Consider a basic HTML Page, not consisting of any Javascript.
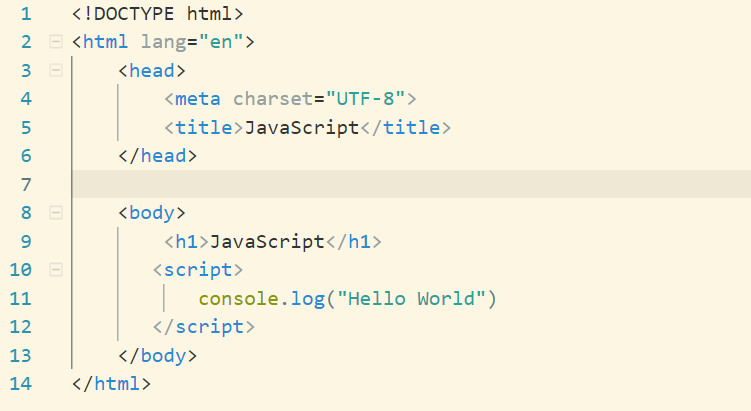
Javascript can be included in this HTML inline or from external source. Let’s add inline script.
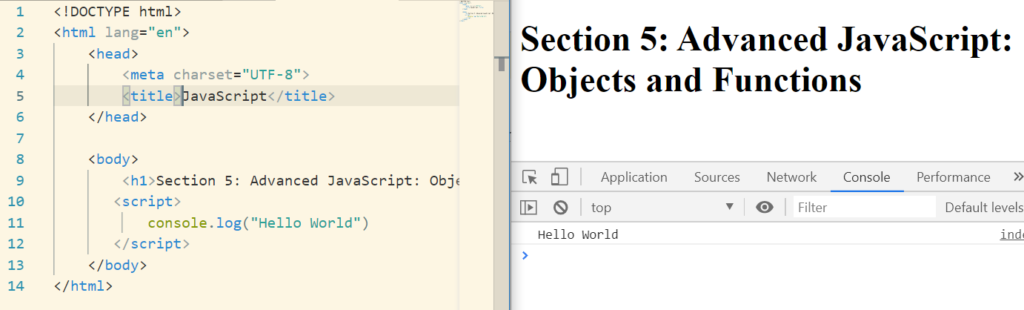
Adding a javascript from external source named script.js
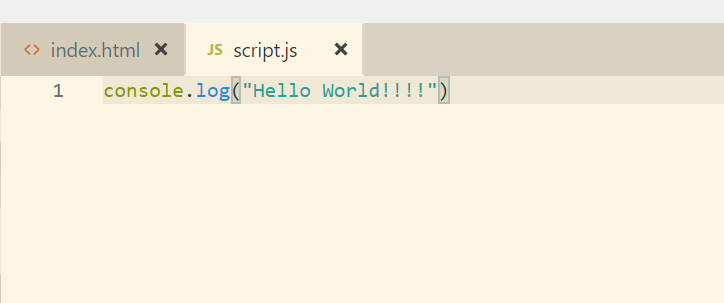
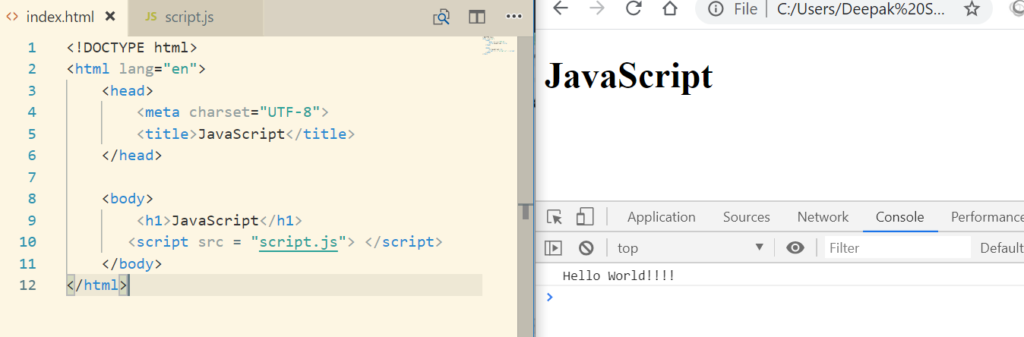
What is Javascript ?
