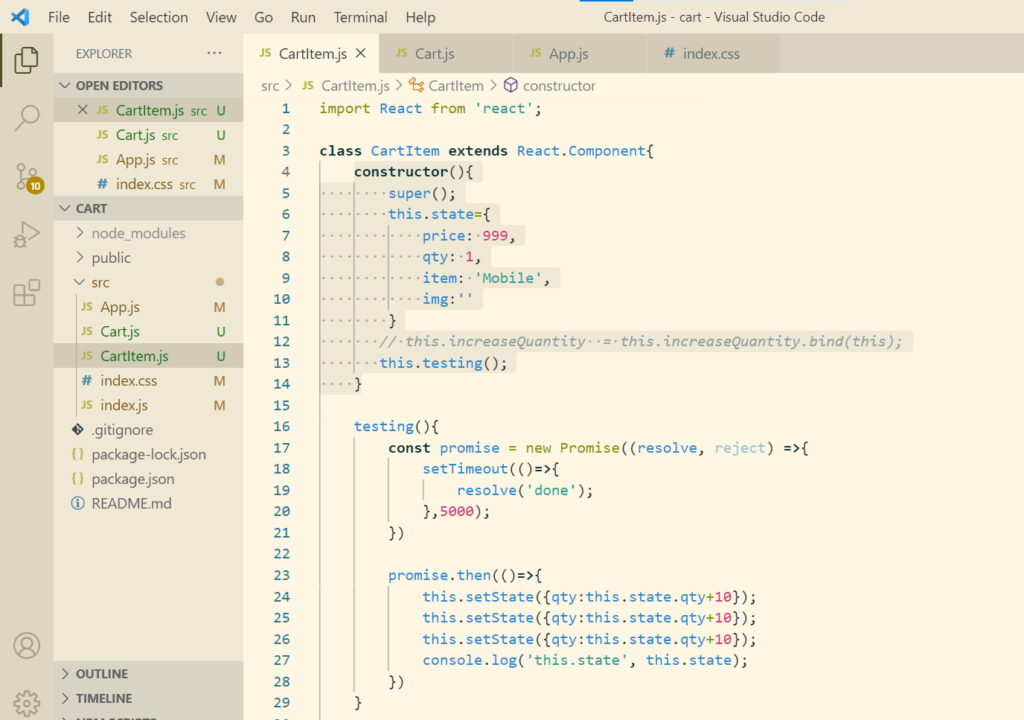
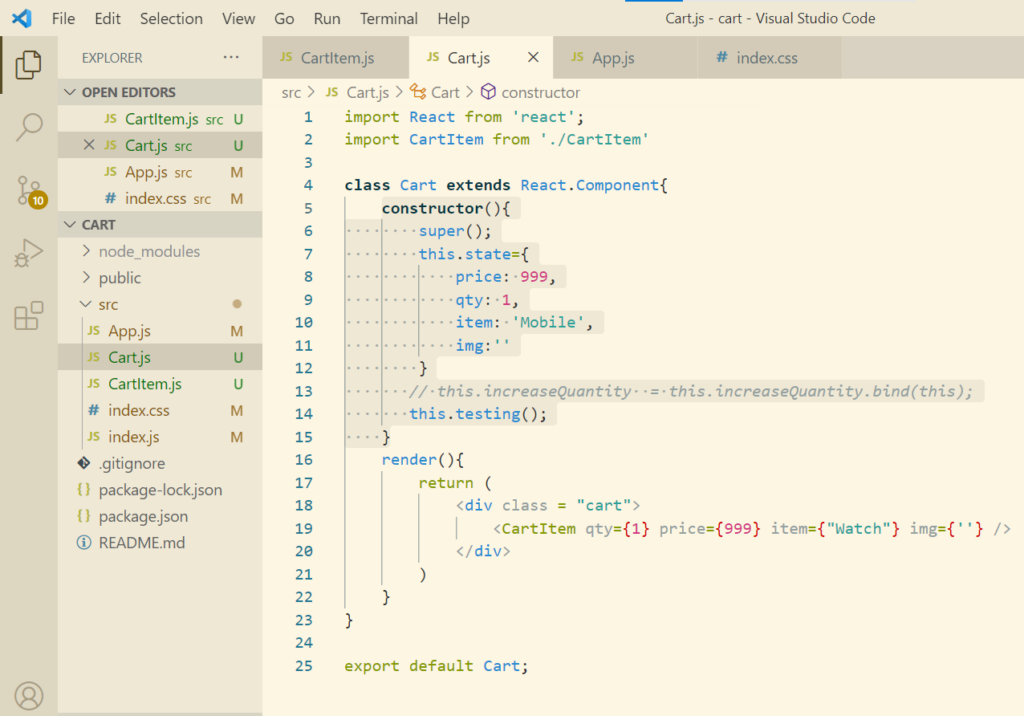
Instead of giving it just 1 product we can say that state have list of products.
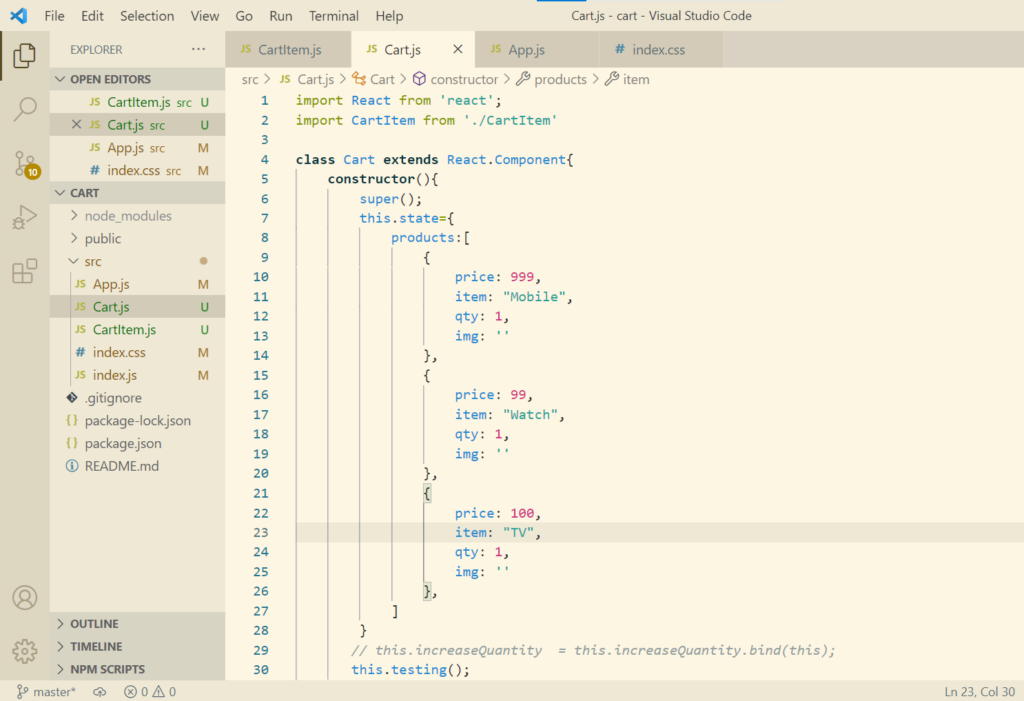
Now we have to render every item of the above product list in the cart item.
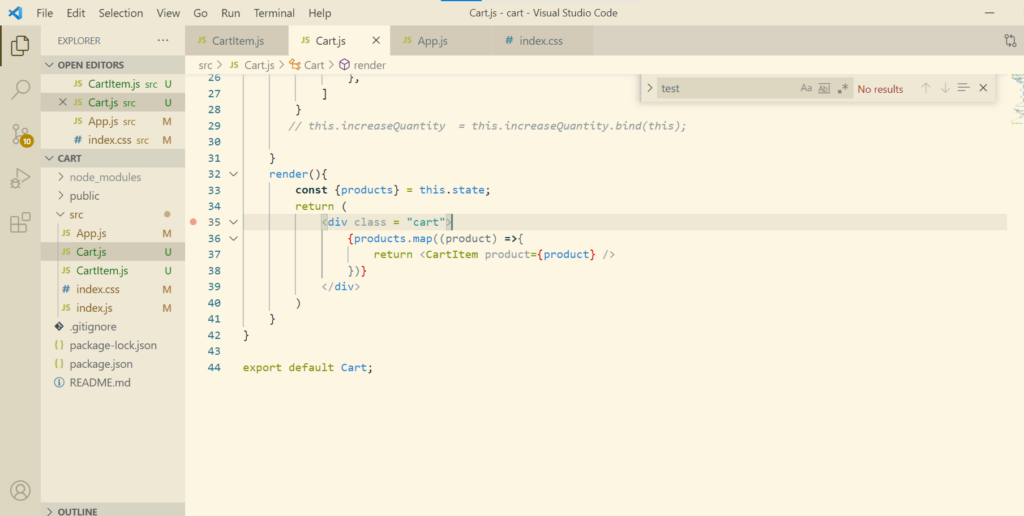
Now in our CartItem I not longer have access to properties like {price, qty, item} inside props object. Let’s change it to product.
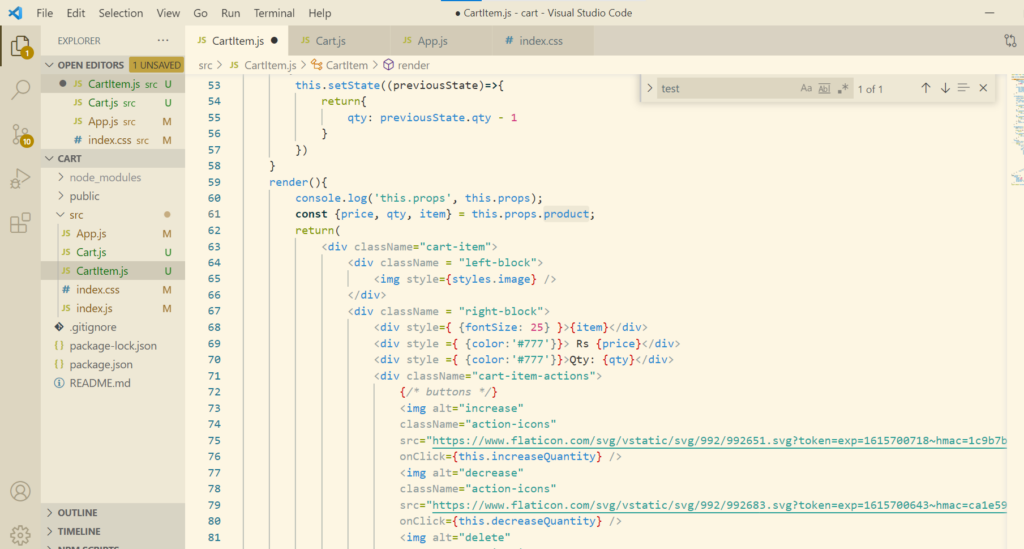
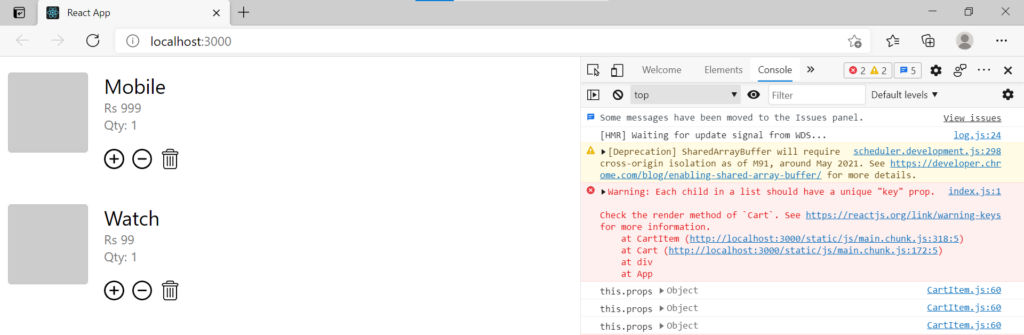
We are getting some warning as well. React wants to differentiate each cart Item which it is unable to so so right now. Let’s help React to do so.
