Everything is an object in Javascript. It is not 100% correct. There are few exceptions to it as well, which we will focus on in this blog.
In Javascript we have 2 different types of values:
- Primitives
- Objects
Primitives
All the datatype fall under this category like
- Number
- String
- Boolean
- Undefined
- Null
Objects
- Array
- Function
- Objects
- Dates
- Wrapper for Number, String, Boolean
As we know how important objects are in Javascript, let’s have a look at Object Oriented Programming.
Object Oriented Programming
- Objects interacting with one another through methods and properties
- Used to store data, structure application into modules and keeping code clean
Till now we have only worked with a single object john. Let’s say if I have to create 3 more objects, I would have created them explicitly. That would have been a tedious task.
But what if we have some blueprint, from which we can create person objects. We can do that in Javascript.
This is how blueprint should look like. In other programming language this blueprint is called a class, but in Javascript we call it a constructor or a prototype.
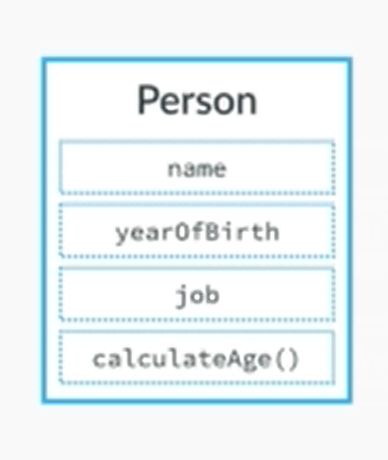
Based on this constructor, we can create as much instances as we want just as below.
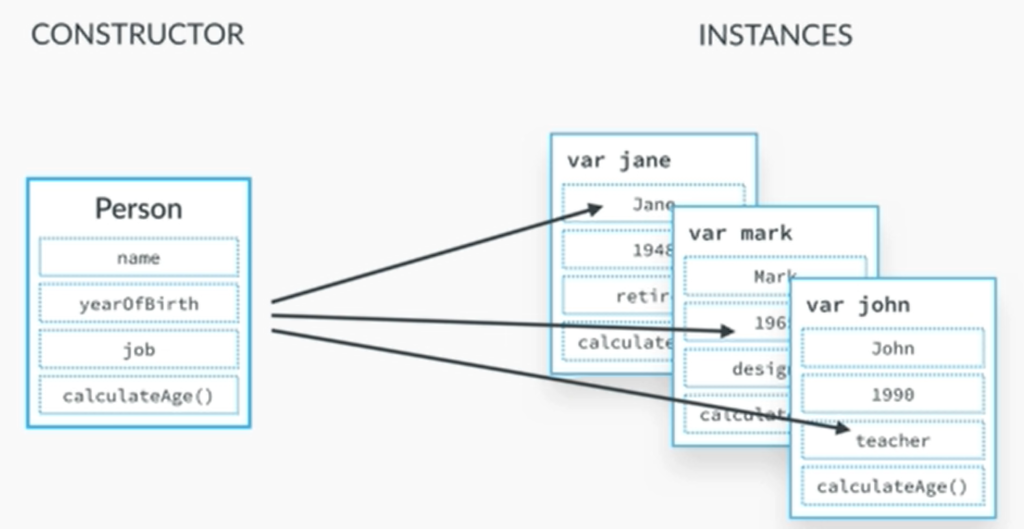
Inheritance In Javascript
Inheritance in Javascript, is when an object is based on other object. In simple language, when an object gets access to the properties and methods of other object.