- Javascript is a lightweight, cross platform, object oriented computer programming language
- JavaScript is one of the three core technologies of web development
- Today, Javascript can be used at client side as well as server side as well. Howver we require node.js along with Javascript at server side
- Framework libraries like React and Angular are 100% based on Javascript.
Javascript in Web Development
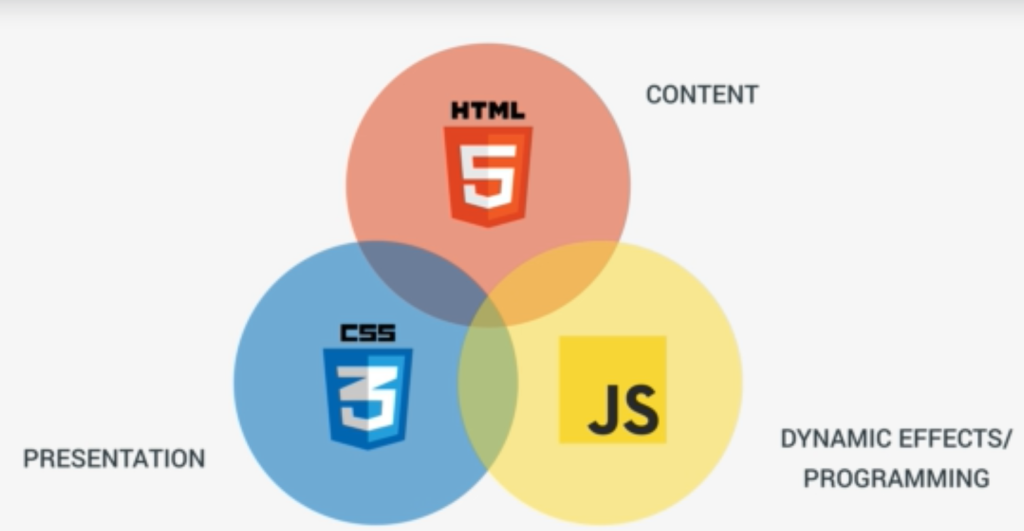
Variables
Variables in Javascript is similar to variables in other programming language. Variable can be considered as a container which stores some value.
Using keyword var, we can store value in a variable. var name = ‘Deepak’
In Javascript we have following different types of datatypes:
- Number
- String
- Boolean
- Undefined
- Null
Javascript has a feature called DYNAMIC TYPING. Using this feature we need not to define specific datatypes(like int x = 10, String str = “Hello”), unlike other languages.
Number, String and Boolean are self explanatory. Undefined is when a variable is defined but no value is assigned to it. Later on in the code the variable is being used, without the value. Then we will get value as undefined. Null means non existent.
Difference between alert and prompt
Alert will display a pop up on the screen whereas prompt will display a popup along with entry field, which you can store in a variable
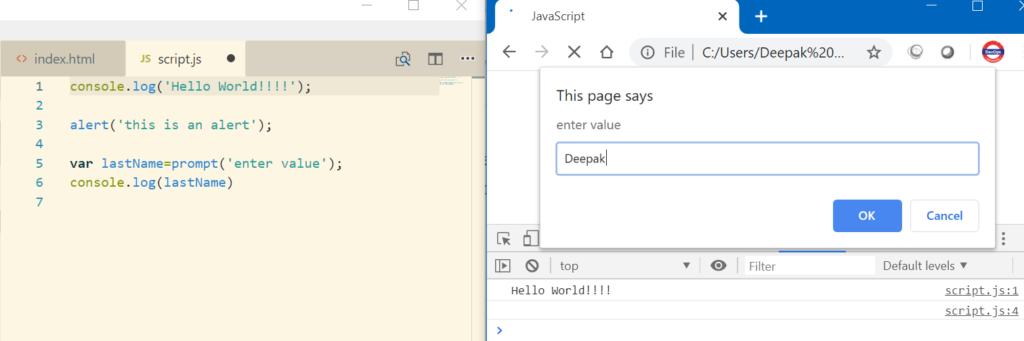
Operator
All the basic operator in Javascript is similar to other programming language. There is one operator which is not available in many language that is typeof.