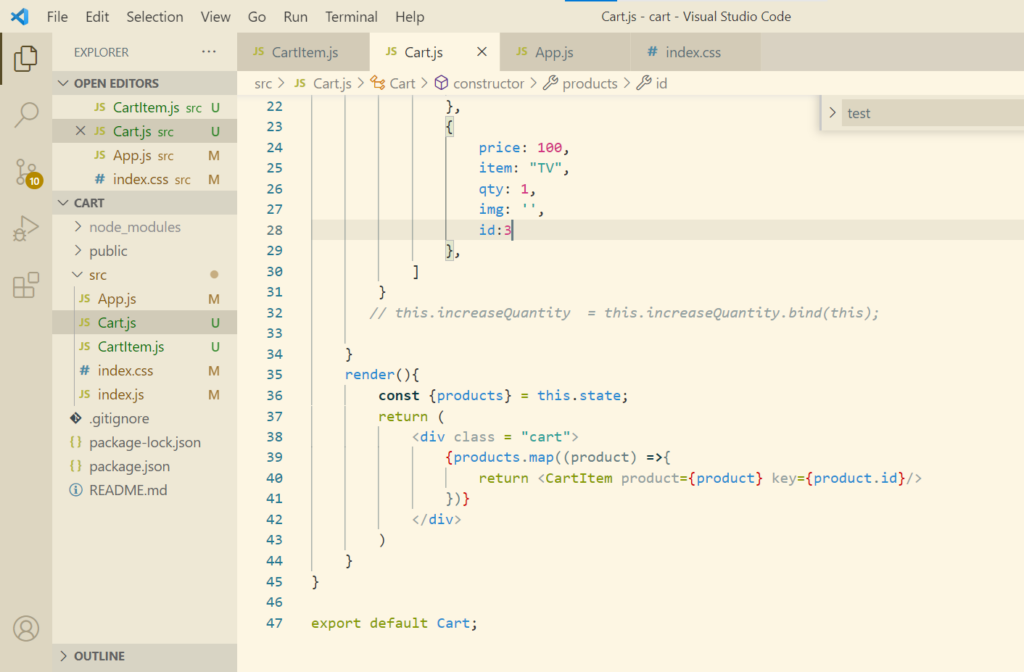
This works perfect. But the only issue here is that our Add, Subtract and delete icon are not working. This is because they are using state available inside CartItem. However there is no state present inside CartItem.js
Handling Icons Issue
Let’s remove the increase Qty and Decrease Qty code from CartItem.js as we don’t need it anymore there.
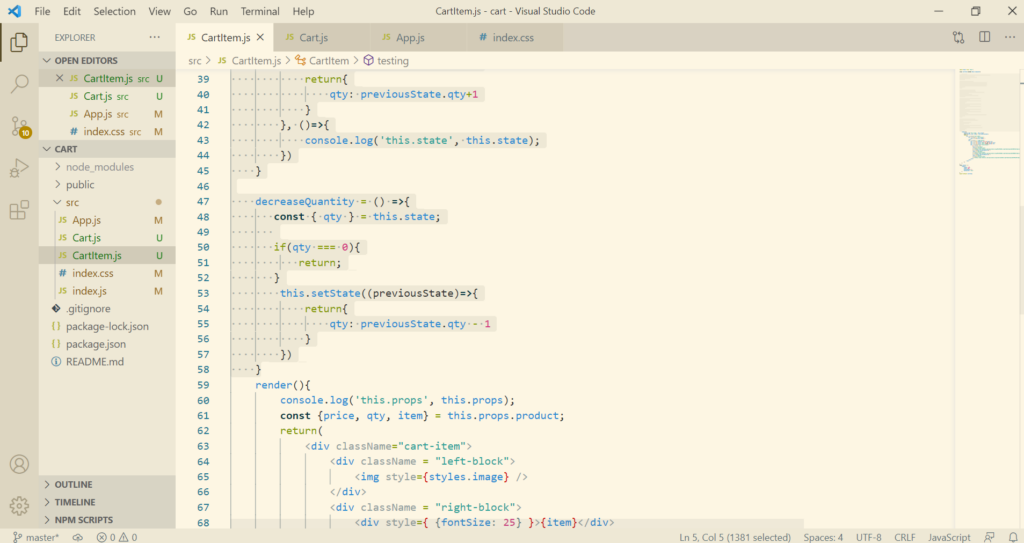
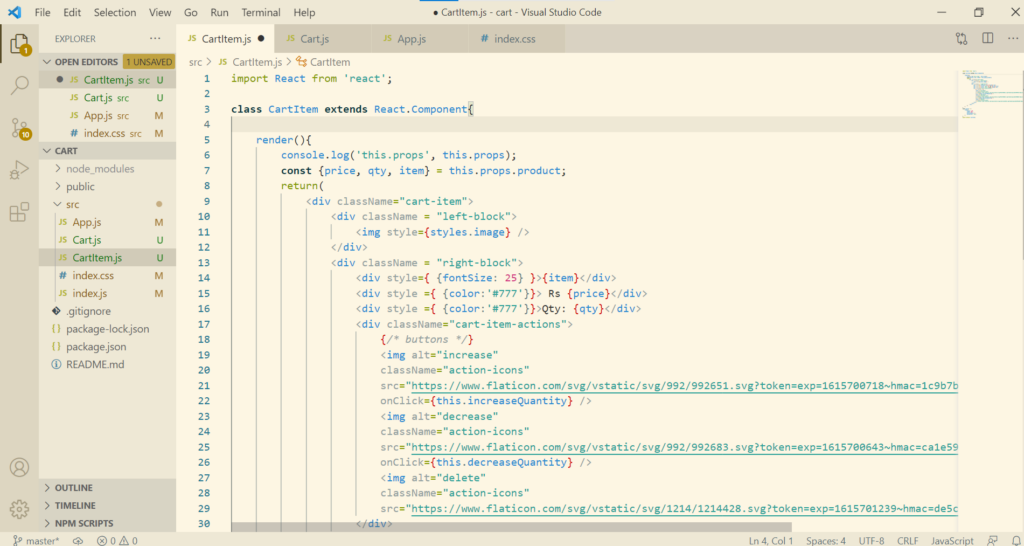
So as to resolve the issue we will make the Cart.js to pass a prop to CartIem.js, prop would be a function. Whenever the function is being called, the Cart would be notified that it has to increase the Quantity.
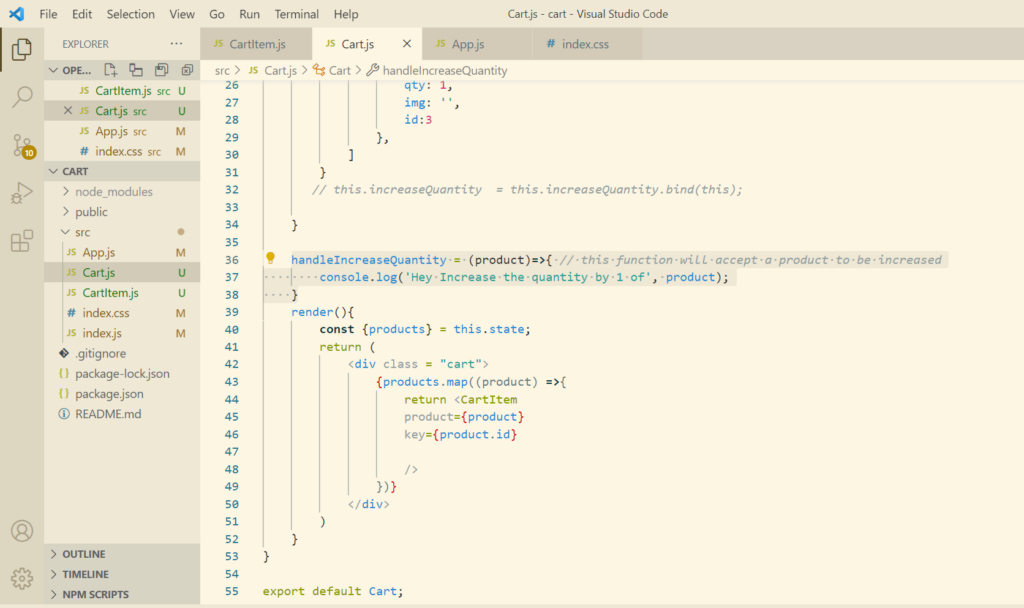
Now let’s pass this function as props to CartItem.
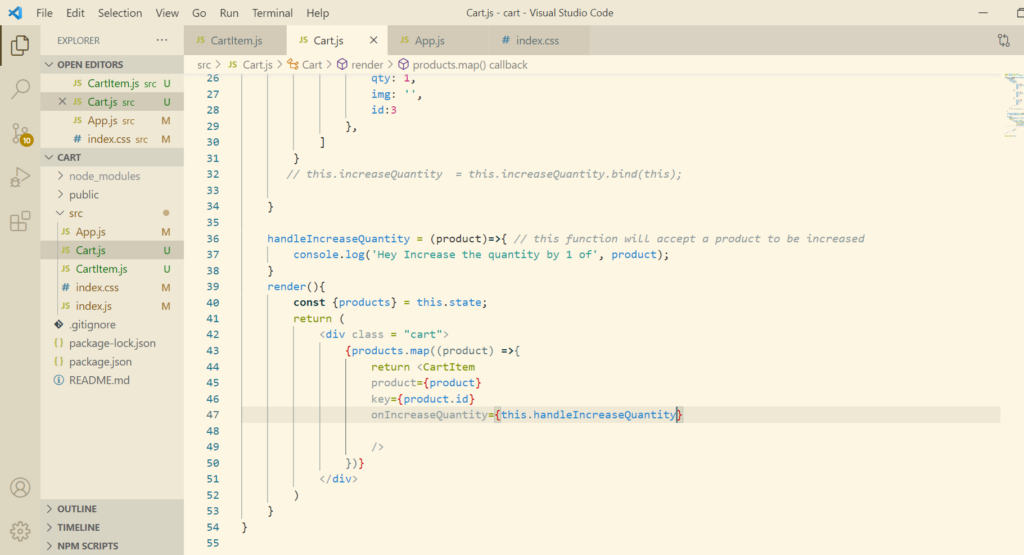
Now we need to remove the function calling which was present previously in CartItem.js {this.increaseQuantity} and call the function created above.
