Let’s launch EC2 instances using Ansible.
When we create EC2 instances we need to login to AWS GUI. Ansible won’t be doing that. Ansible will login using API calls. Ansible will do it in 2 ways:
- IAM user
- IAM roles
Login via IAM user
Create a IAM user on AWS. And give it full access.
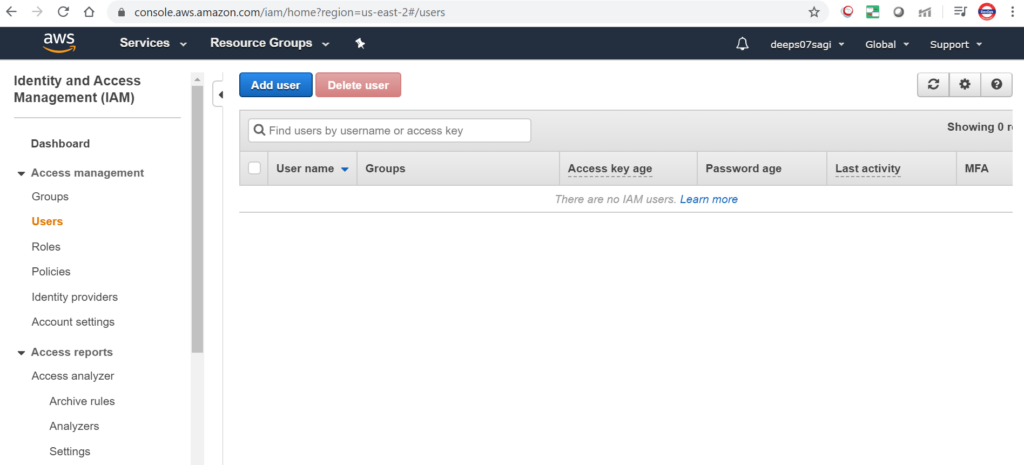
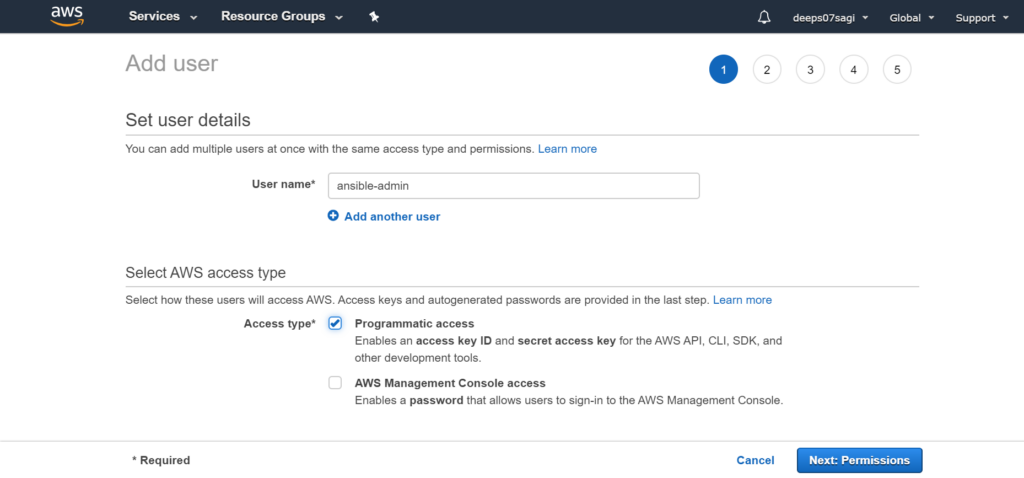
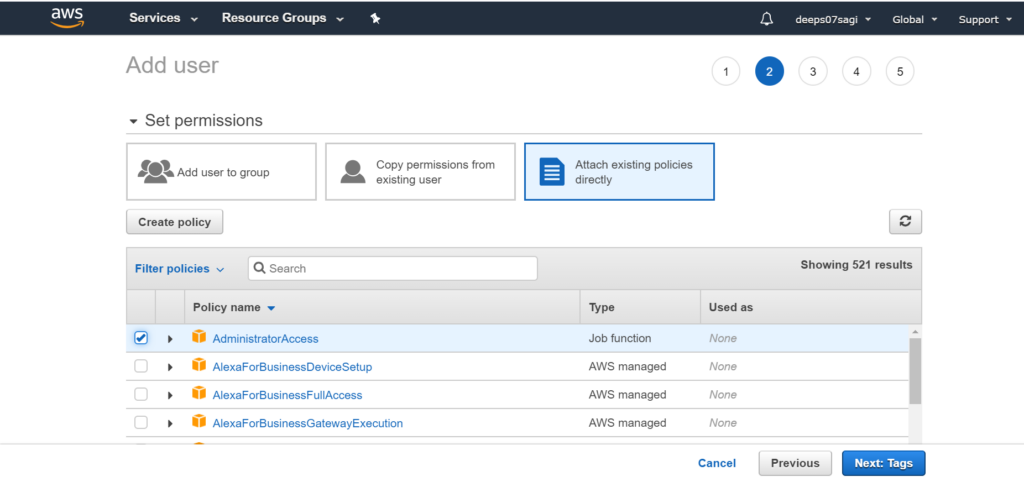
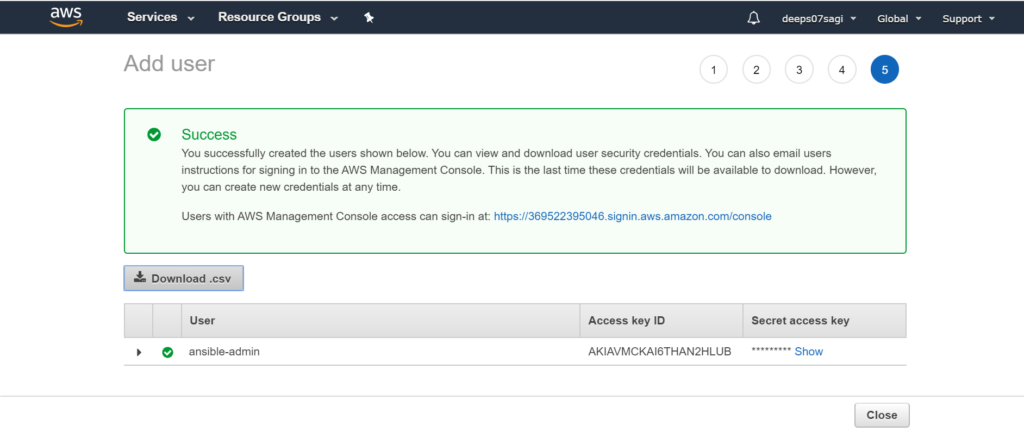
Download the credential file. This credential file consists of Access Key and Secret