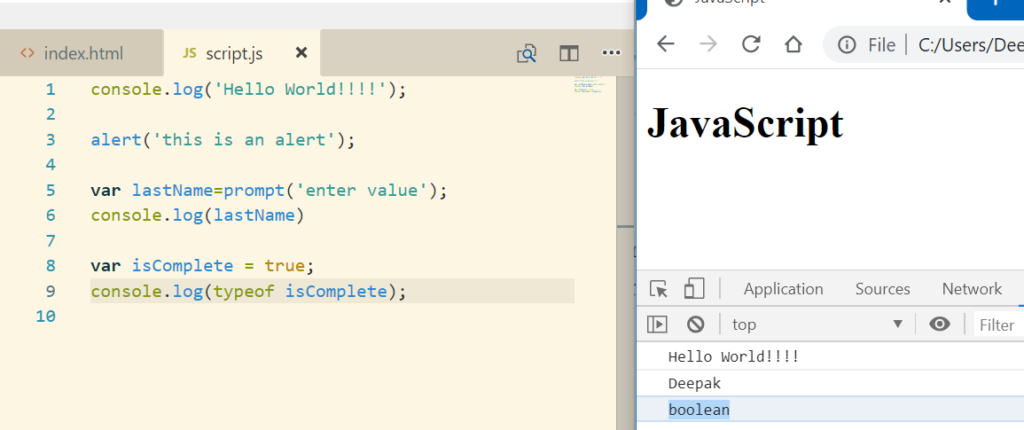
Ternary Operator
Ternary operator is similar to if/else. It is used at simple situation only. Ternary operator is having 3 parts to it. 1. the condition 2. the if statement 3. the else statement
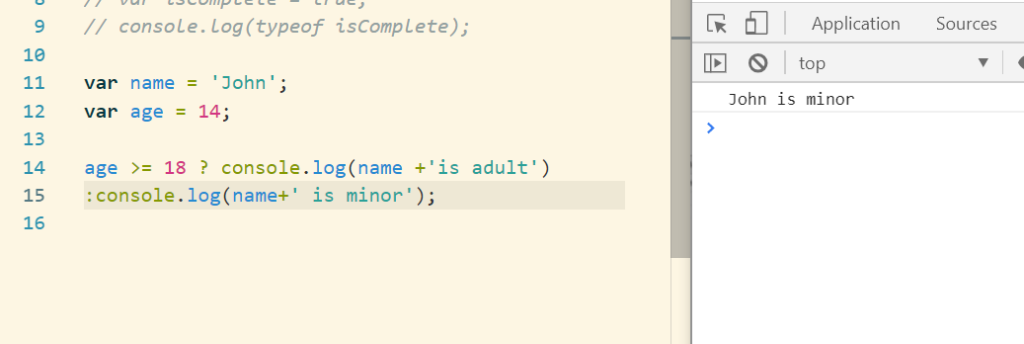
Equaity Operators(===, ==)
=== does the exact match(value and the datatype)
== matches only the value, not the datatype
Functions
Functions are like containers, which have some line of code and they return us some value in the end.