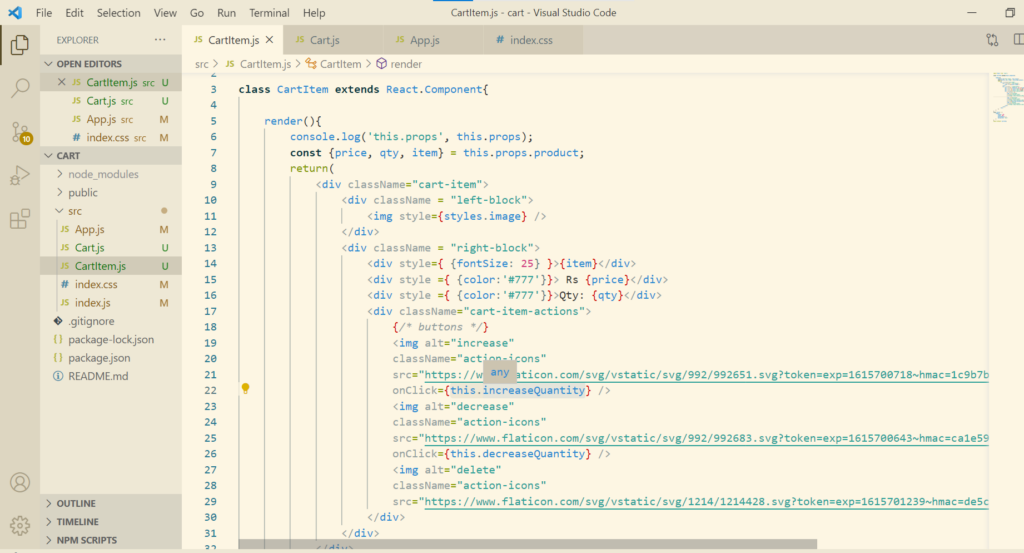
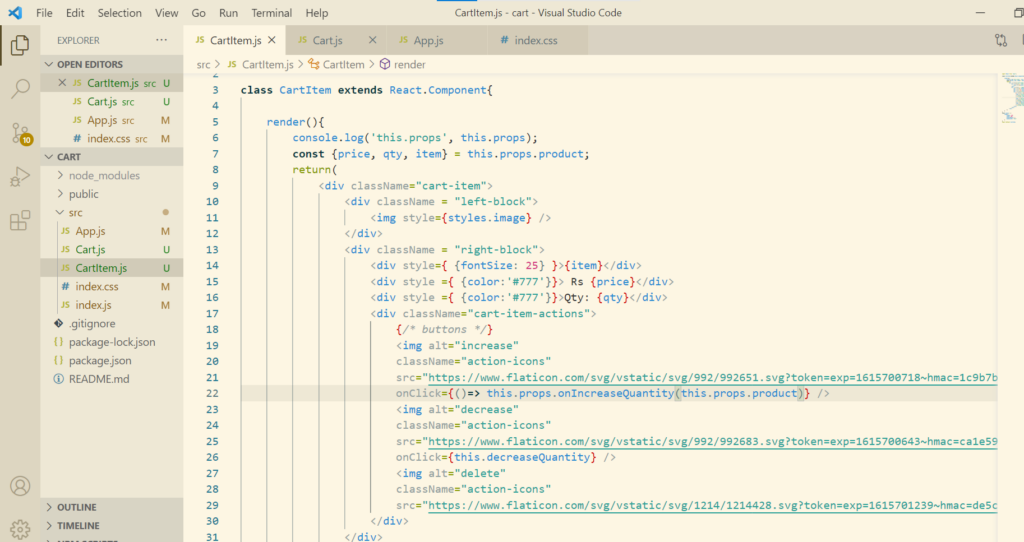
On clicking the add button the function is being called
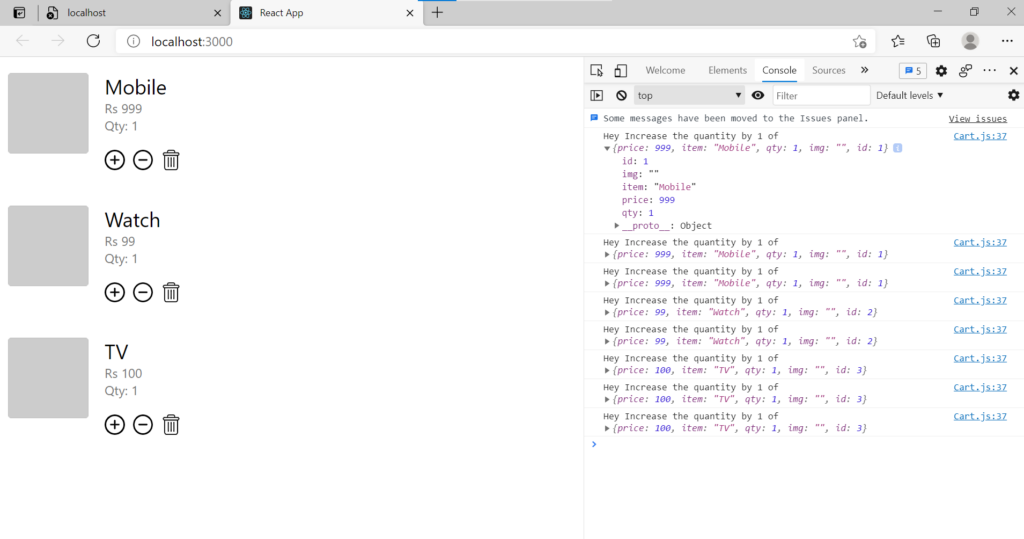
Now let’s add the +1 functionality
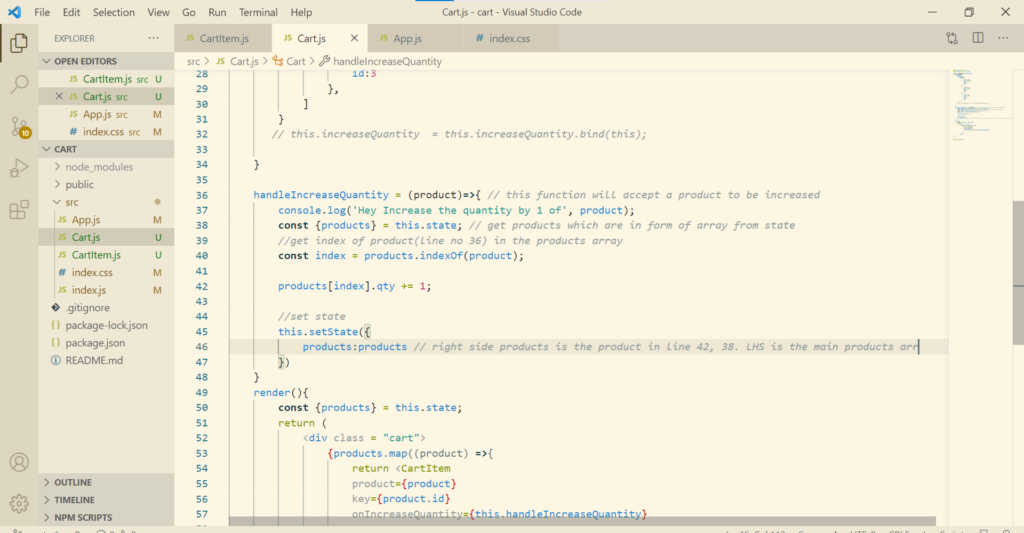
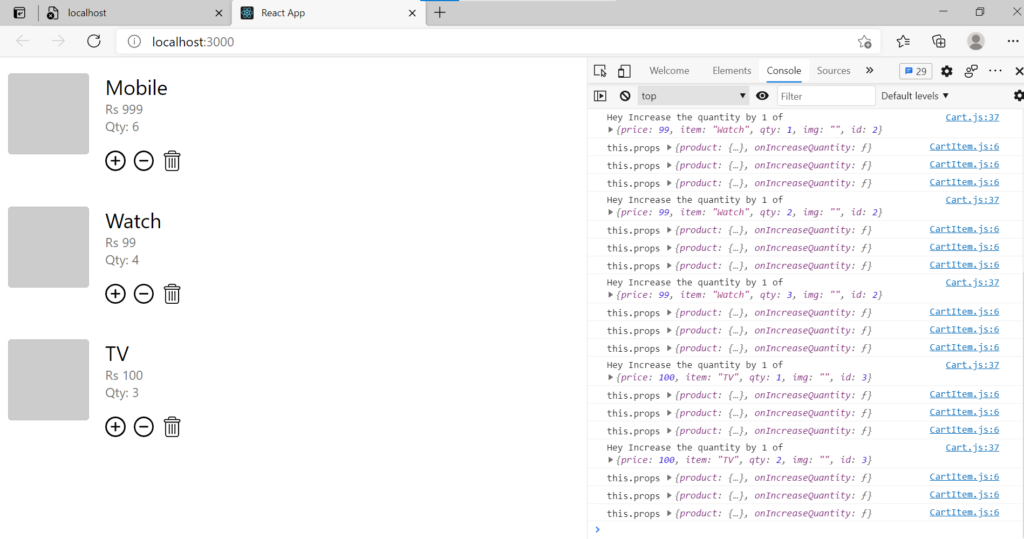
Similarly implement the functionality for decreasing the Quantity
Deleting Products From Cart
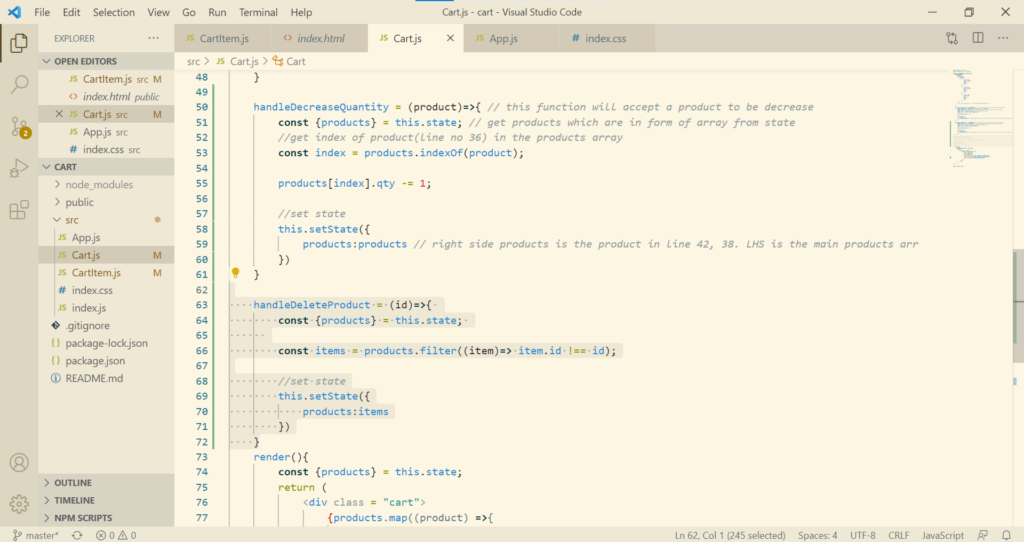
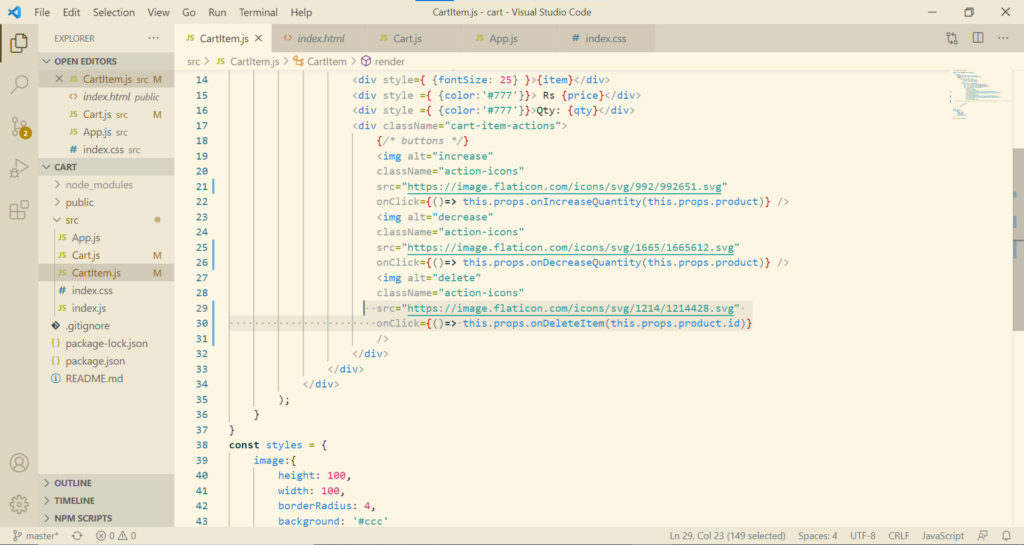