key. Do not share this file with anyone. However, this approach is not recommended. We will look into IAM roles later which is recommmended.
Let’s keep this access key and secret key on our machine. Rather than keeping it in playbooks, it would be better to keep it in .bashrc file.
We can pick the syntax of exporting the keys from the documentation.
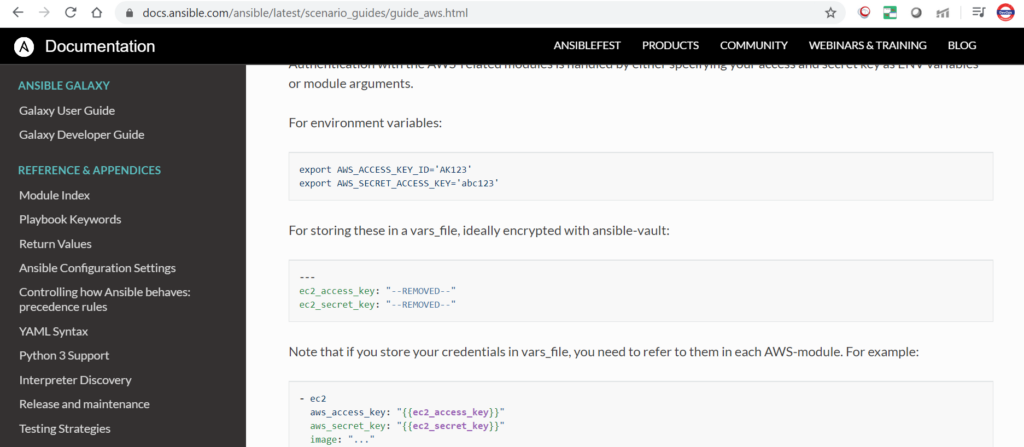

Copy above two line and paste it in .bashrc file

Let’s write test playbook to check. yml code can be fetched from the same documentation as above.