Let’s create a function
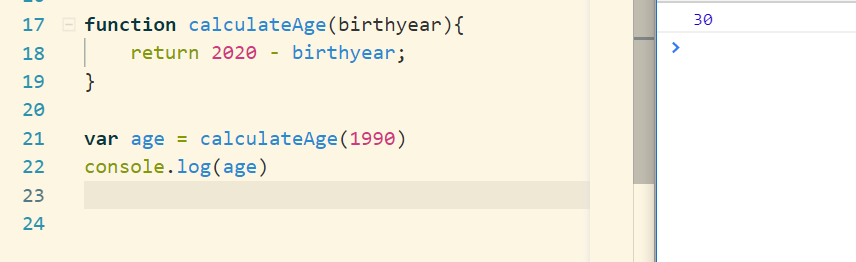
Let’s now create 1 more function which will calculate years until retirement. Also, the function will check if the person is already retired or not.
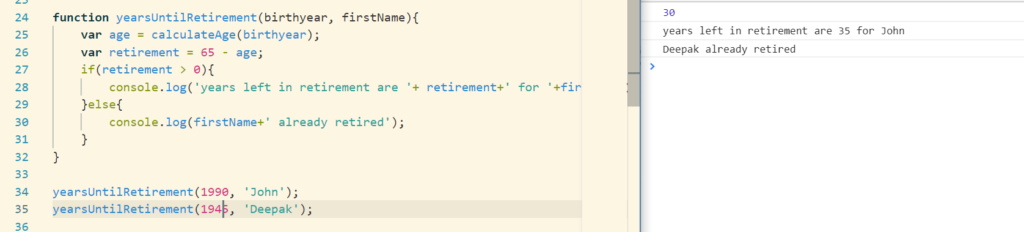
Function Statements And Expression
Till now we have learnt how to write a function, which is called function declaration, but there is one more way to write function i.e using function expression.
In function expression we assign a function to a variable. The passing of parameter and calling the function works the same way in function expression.
Let’s write a code for the function expression
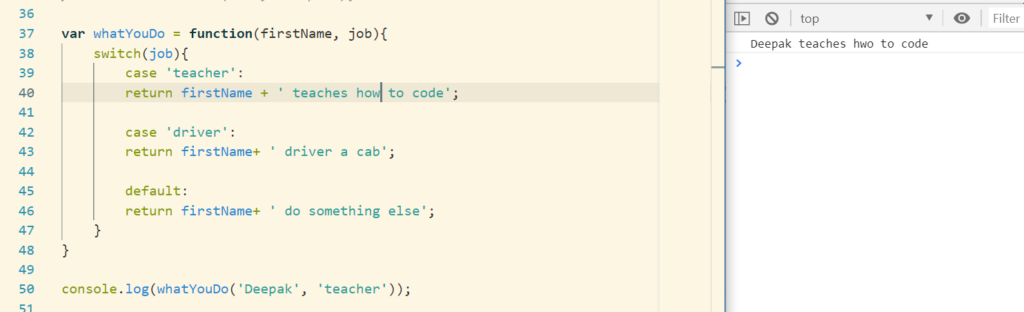
Difference between Function Expression and Function Declaration
The main difference between function expression vs function declaration is that, function expression return you a value always, unlike in the case of function declaration which do not produce any immediate value.