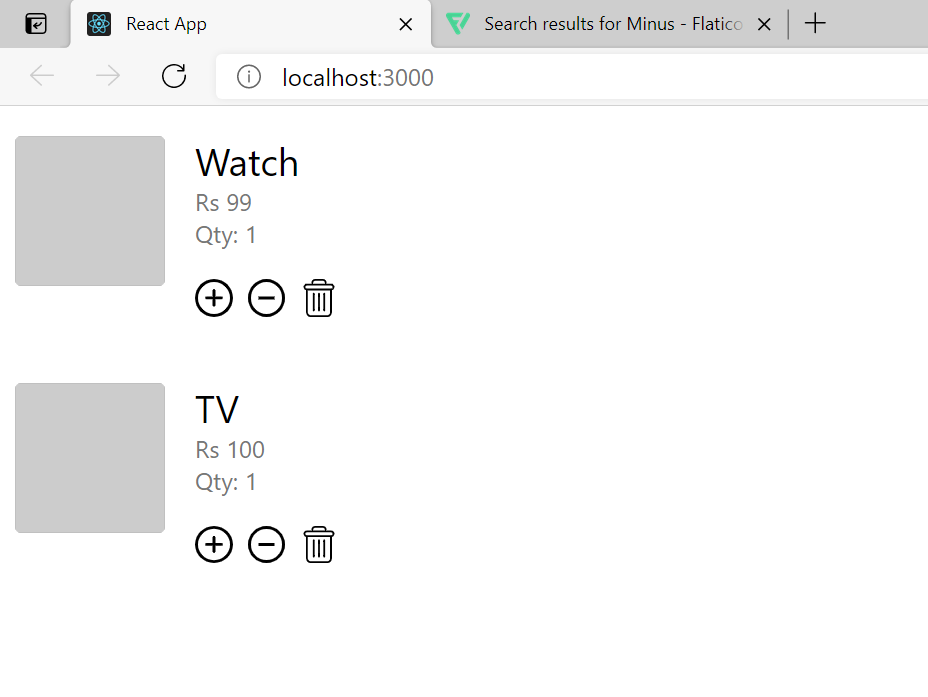
Adding the Navbar Component
Let’s copy some basic code from CartItem
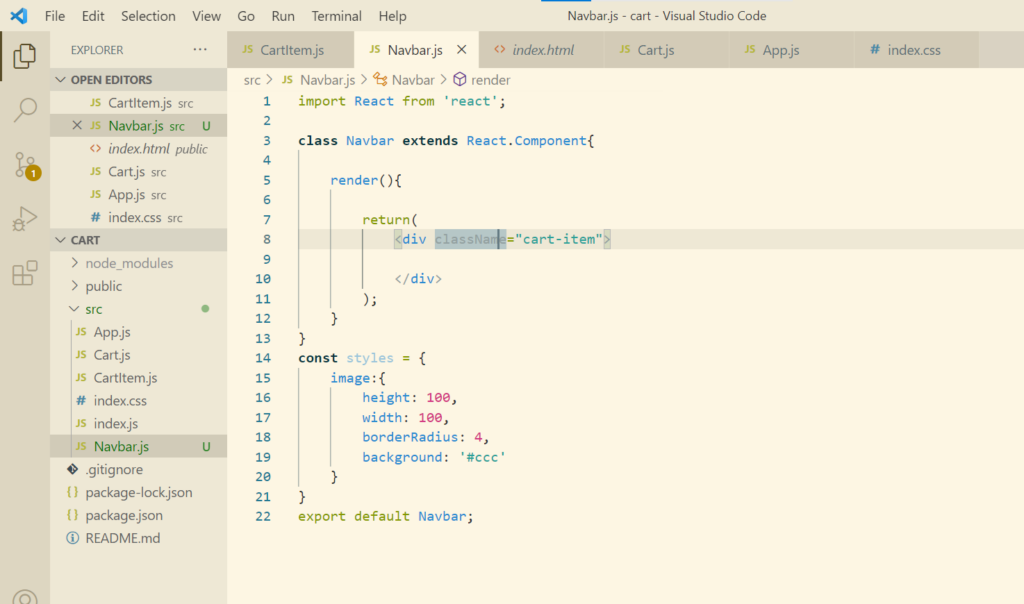
Let’s now start creating a Navbar component.
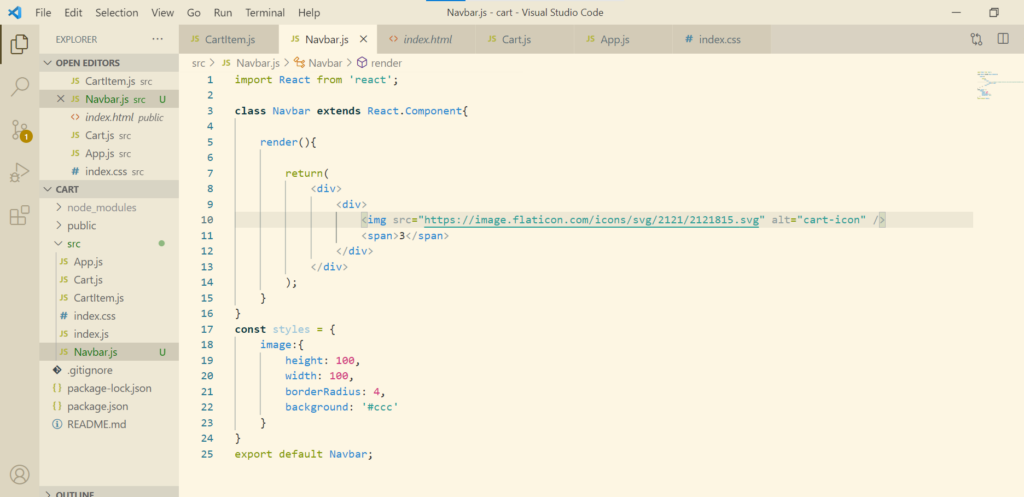
Nothing shows up on the browser as we need to import and render our Navbar component in App.js
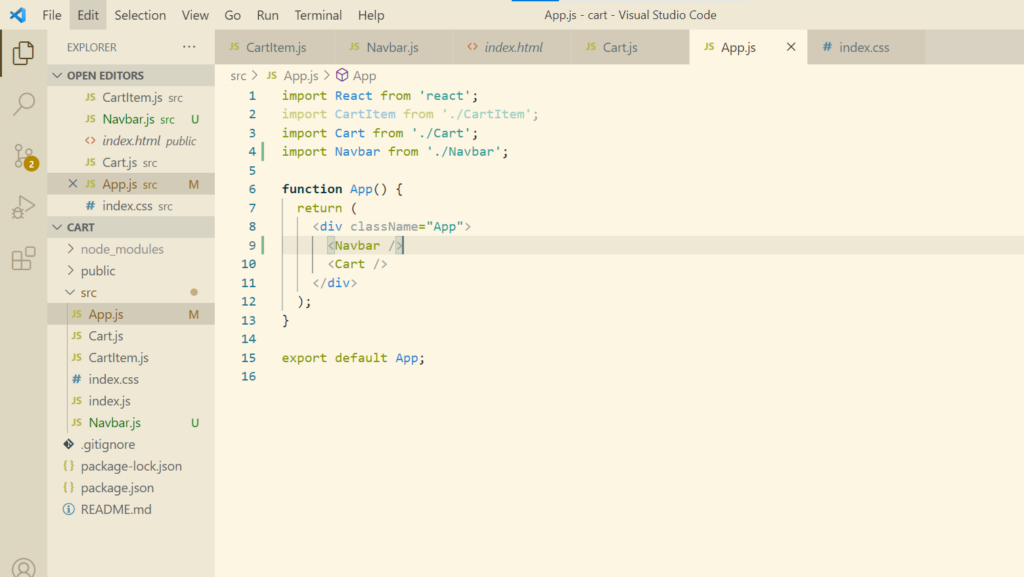
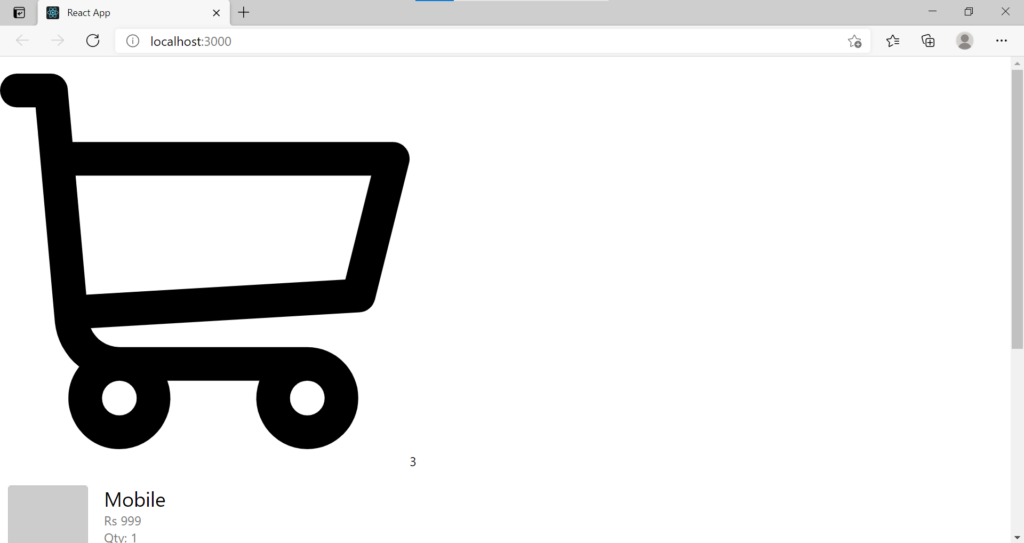
Let’s add some styles.