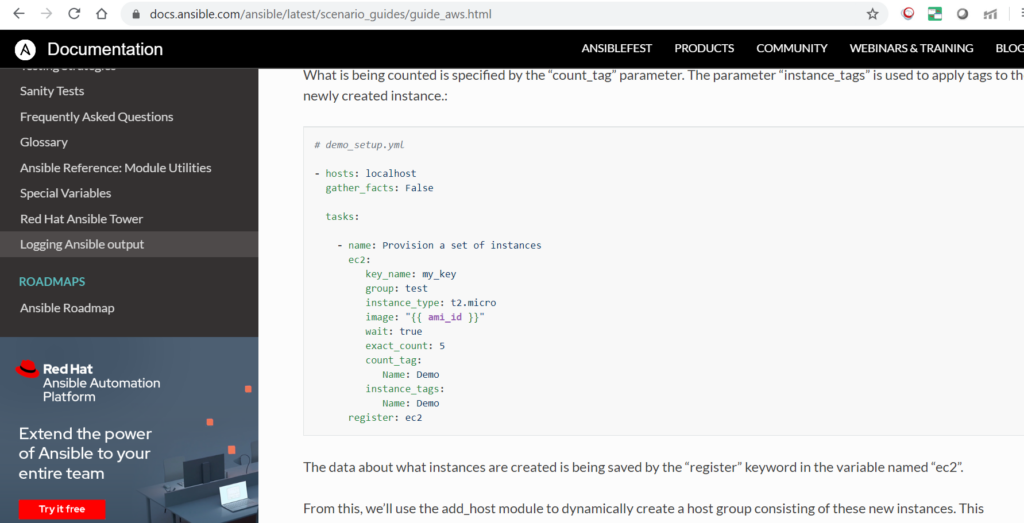
Create a playbook.

- hosts: localhost
gather_facts: False
tasks:
- name: Provision a set of instances
ec2:
key_name: my_key
group: test
instance_type: t2.micro
image: "{{ ami_id }}"
wait: true
exact_count: 5
count_tag:
Name: Demo
instance_tags:
Name: Demo
register: ec2
//register will hold all the information which will be executed in tasks
Let’s replace the values above. Creating a key as my_key, providing a ami_id, changing the count and security group.
- hosts: localhost
gather_facts: False
tasks:
- name: Provision a set of instances
ec2:
key_name: my_key
group: AnsibleServerNew-sg
instance_type: t2.micro
image: ami-097834fcb3081f51a
wait: true
exact_count: 1
count_tag:
Name: Demo
instance_tags:
Name: Demo
register: ec2_info
- debug:
msg: "{{ec2_info}}"
Let’s test it now. But before that I need to source my .bashrc file which will export access key and secret key
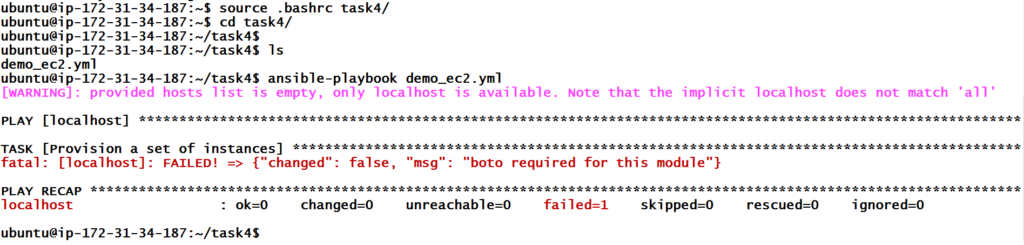
On running the playbook we got an error that boto module is required. For ansible to make API calls to AWS, ansible will require various boto modules. Boto is a python module, which will help in connecting to AWS.