For instance whatYouDo is a function expression
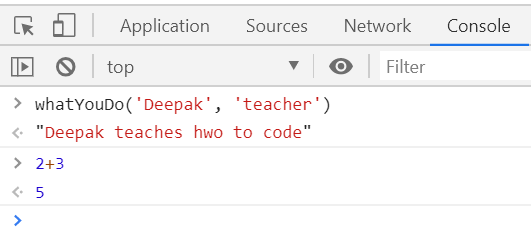
Arrays
Arrays are collection of variables, which can be of different datatype as well. Let’s jump into practical directly
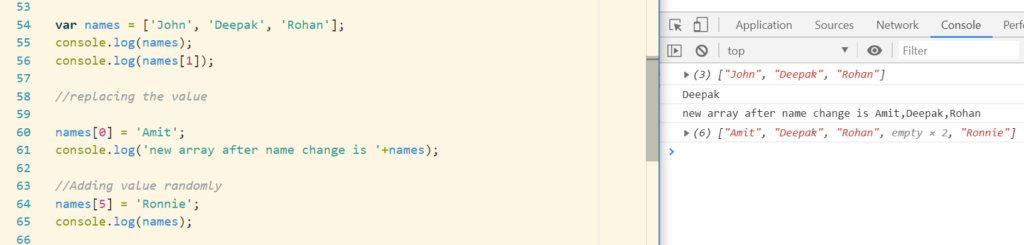
Some more operations on array are as follows:
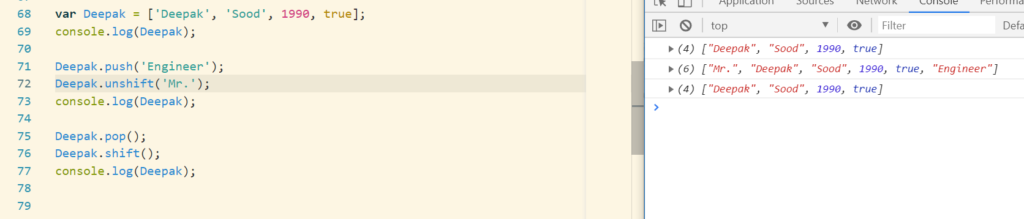
Objects And Properties
We can use objects to group together different variables that belong together and have no particular order. Let’s create an object:
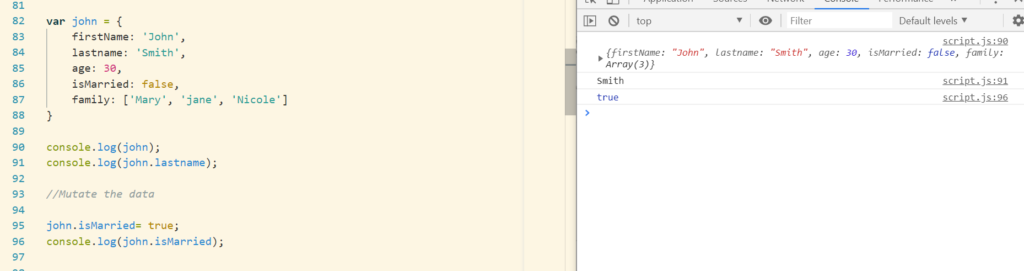
Other way to create an Object is using new Object syntax as follows
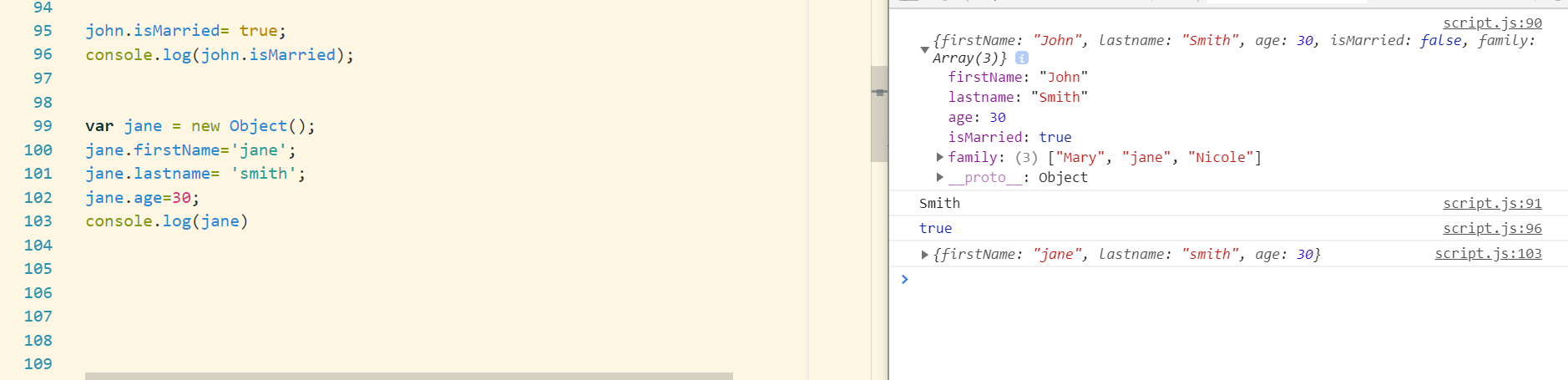
Objects And Methods
As we already know that objects can store different types of data like String, number, boolean, array. In this topic we will have a look at the fact that objects can hold functions as well, which are called methods in objects.