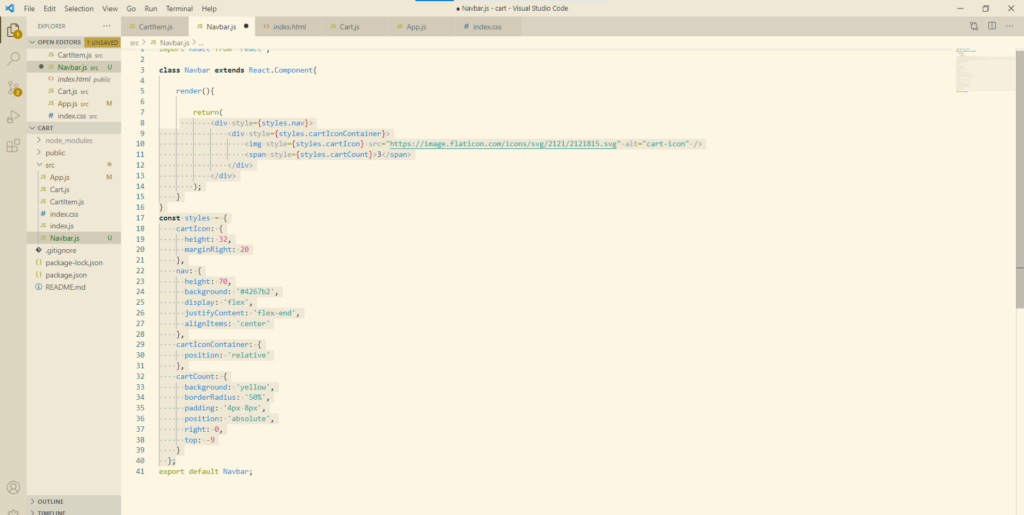
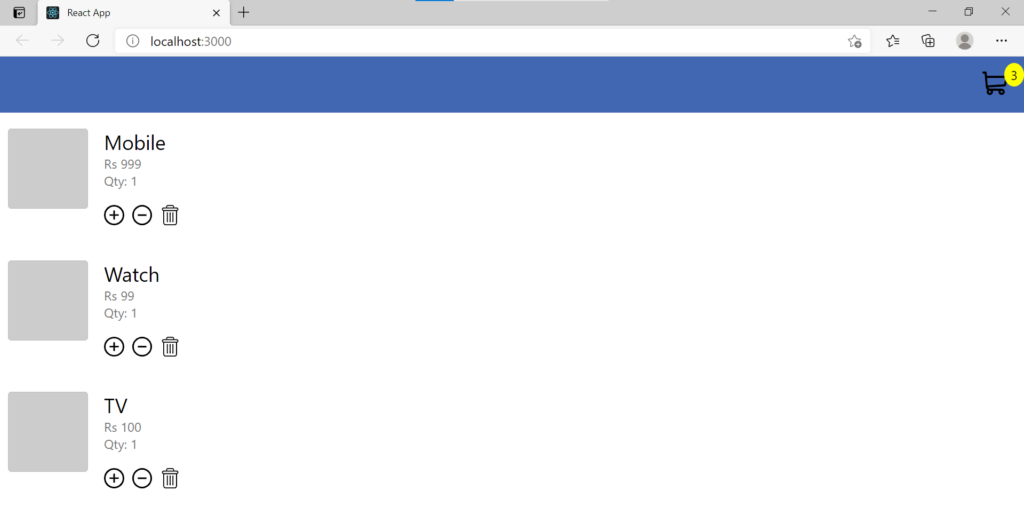
As of now the above code is a class component and it does not have any state. So we can change it to a fucntional component because it does not have any state. The way to do is as below:
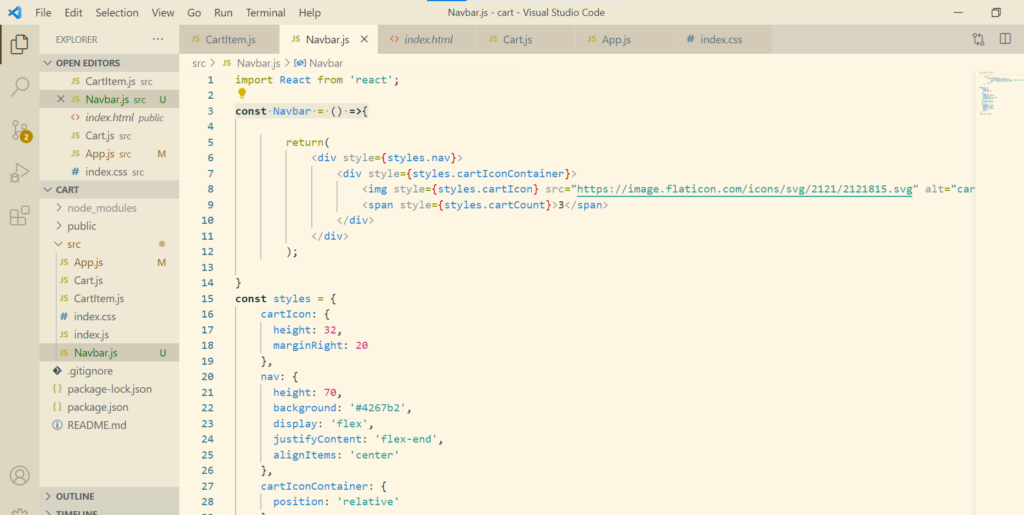
The react will pass props by default for every functional component. Therefore, we can pass it as argument.
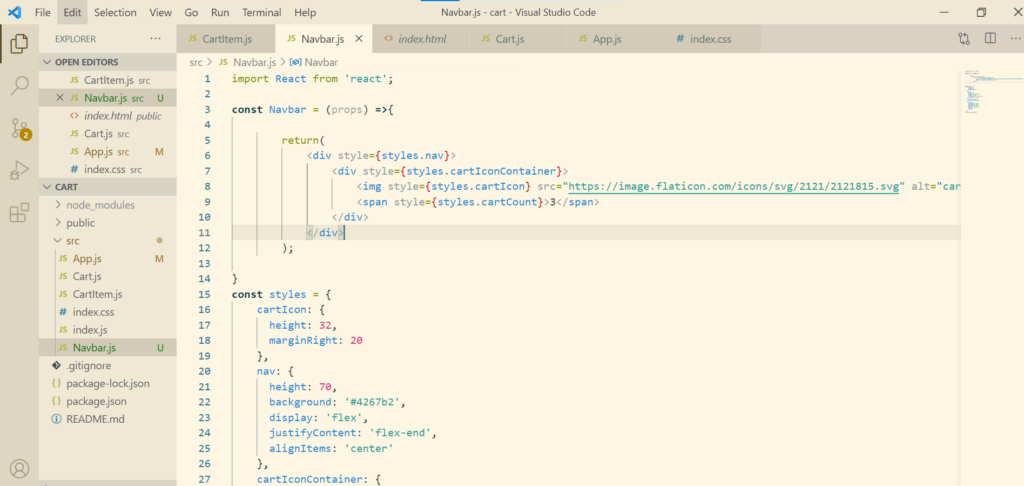
Similarly we can change CartItem to a functional component as well.
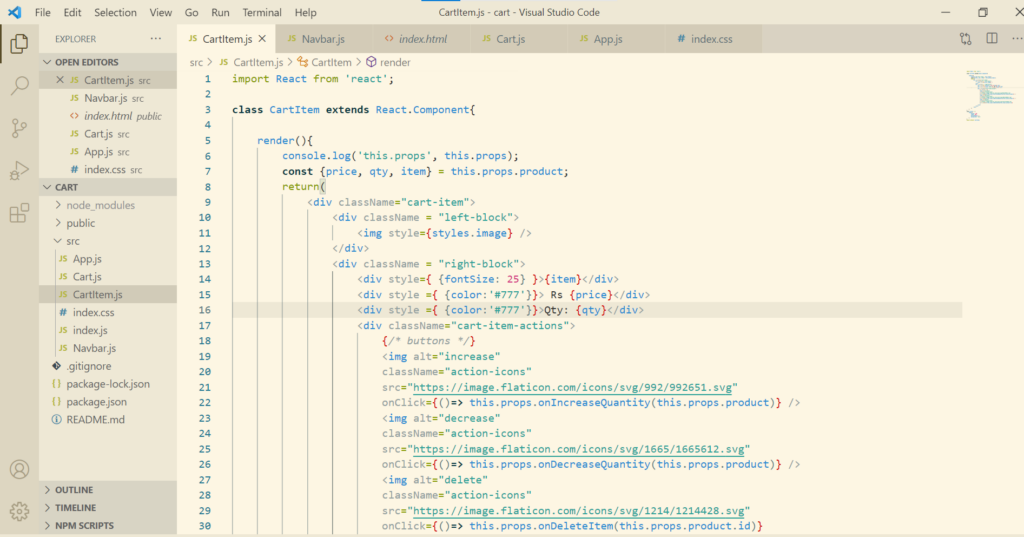
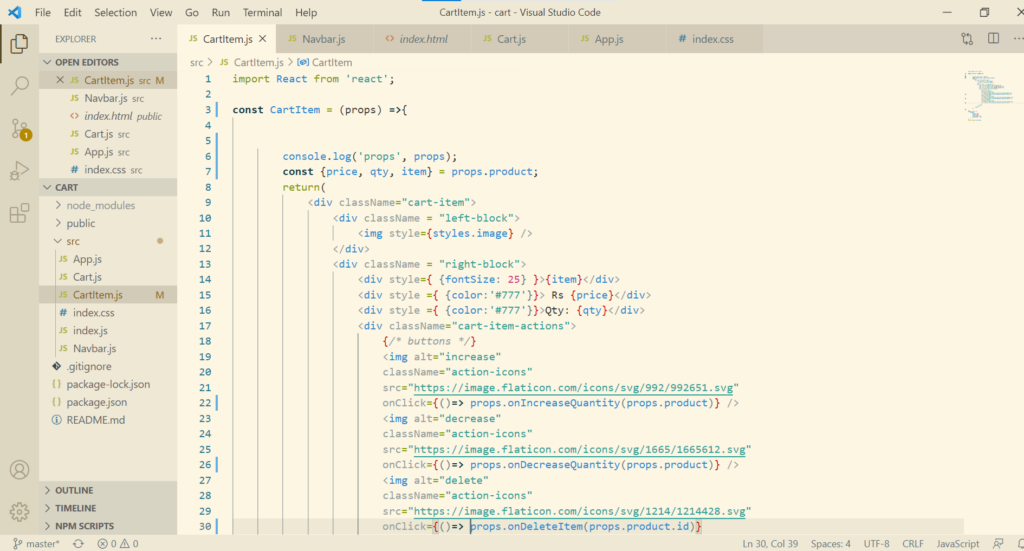
Adding Products Count To Navbar
Count of Navbar = Item1 qty + Item2 qty ….