We would be requiring few boto modules. Let’s install them all.
ubuntu@ip-172-31-34-187:~/task4$ sudo apt install python-boto python-botocore python-boto3 python3-botocore python3-boto -y

Let’s run our playbook again.

It is asking to specify a region. Let’s specify a region in our playbook. Obviously, we will use the docs to pick up region parameter.
- hosts: localhost
gather_facts: False
tasks:
- name: Provision a set of instances
ec2:
key_name: my_key
group: AnsibleServerNew-sg
instance_type: t2.micro
image: ami-097834fcb3081f51a
region: us-east-2
wait: true
exact_count: 1
count_tag:
Name: Demo
instance_tags:
Name: Demo
register: ec2_info
- debug:
msg: "{{ec2_info}}"
Let’s run our playbook now.
And its a success.
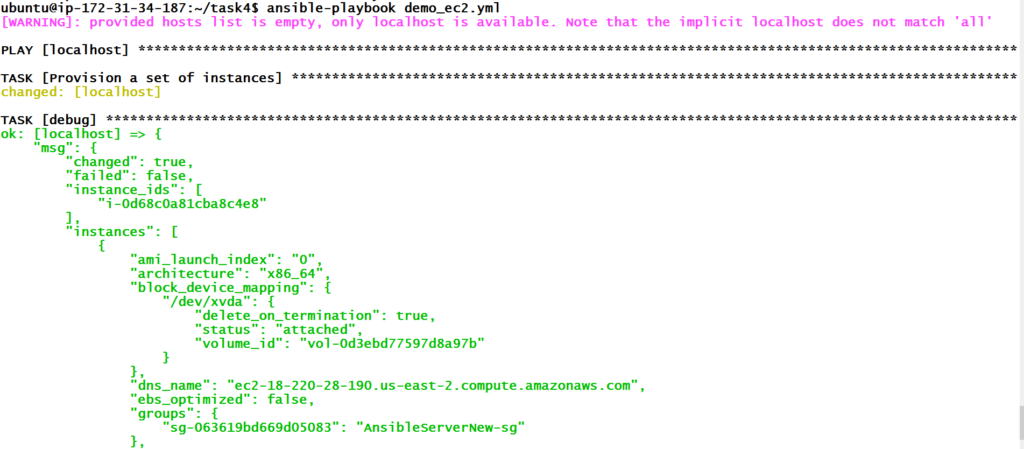
This info we have received because of register and debug module.
We can see demo instance being created:
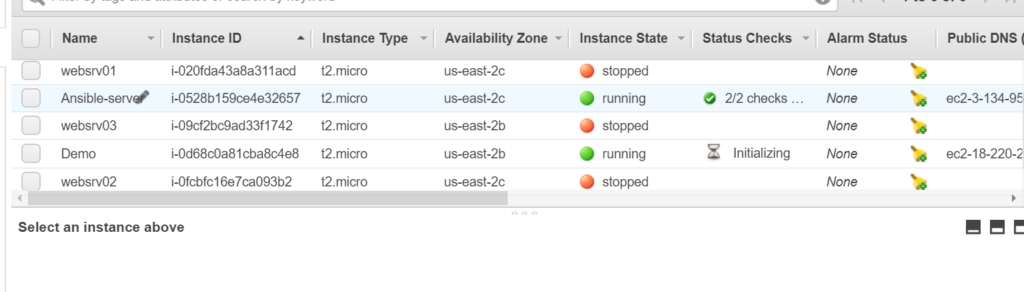
Now let’s see how this thing can be done using IAM roles. I will now delete the exported variables from .bashrc file and logout and login again so that all the exported variables are gone.