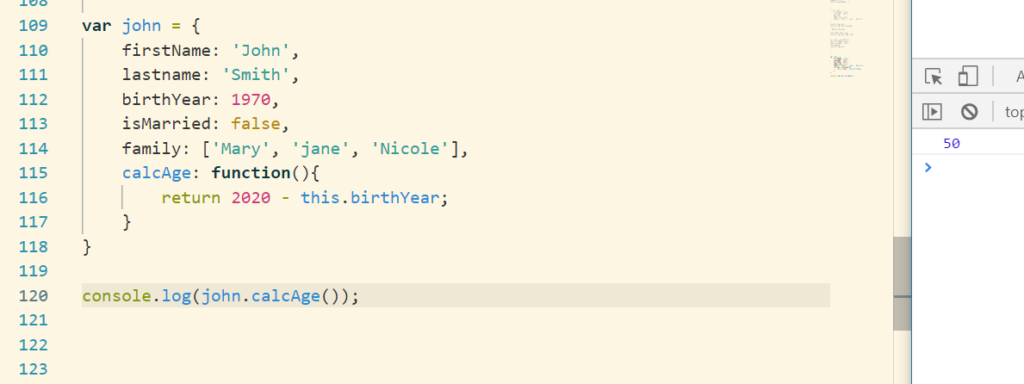
this keyword is used to point towards the current object property.
We can create one more property “age” for john object.
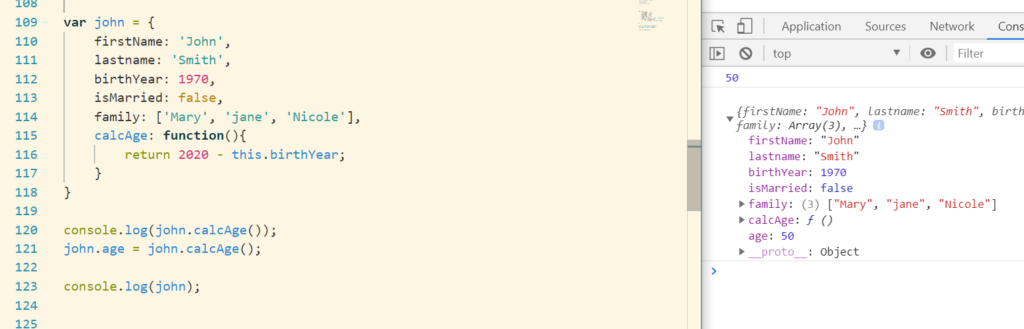
We can further optimize the way to write the age property.
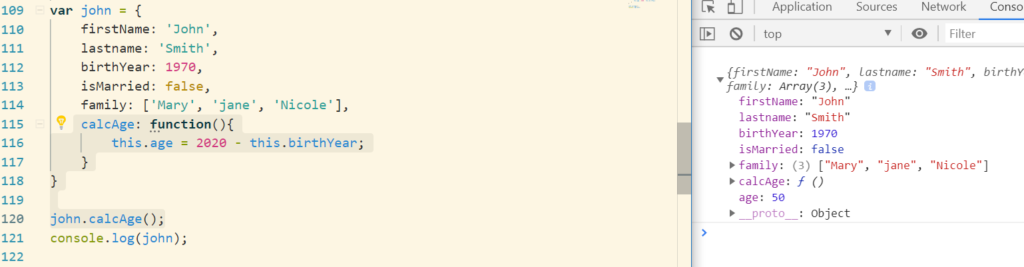