We know that data flows only in one direction in React i.e from Parent to child via props. Here in the above case above two components are having a sibling relation. In such cases we move our state(in this case cart state) to a component which is common to both the above component, which will be parent. And that is the App
Let’s move the state to App
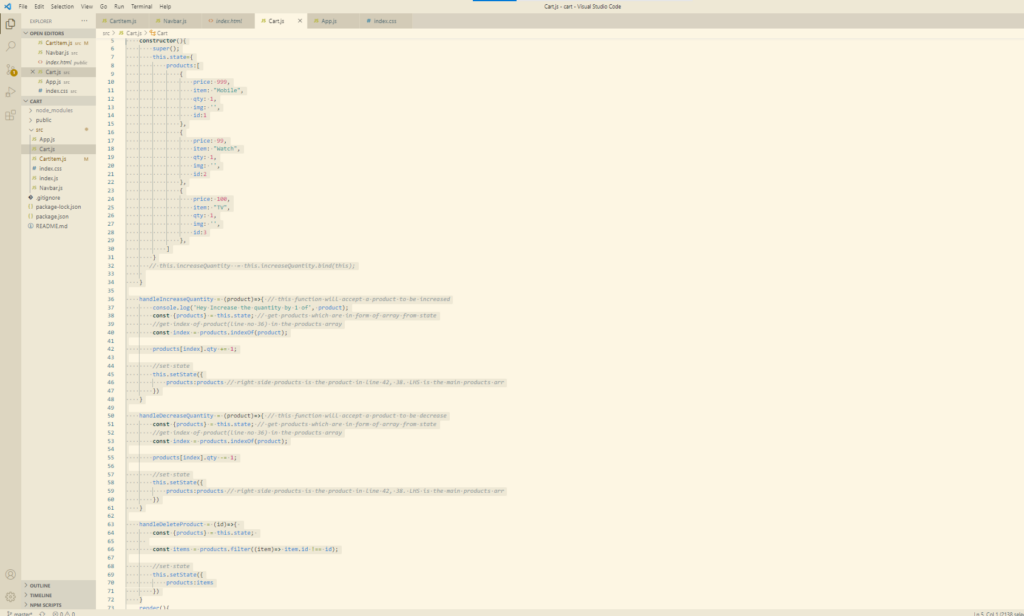
Making App as a class component and adding render function and pasting the above cut code.
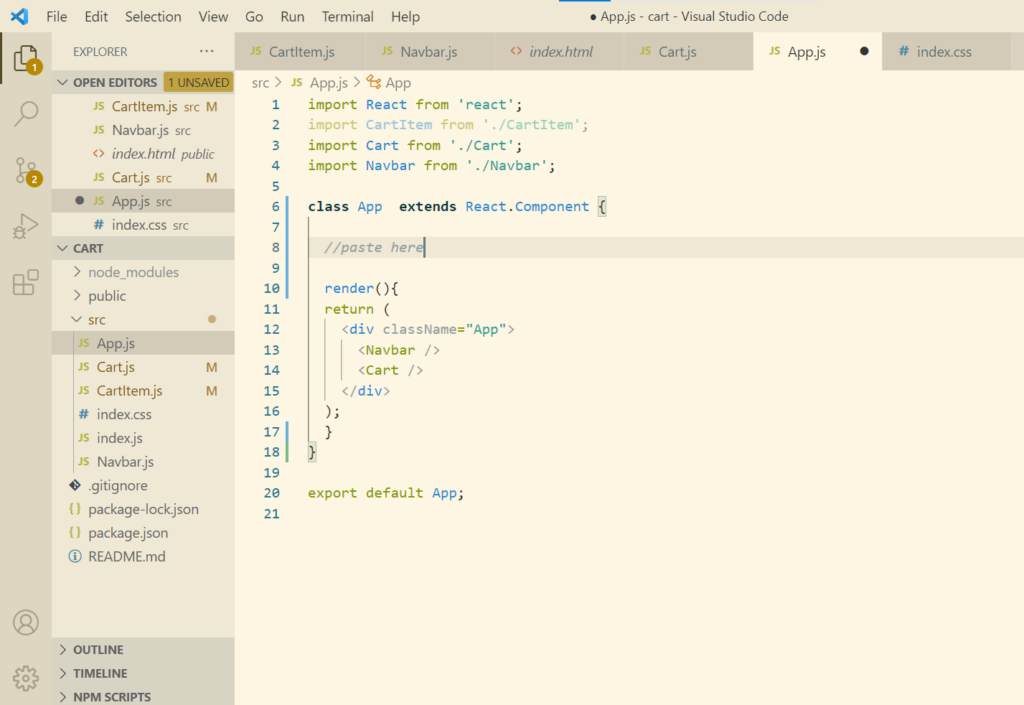
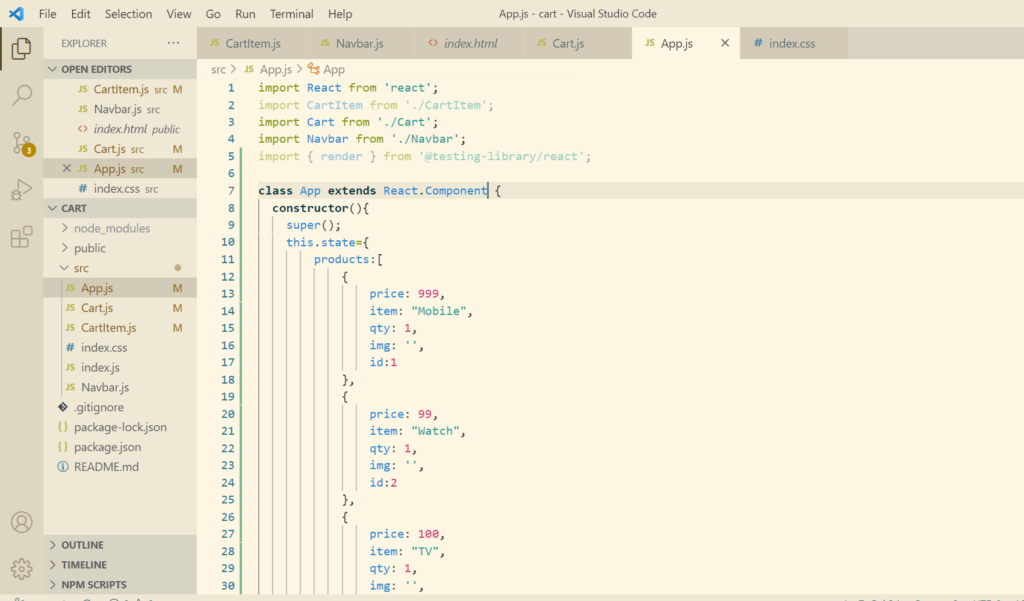
Now I want to pass all these functions defined like handleIncreaseQuantity etc to Cart component via props as that is missing.