Let’s understand this with some simple examples
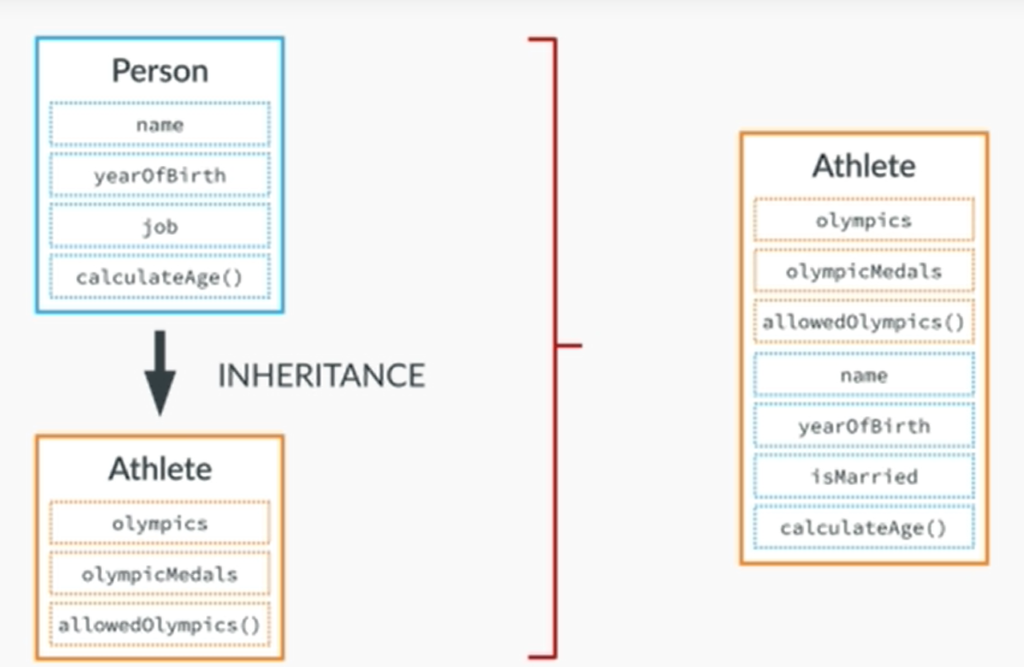
In the above examples, there are 2 constructors Person and athlete. However, athlete do have a name, yearOfBirth and many other things available in person constructor. Rather than declaring the same things over and again in the athlete constructor, athlete constructor can inherit the properties from person constructor. This is how inheritance works in all programming language based on Object Oriented Programming.
Let’s understand how this works for Javascript with the same example.
Javascript is a prototype based language which means Javascript use inheritance by using something called as prototypes. In practical it means, each and every Javascript object has a prototype property which makes inheritance possible in Javascript.
Behind the scenes
Let’s see behind the scenes how Javascript use inheritance using the same example.
In the previous example, we have a person object is a constructor and john is one of the instances of the person constructor. Now if we want john tpo inherit a method/property from Person object, we have to add that method/property to the person prototype property. Here in the below example we have calculateAge() method and john is able to inherit the method. Also, any instance created from person constructor will inherit the calculateAge() method.
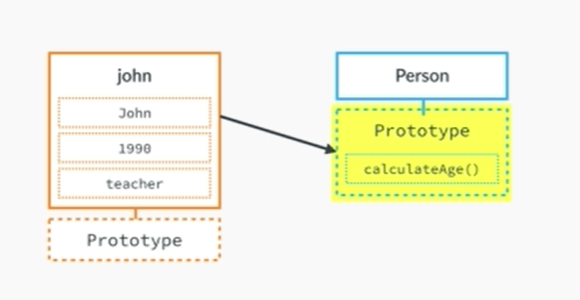
The Prototype property of an object is where we put method and properties that we want other objects to inherit.
Here Person object itself is a prototype of a much bigger constructor, which is the Object object. This is how its works. Each and every object we create, is an instance of Object constructor and we can call the method from the Object constructor. Below is the diagram which will represent the same.
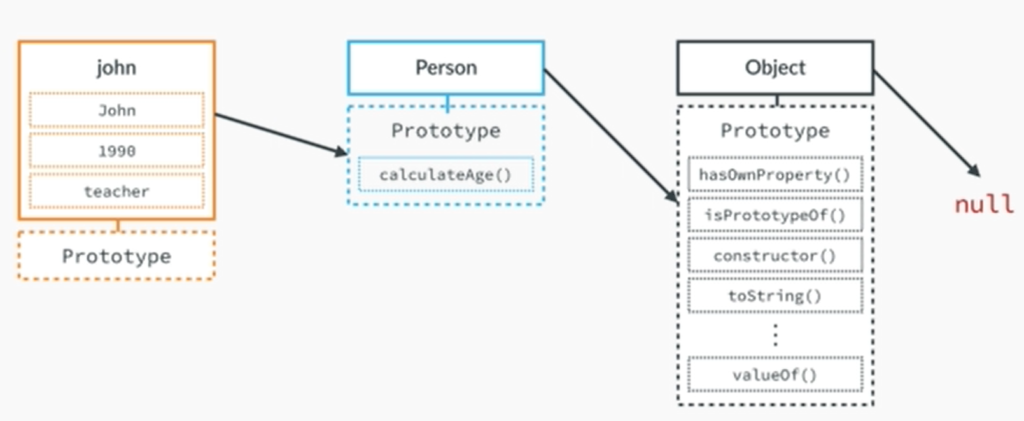
If we call a method, it first searches in its own object. If not found, then it searches in the prototype property of its parent and this chain continues. If still not found it reaches the topmost hierarchy which is null and undefined is returned to the user.