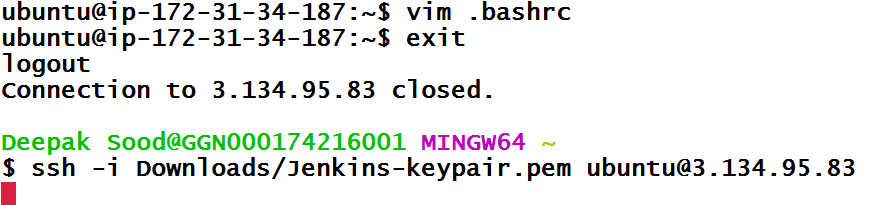
Let’s run our playbook again.

We get an error that there are no credentials
Now in AWS we will create roles. Roles are premission to the service, not to the user.
Now I will give permission to EC2 instance where Ansible is installed.
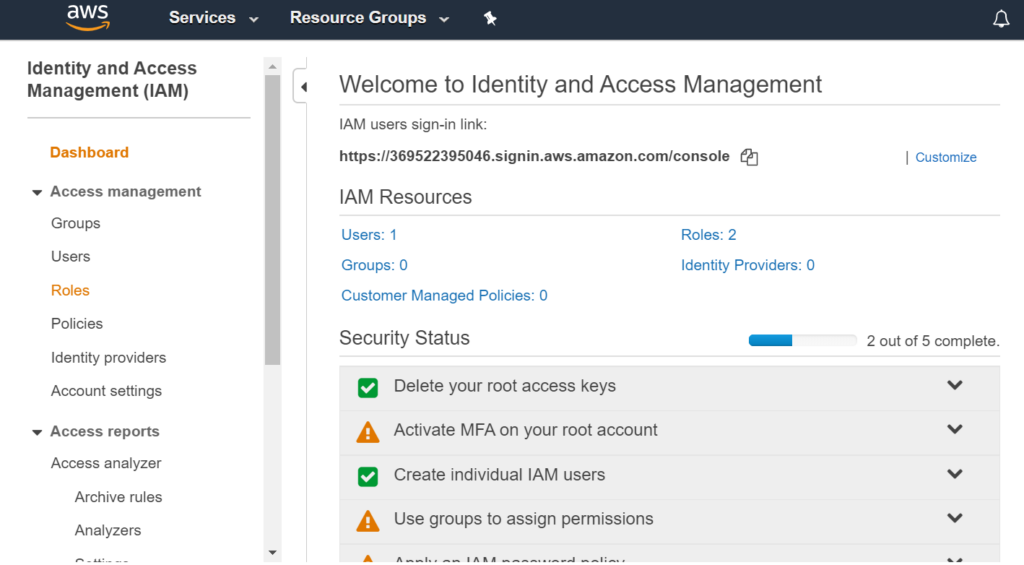
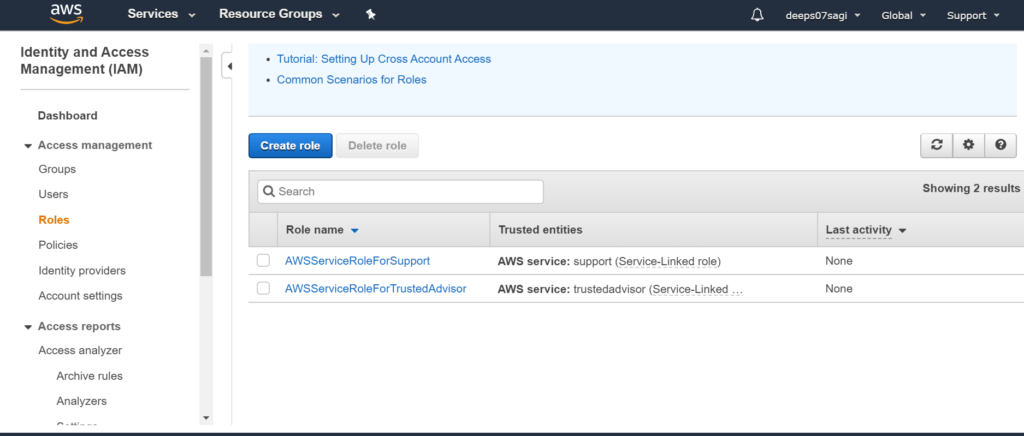
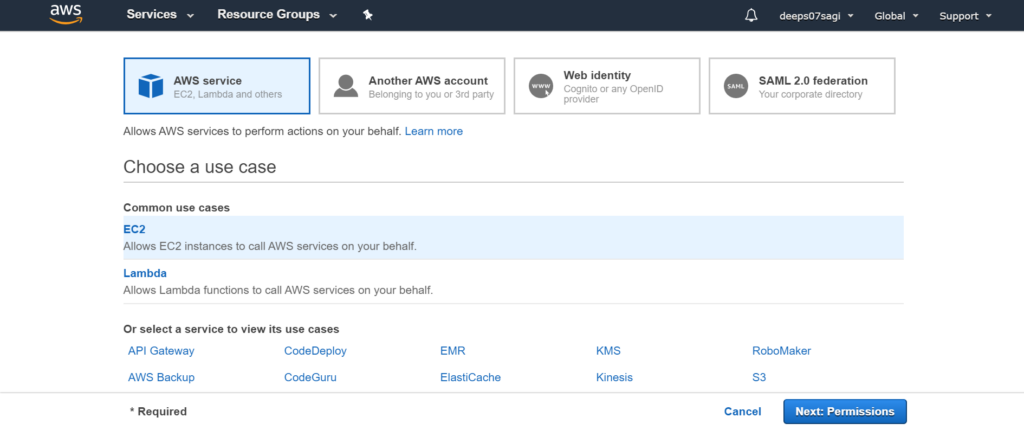
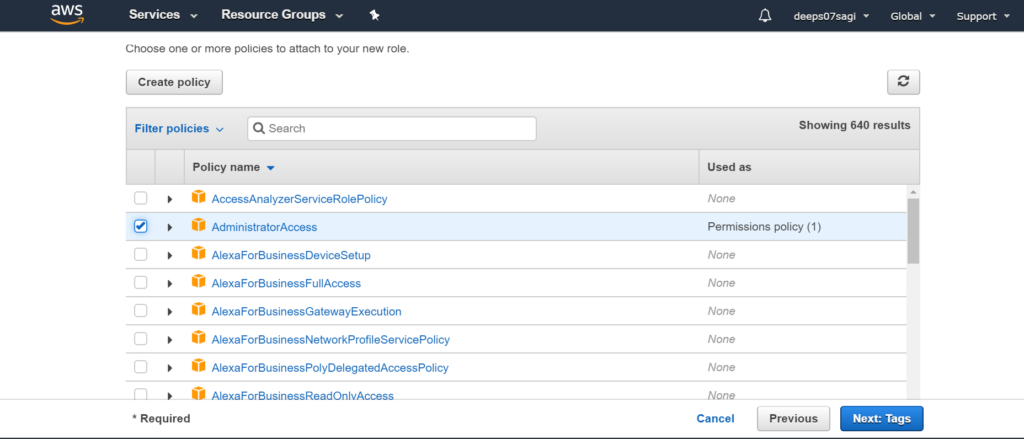
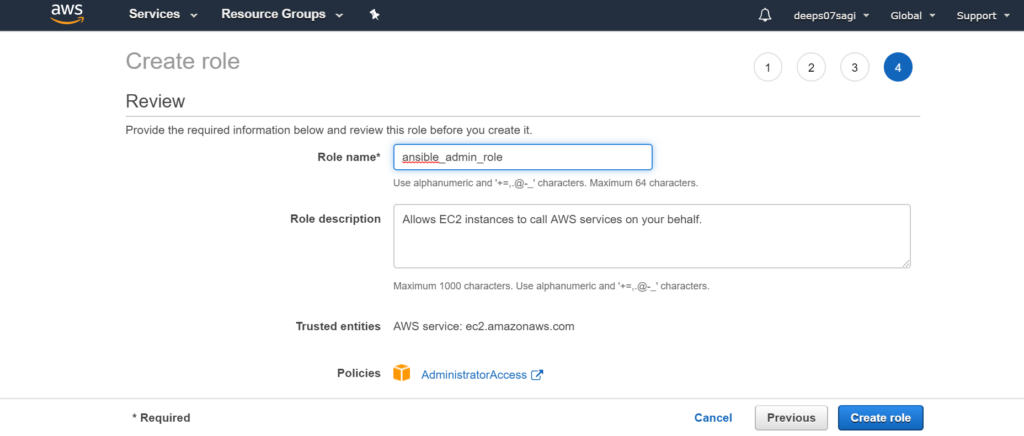
Now attaching the role to EC2 instance.