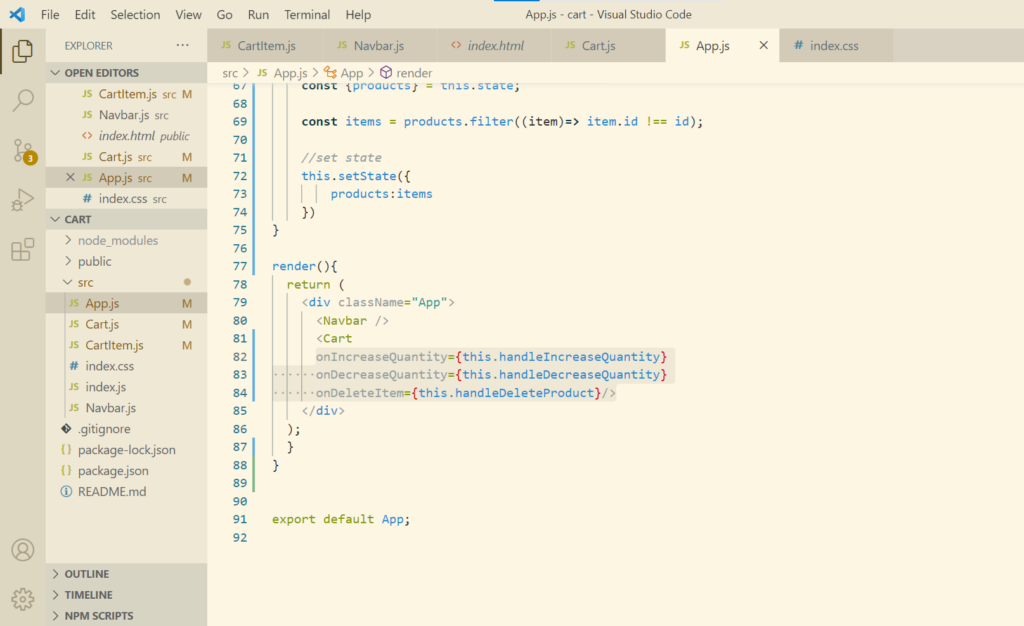
Now calling these functions using props in Cart.js
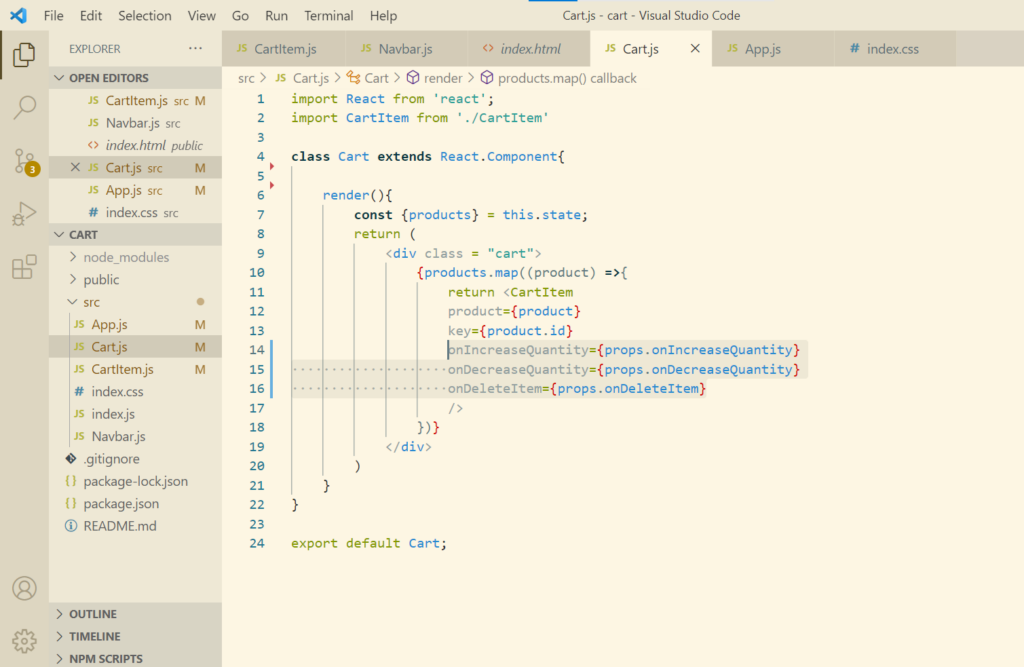
We also need to pass products in App.js and the Cart component inside it
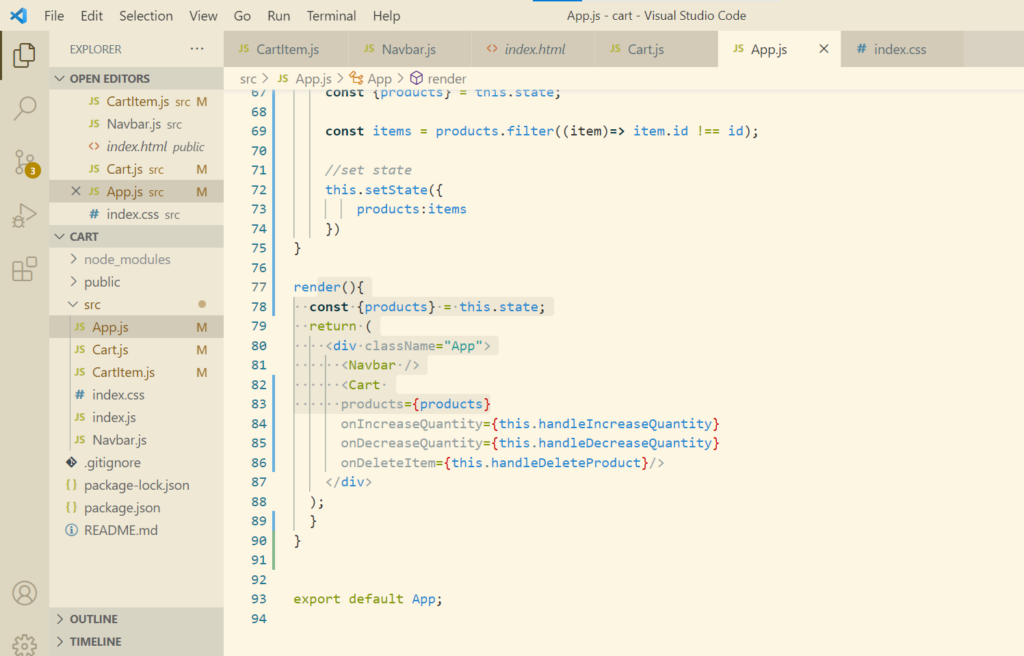
So we are not having access to this.state inside Cart.js component. So let’s change it to props.
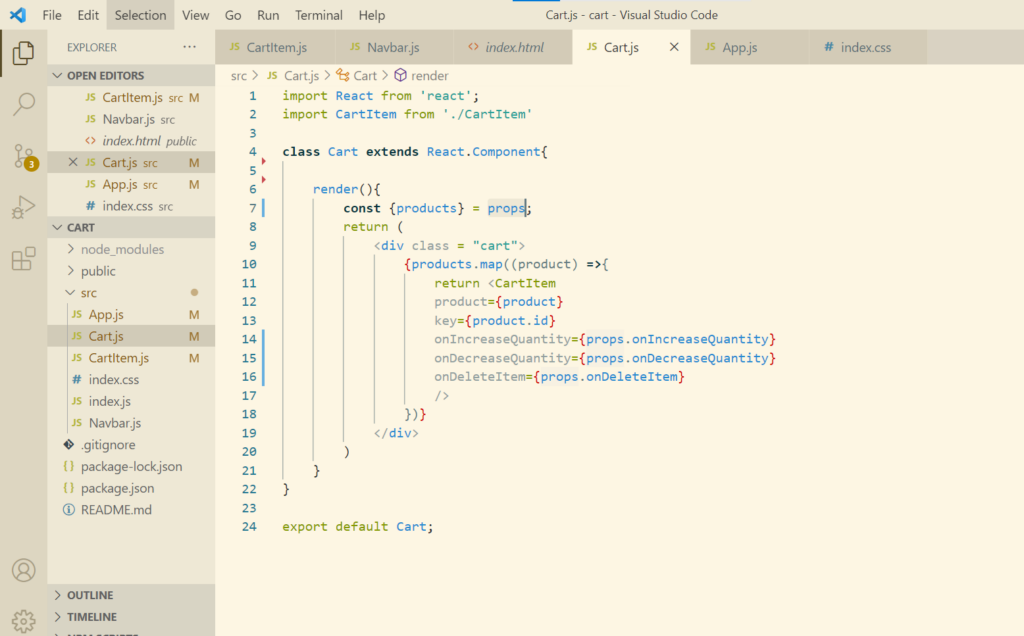
Let’s create Cart as a functional component as it does not have any state now.
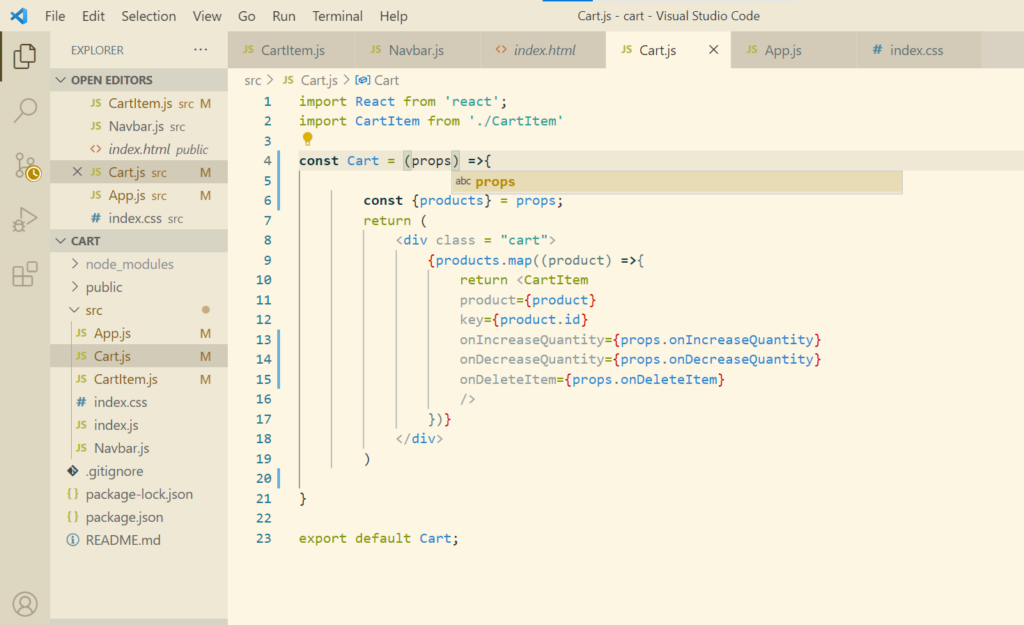
It time to check if everything is working fine or not
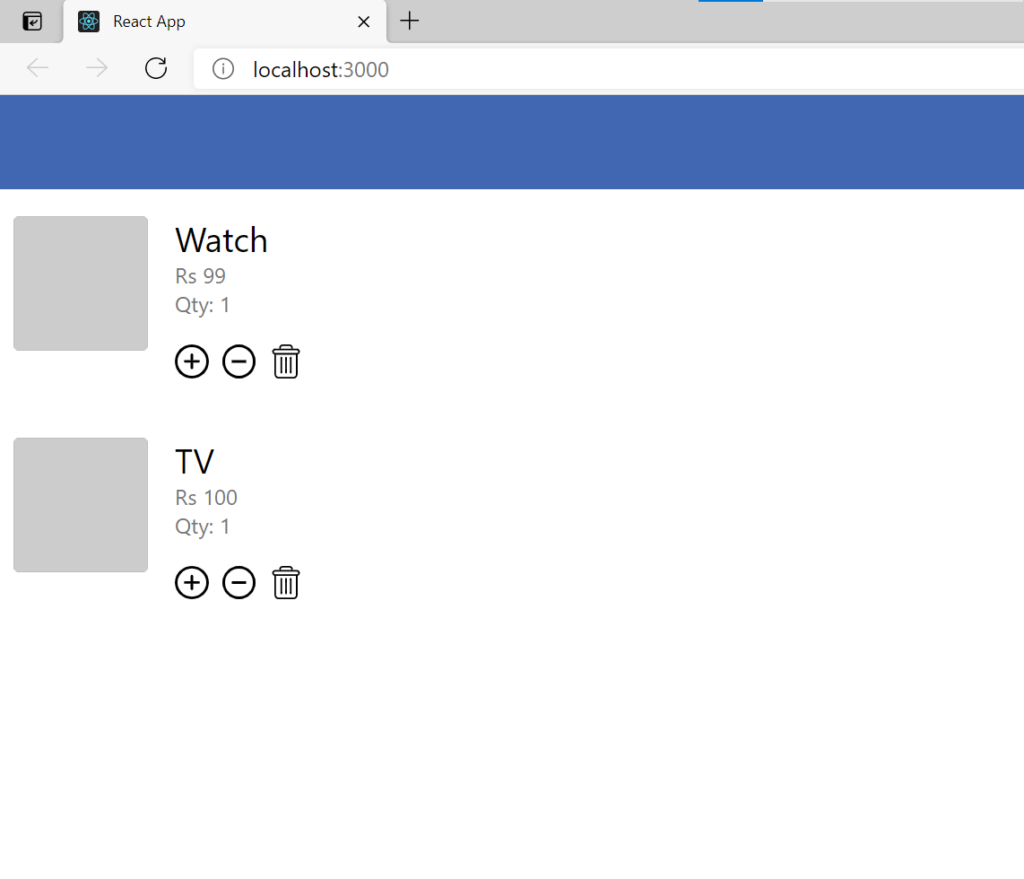
It’s time to make the quantity count dynamic in our Navbar.