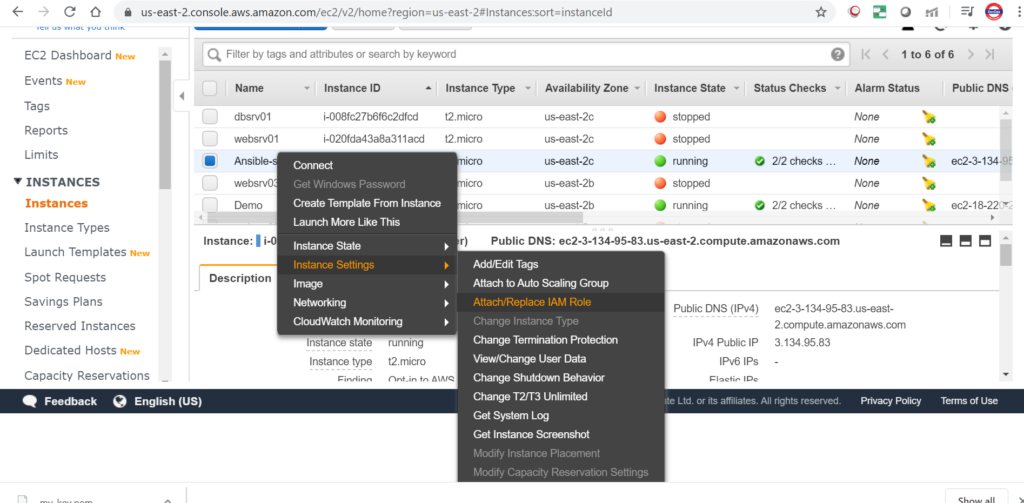
All the setting have been completed. let’s run the playbook again.
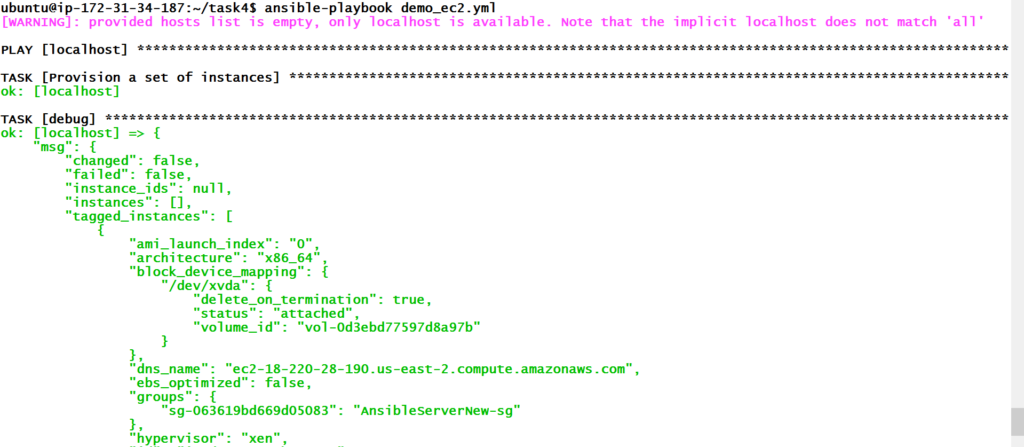
No errors. This will not create another EC2 instance. There is a information in our playbook which will check, if the EC2 instance is already launched, it will not launch it again.
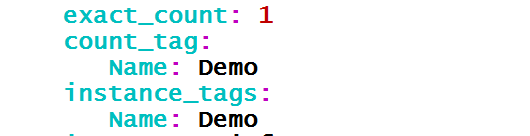