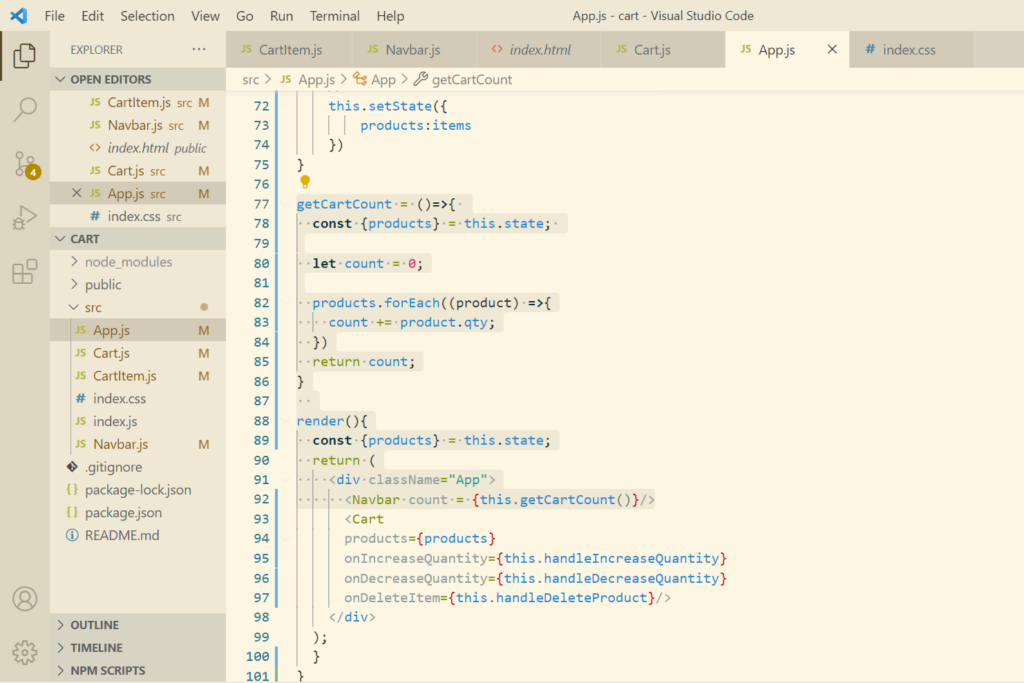
Let’s call this property in Navbar using props
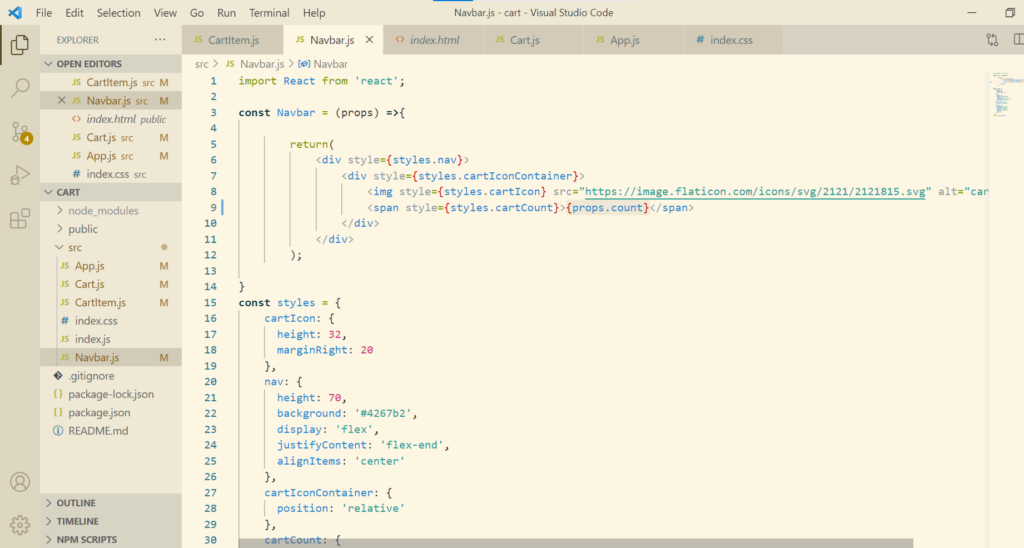
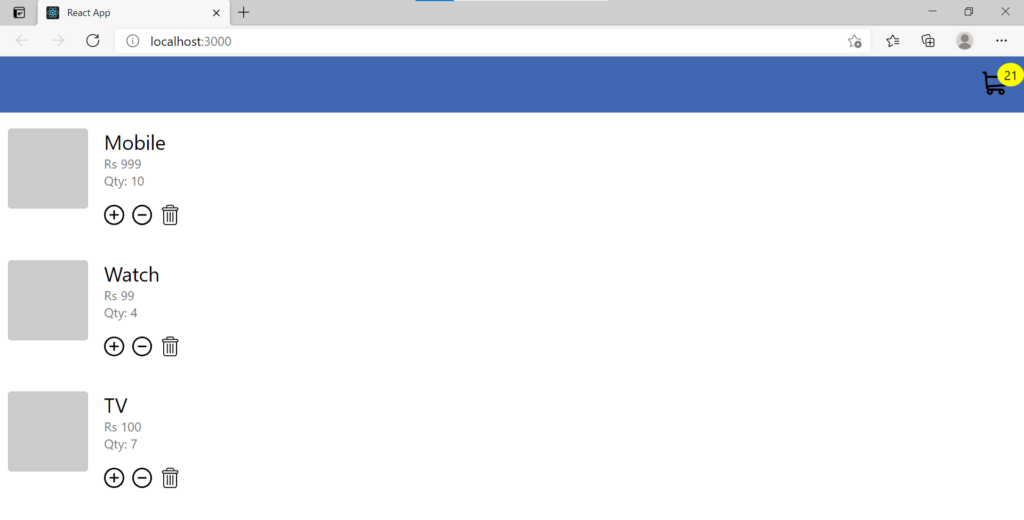
Adding Total Price
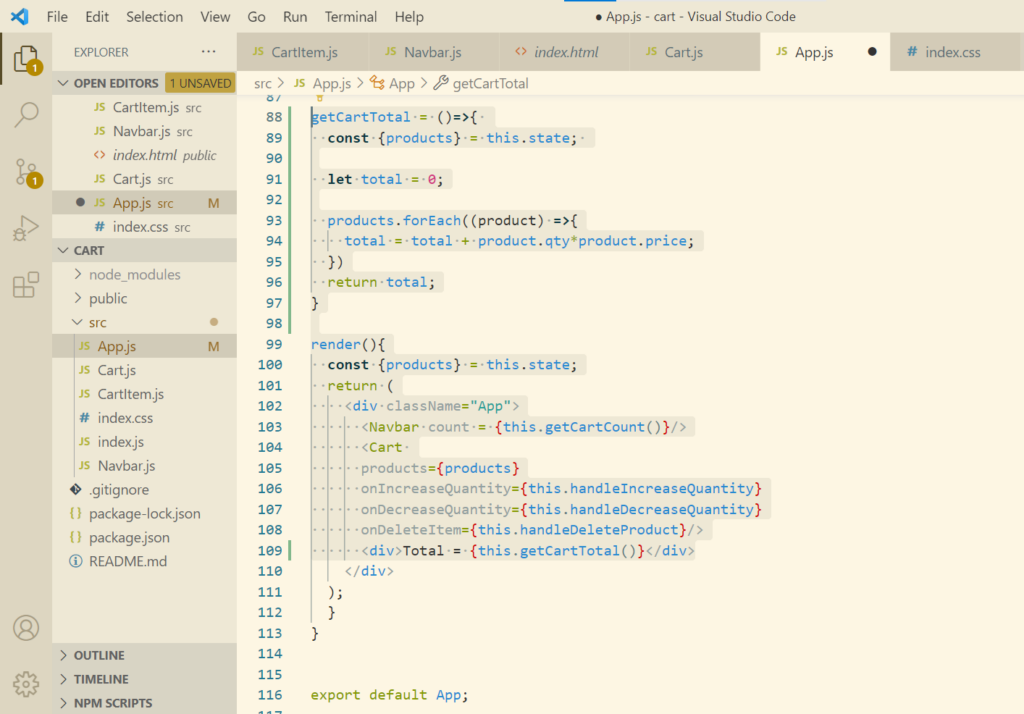
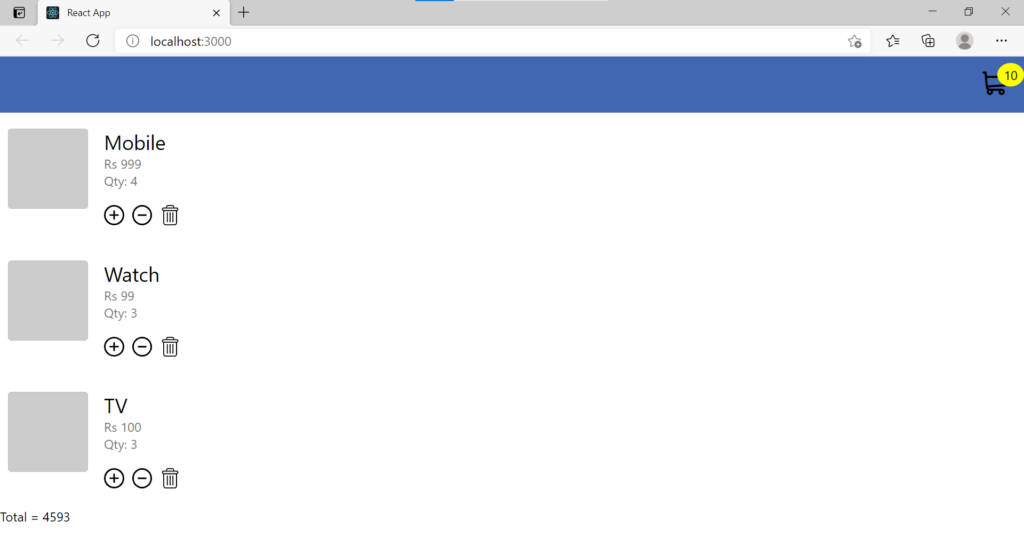